Write code to read a list of song durations and song names from input. For each line of input, set the duration and name of currSong. Then add currSong to playlist. Input first receives a song duration, then the name of that song (which you can assume is only one word long). Input example: 424 Time 383 Money -1 CODE AS IS NOW #include #include #include using namespace std; class Song { public: void SetDurationAndName(int songDuration, string songName) { duration = songDuration; name = songName; } void PrintSong() const { cout << duration << " - " << name << endl; } int GetDuration() const { return duration; } string GetName() const { return name; } private: int duration; string name; }; int main() { vector playlist; Song currSong; int currDuration; string currName; unsigned int i; cin >> currDuration; while (currDuration >= 0) { /* Your code goes here */ cin >> currDuration; } for (i = 0; i < playlist.size(); ++i) { currSong = playlist.at(i); currSong.PrintSong(); } return
NEED C++ HELP WITH WRITING VECTORS WITH CLASSES
Write code to read a list of song durations and song names from input. For each line of input, set the duration and name of currSong.
Then add currSong to playlist.
Input first receives a song duration, then the name of that song (which you can assume is only one word long).
Input example: 424 Time
383 Money
-1
CODE AS IS NOW
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Song {
public:
void SetDurationAndName(int songDuration, string songName) {
duration = songDuration;
name = songName;
}
void PrintSong() const {
cout << duration << " - " << name << endl;
}
int GetDuration() const { return duration; }
string GetName() const { return name; }
private:
int duration;
string name;
};
int main() {
vector<Song> playlist;
Song currSong;
int currDuration;
string currName;
unsigned int i;
cin >> currDuration;
while (currDuration >= 0) {
/* Your code goes here */
cin >> currDuration;
}
for (i = 0; i < playlist.size(); ++i) {
currSong = playlist.at(i);
currSong.PrintSong();
}
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

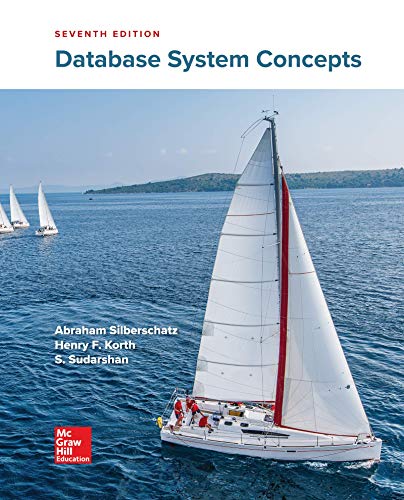
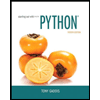
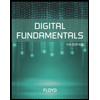
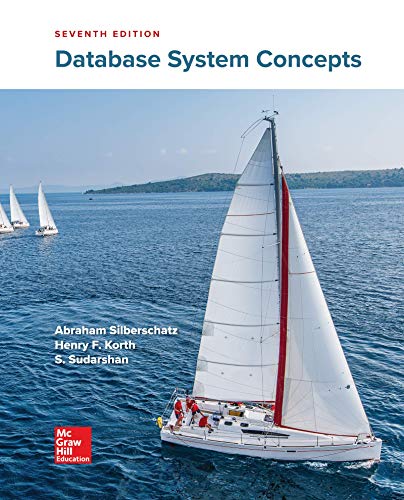
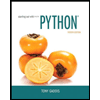
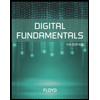
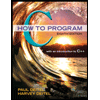
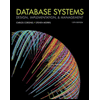
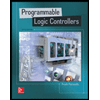