Write C++ code and make it compile and run showing how to use: abstract data type const class constructor You can write a single program or multiple programs to demonstrate the above, I just need to see how each is used and how it runs.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write C++ code and make it compile and run showing how to use:
abstract data type
const
class constructor
You can write a single program or multiple programs to demonstrate the above, I just need to see how each is used and how it runs.

Step-1: Start
Step-2: Define a class Animal as an abstract data type with a pure virtual function makeSound()
Step-3: Define a class Dog that inherits from Animal and has a private string variable name
Step-4: Implement the Dog constructor that takes a string argument to initialize the name variable
Step-5: Implement the makeSound() function in the Dog class to print the name followed by "barks!" to the console
Step-6: Define a constant integer MAX_DOGS with a value of 3
Step-7: Create three Dog objects using the Dog constructor and assign them to variables dog1, dog2, and dog3
Step-8: Create an array of Animal pointers called animals with a size of MAX_DOGS
Step-9: Assign each dog object to a position in the animals array
Step-10: Loop through the animals array using a for loop with the loop variable i ranging from 0 to MAX_DOGS-1
Step-11: Call the makeSound() function on each animal using the arrow operator
Step-12: Stop
Step by step
Solved in 4 steps with 3 images

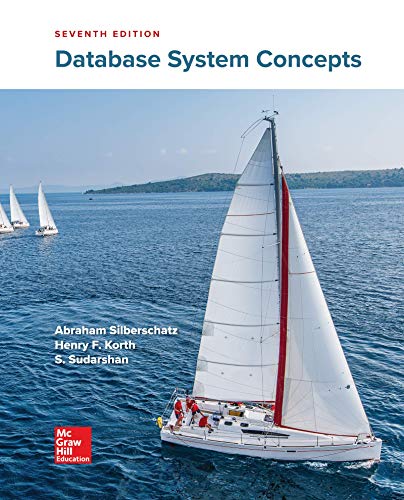
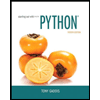
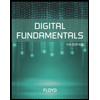
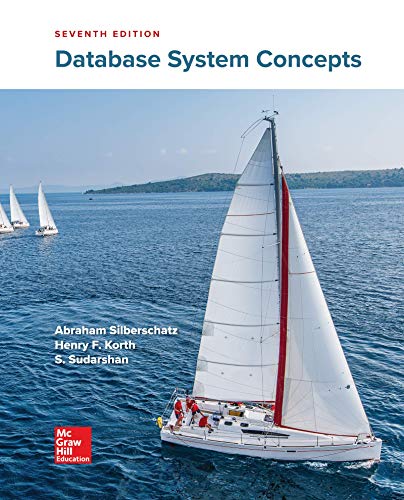
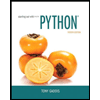
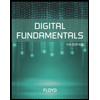
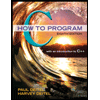
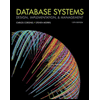
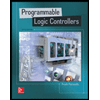