Write a thread class TextThread that prints a text every 1 second 10 times.
-
Write a thread class TextThread that prints a text every 1 second 10 times.
-
Read the following main class. Try to guess what will be its output.
class Test {
public static void main(String s[]) throws Exception {
TextThread x = new TextThread ("I am thread x");
TextThread y= new TextThread ("I am thread y");
System.out.println("I am Main thread");
}
}
-
Write it and execute it. Is the output convenient with your previous answer? What’s wrong? Complete the code so that all thread execute properly.
-
Read the following second main class. Guess its output.
class Test {
public static void main(String s[]) throws Exception {
TextThread x = new TextThread ("Hello");
TextThread y = new TextThread ("Java");
x.start();
x.join();
y.start();
System.out.println("I am Main thread");
}
}
-
Write it and execute it to check your previous answer.
Exercise 3:
-
Read the following code and try to guess what will be its output.
import java.util.*;
class Car {
private int speed;
public void setSpeed(int s){ this.speed=s; }
public int getSpeed(){ return speed; } }
class ChangeSpeedCar extends Thread{
Car car;
int step;
public ChangeSpeedCar(Car obj,String s,int a){
super(s);
this.step=a;
this.car=obj; }
public void run(){
for(int i=0;i<10000;i++)
{ int c=car.getSpeed();
c+=step;
car.setSpeed(c);}
}}
public class test {
public static void main(String[]args){
Car obj =new Car();
obj.setSpeed(0);
ChangeSpeedCar Th1 = new ChangeSpeedCar(obj , "MyThread1",1);
ChangeSpeedCar Th2 = new ChangeSpeedCar(obj , "MyThread2",-1);
Th1.start(); Th2.start();
try{
Thread.sleep(1000);
}catch(Exception e){System.out.print("Error");}
System.out.println("The speed of the car is "+obj.getSpeed());}}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

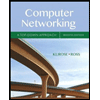
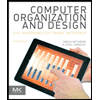
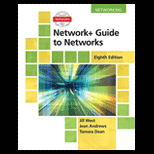
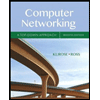
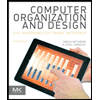
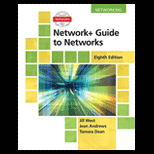
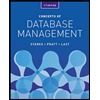
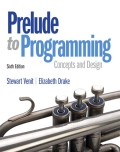
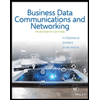