Write a Python program to display cost according to the following table: Distance 0 through 100 More than 100 but not more than 500 More than 500 but less than 1000 1000 or more Cost 5.00 8.00 10.00 12.00
Write a Python program to display cost according to the following table: Distance 0 through 100 More than 100 but not more than 500 More than 500 but less than 1000 1000 or more Cost 5.00 8.00 10.00 12.00
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:### Python Programming: Calculate Shipping Cost Based on Distance
In this lesson, we will create a Python program that calculates the shipping cost based on distance using the following table:
| Distance | Cost |
|-----------------------------------|-------|
| 0 through 100 | 5.00 |
| More than 100 but not more than 500 | 8.00 |
| More than 500 but less than 1000 | 10.00 |
| 1000 or more | 12.00 |
Use the table to set the conditions in your Python program to determine the shipping cost based on the distance provided by the user.
#### Example Code
Here is an example of how you might write this program in Python:
```python
# Function to calculate shipping cost based on distance
def calculate_shipping_cost(distance):
if distance >= 0 and distance <= 100:
return 5.00
elif distance > 100 and distance <= 500:
return 8.00
elif distance > 500 and distance < 1000:
return 10.00
elif distance >= 1000:
return 12.00
else:
return "Invalid distance"
# Example usage
distance = float(input("Enter the distance: "))
cost = calculate_shipping_cost(distance)
print(f"The cost for the given distance is: ${cost:.2f}")
```
#### Explanation of the Table
- **Distances between 0 and 100:** If the shipping distance is between 0 and 100 units, the cost is $5.00.
- **Distances more than 100 but not more than 500:** For distances that are strictly greater than 100 but do not exceed 500 units, the cost is $8.00.
- **Distances more than 500 but less than 1000:** For distances that are strictly greater than 500 but less than 1000 units, the cost is $10.00.
- **Distances of 1000 units or more:** For distances that are 1000 units or more, the cost is $12.00.
This structured approach ensures accurate and consistent calculation of shipping costs based on distance, which is an essential task in many logistical and e-commerce applications.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
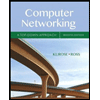
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
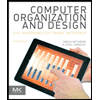
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
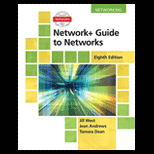
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
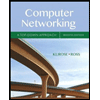
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
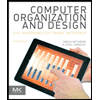
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
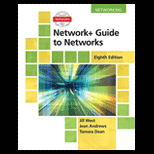
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
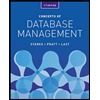
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
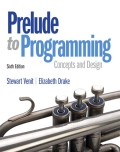
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
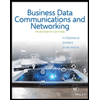
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY