Write a Python program that will generate 1000 random numbers between 1 and 100 inclusive from the random generator. For each value returned from the random generator, keep a count of the number of even numbers generated and the number of odd numbers generated. Use the following functions within this program: - getRandom(): this function will call the random generator and return the generated integer value. - isOdd(): this function will return true or false depending on whether the number generated is odd or even. - update(): this function will update the counters for odd or even. - display(): once the program has completed 1000 random numbers, the counters for the number of odd and even will be displayed. Logistics: - be sure to add a beginning statement when the program starts to execute. - be sure to add an ending statement when the program is complete. - be sure to call the random generator 1000 times for a randomly generated number between 1 and 100 inclusive. - each time you run the project, you will get different output, but you should see a pattern of values. - add comments throughout the code. NOTE: Any project submitted that has all code written in main will receive a grade of 0. An Example: The Even/Odd Challenge Generating random numbers..... After randomly generating 1000 numbers between 1 and 100: The number of odd numbers: 524 The number of even numbers: 476 End of program.
Write a Python
For each value returned from the random generator, keep a count of the number of even numbers generated and the number of odd numbers generated.
Use the following functions within this program:
- getRandom(): this function will call the random generator and return the generated integer value.
- isOdd(): this function will return true or false depending on whether the number generated is odd or even.
- update(): this function will update the counters for odd or even.
- display(): once the program has completed 1000 random numbers, the counters for the number of odd and even will be displayed.
Logistics:
- be sure to add a beginning statement when the program starts to execute.
- be sure to add an ending statement when the program is complete.
- be sure to call the random generator 1000 times for a randomly generated number between 1 and 100 inclusive.
- each time you run the project, you will get different output, but you should see a pattern of values.
- add comments throughout the code.
NOTE: Any project submitted that has all code written in main will receive a grade of 0.
An Example:
The Even/Odd Challenge Generating random numbers..... After randomly generating 1000 numbers between 1 and 100: The number of odd numbers: 524 End of program. |

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

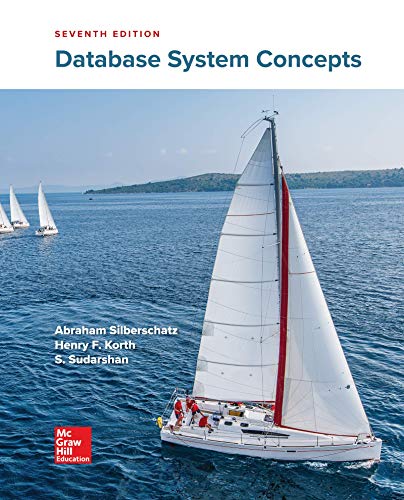
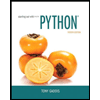
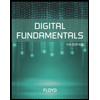
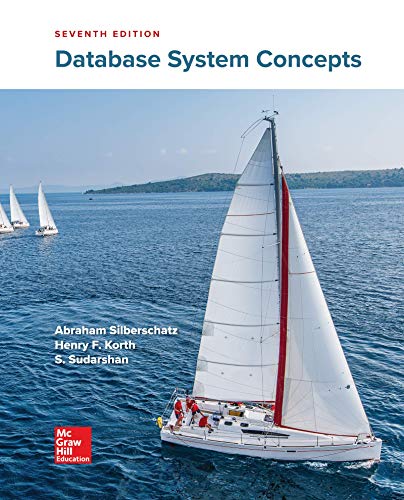
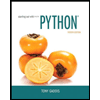
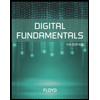
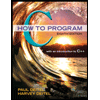
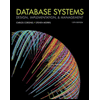
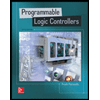