Write a Python program that takes a String as an input and counts the frequency of each character using a dictionary. For solving this problem, you may use each character as a key and its frequency as values. \[You are not allowed to use the count() function\]
### Task 11 (8 points)
Write a Python program that takes a String as an input and
counts the frequency of each character using a dictionary. For solving
this problem, you may use each character as a key and its frequency as
values. \[You are not allowed to use the count() function\]
**Hint:**
1. You can create a new dictionary to store the frequencies.
2. Ignore case for simplicity (i.e. consider P and p to be the same).
3. keys need to be lower case
4. Do not count space. Remove space before counting.
===================================================================
**Sample Input:**
"Python
**Sample Output:**
{'p': 2, 'y': 1, 't': 1, 'h': 1, 'o': 2, 'n': 3, 'r': 2, 'g': 2, 'a': 1,
'm': 2, 'i': 2, 's': 1, 'f': 1, 'u': 1}
===========================
here is my code but I don't know what is wrong
def task11(in_str):
# YOUR CODE HERE
test_str = in_str.lower()
dict_out = {}
for i in test_str:
if i in dict_out:
dict_out[i] += 1
else:
dict_out[i] = 1
return dict_out
![In [61]: #todo
def task11(in_str):
# YOUR CODE HERE
test_strin_str.lower()
dict_out = {}
for i in test_str:
if i in dict_out:
dict_out[i]+= 1
else:
dict_out[i] = 1
return dict_out
In [60]: ning is fun") == {'p': 2, 'y': 1, 't': 1, 'h': 1, 'o': 2, 'n': 3, 'r': 2, 'g': 2, 'a': 1, 'm': 2, 'i': 2, 's': 1, 'f': 1, 'u': 1}
◄
AssertionError
Input In [60], in <cell line: 1>()
----> 1 assert task11("Python programming
'm': 2, 'i': 2, 's': 1, 'f': 1, 'u': 1}
AssertionError:
In [62]: task11("Python programming is fun")
Out [62]: {'p': 2,
'y': 1,
't': 1,
'h': 1.
'o': 2,
'n': 3,
': 3,
'r': 2,
'g': 2,
'a': 1.
'm': 2,
'i': 2.
's': 1,
'f': 1,
'u': 1}
Traceback (most recent call last)
is fun") == {'p': 2, 'y': 1, 't': 1, 'h': 1, 'o': 2, 'n': 3, 'r': 2, 'g': 2, 'a': 1,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa1d592a5-ec96-4b1d-bddf-23e27014debe%2F39b8a671-a12b-4575-abad-e361a6906493%2F5oj0nsq_processed.png&w=3840&q=75)

Step by step
Solved in 4 steps with 2 images

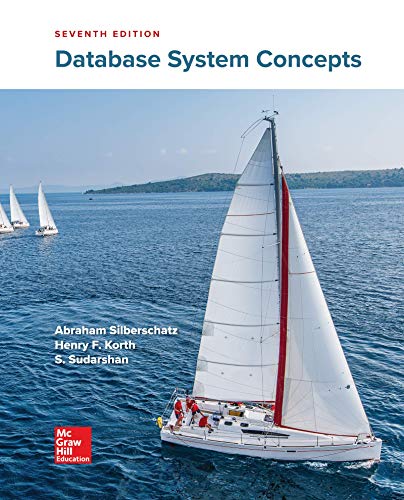
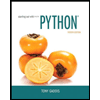
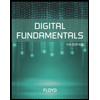
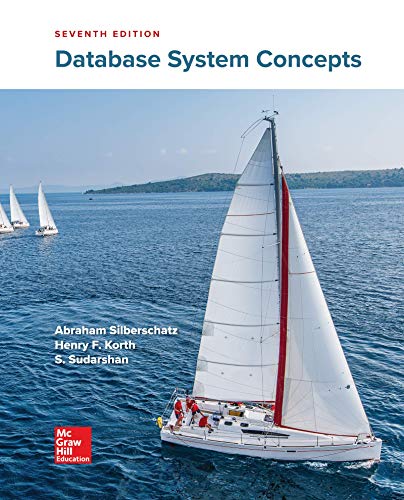
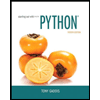
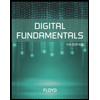
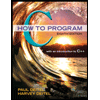
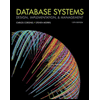
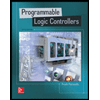