Write a program to illustrate how to use the temporary class, designed in Exercises 13 and 14 of this chapter. Your program must contain statements that would ask the user to enter data of an object and use the setters to initialize the object. The header file for the class temporary from Exercise 13 has been provided. An example of the program is shown below: Enter object name (rectangle, circle, sphere, or cylinder: circle Enter object's dimensions: rectangle (length and width) circle (radius and 0) sphere (radius and 0) rectangle (base raidus and height) 10 0 After setting myObject: circle: radius = 10.00, area = 314.1
Instructions
Write a program to illustrate how to use the temporary class, designed in Exercises 13 and 14 of this chapter. Your program must contain statements that would ask the user to enter data of an object and use the setters to initialize the object.
The header file for the class temporary from Exercise 13 has been provided.
An example of the program is shown below:
Enter object name (rectangle, circle, sphere, or cylinder: circle Enter object's dimensions: rectangle (length and width) circle (radius and 0) sphere (radius and 0) rectangle (base raidus and height) 10 0 After setting myObject: circle: radius = 10.00, area = 314.1
I have already tried a code but I can't get it to do one of the steps right. I have added images of what cant be completed with my code now.
Here is my code :
Main.cpp



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

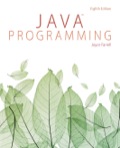
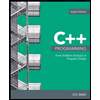
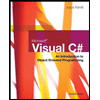
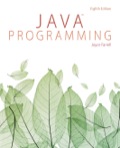
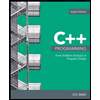
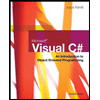
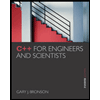
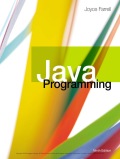