Write a program to calculate the total amount with GST for the events. The Events are Stage show and Exhibition. For the Stage show, GST will be 15% and for exhibition, GST will be 5%.
Write a program to calculate the total amount with GST for the events. The Events are Stage show and Exhibition. For the Stage show, GST will be 15% and for exhibition, GST will be 5%.
[Note: Strictly adhere to the object oriented specifications given as a part of the problem statement.
Follow the naming conventions as mentioned]
Consider class Event with the following protected attributes/variables.
Data Type | Variable |
String | name |
String | type |
Double | costPerDay |
Integer | noOfDays |
Prototype for the parametrized constructor,
public Event(String name, String type, Double costPerDay, Integer noOfDays)
Consider class Exhibition which extends the Event class with the following private attributes/variables.
Data Type | Variable |
static Integer | gst = 5 |
Integer | noOfStalls |
Prototype for the parametrized constructor,
public Exhibition(String name, String type, Double costPerDay, Integer noOfDays, Integer noOfStalls)
Include appropriate getters and setters.
Include the following method.
Method Name | Description |
public Double totalCost() | This method is to calculate the total amount with 5% GST |
Consider class StageEvent which extends the Event class with the following private attributes/variables.
Data Type | Variable |
static Integer | gst = 15 |
Integer | noOfSeats |
Prototype for the parametrized constructor,
public StageEvent(String name, String type, Double costPerDay, Integer noOfDays, Integer noOfSeats)
Include appropriate getters and setters.
Include the following method.
Method Name | Description |
public Double totalCost() | This method is to calculate the total amount with 15% GST |
Override toString() method in all classes to display the event details in the format specified in sample input and output.
Consider Main class with main method.
In the main() method, read the event details from the user and then create the object of the event according to the event type.
The total amount will be calculated according to the GST of the corresponding event.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

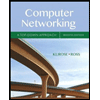
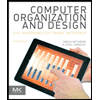
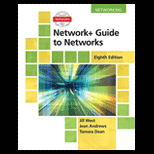
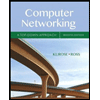
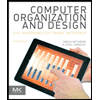
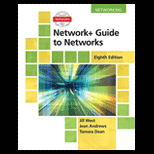
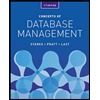
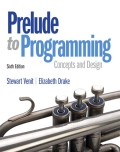
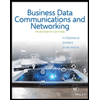