Write a program that simulates the Powerball lottery. In Powerball, a ticket is comprised of 5 numbers be
Lotto Program C++
Write a program that simulates the Powerball lottery. In Powerball, a ticket is comprised of 5 numbers between 1 and 69 that must be unique, and a Powerball number between 1 and 26. The Powerball does not have to be unique.
Hint: You can use an array to represent the 5 unique numbers between 1 and 69, and an integer variable to represent the powerball.
The program asks the player if they'd like to select numbers or do a 'quickpick', where the numbers are randomly generated for them. If they opt to select numbers, prompt them to type the numbers in and validate that they are unique (except the powerball), and in the correct range.
The program then generates a 'drawing' of 5 unique numbers and a Powerball, and checks it against the user's lotto ticket. It assigns winnings accordingly. Because it is so rare to win the lottery, I suggest hard coding or printing values to when testing the part of the program that assigns winnings.
![Enter a unique number (169):24
Enter the powerball number (126): 66
Try again.
Enter the powerball number (126): 24
Player's Ticket: 23 67 5 13 24
Drawing:
15
16 5 12 27
Better luck next time!
Welcome to the Lottery
1. Quick Pick
2. Pick Numbers
3. Exit
Selection: 3
Program ended with exit code: 0
ticket [0] = 1;
ticket [1] = 2;
ticket [2] = 3;
ticket [3] = 4;
ticket [4] = 5;
Arun of the program that shows the input validation and that the program loops until the user chooses to exit.
Unfortunately, I did not win.
drawing[0] = 5;
drawing [1] = 4;
drawing [2] = 2;
drawing [3] = 1;
drawing [4] = 3;
powerball2 = powerball1 = 13;
Then, to test my win logic I hard coded my drawing and ticket values:
Player's Ticket: 1
Drawing:
5
You won the Grand Prize
The progra
24
32
41
1 23
5 4
5
3
32
24
n works when I win the grand prize. I changed a value in the player's ticket:
4
1
9
Player's Ticket:
Drawing:
You won 50,000$
50,000 is awarded. After testing the different combinations, I can be confident it works.
53
13
13
13
13](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F14cda2c8-a0c2-4234-b84b-20d7f7c053e9%2Fb431d4b7-d810-40f3-a90b-19ba77777126%2F7wzxnzh_processed.jpeg&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

The firsrt picture that has on headther "MATCH and PRIZE" does not have to be coded, it is only an example of the poweball game.
The actual sample output it is bellow it.
Here is my code that need some review.
1. The input validation is working perfectly good.
2. I would like to complete the first selection as stated on the questionnaire output.
// Lotto Program
#include<iostream>
using namespace std;
// Functions declaration
void printMenu();
int getSelect(int &);
bool isUnique(int[], int, int);
void printArray(int [], int);
int main()
{
int sel;
printMenu();
getSelect(sel);
return 0;
}
// Functions implementation
// Menu function.
void printMenu()
{
cout << "\tWelcome to the Lottery\t\n";
cout << " ---------------------------------\n";
cout << " 1. Quick Pick\n";
cout << " 2. Pick Numbers\n";
cout << " 3. Exit\n";
}
// getSelection function
int getSelect(int &sel)
{
int count = 0;
int ticket[5];
cout << " Selection: ";
cin >> sel;
// input validation.
while (sel == 3)
{
cout << "Program ending..." << endl;
return 0;
}
while (true)
{
while (sel <= 0 || sel > 3)
{
cout << " Please your selction must be between 1 and 3. Try again.\n";
return 0;
}
//sel = getSelect(sel);
while (sel == 2)
{
while (count < 5)
{
cout << "Enter a unique number (1 - 69): ";
cin >> ticket[count];
while (ticket[count] < 1 || ticket[count] > 69 || !isUnique(ticket, ticket[count], count))
{
cout << "Try again.\n";
cout << "Enter a unique number (1 - 69): ";
cin >> ticket[count];
break;
}
count++;
cout << endl;
}
isUnique(ticket, ticket[count], count);
cout << "Player Ticket: ", printArray(ticket, count);
break;
}
return sel;
}
}
// isUnique Function
// This function determines if there is unique number
// in the array.
bool isUnique(int ticket[], int n, int count)
{
for (int i = 0; i < count; i++)
{
if (ticket[i] == n)
{
return false;
}
}
return true;
}
// printArray Function.
void printArray(int ticket[], int count)
{
for (int i = 0; i < count; i++)
{
cout << ticket[i] << " ";
}
cout << endl;
}
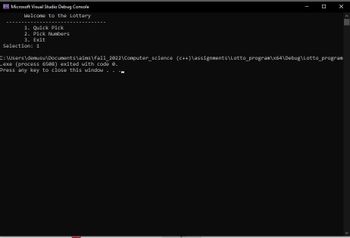
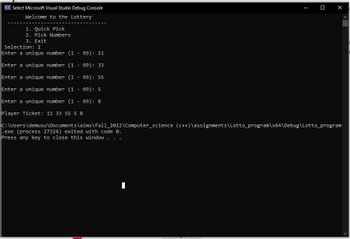
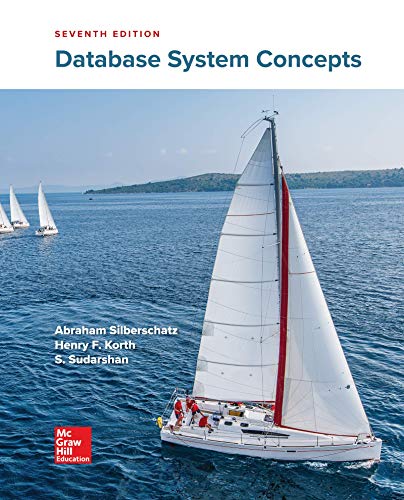
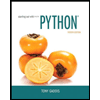
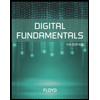
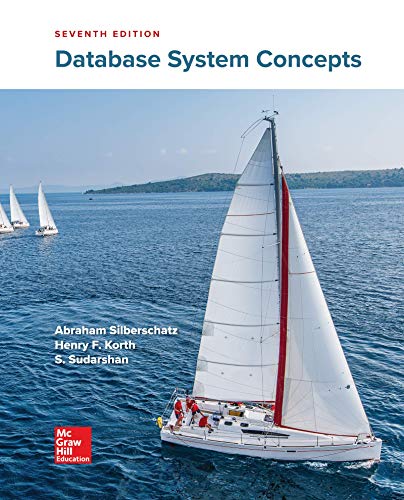
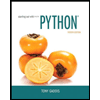
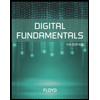
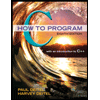
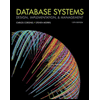
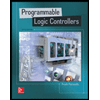