Write a program that reads an array of the following structure and sorts the data in ascending order by name or age using the bubblesort algorithm. struct person ( char name [30]; int age; Your program should read the number of persons from the standard input followed by the array of data corresponding to the persons. You should print the lists of sorted persons in ascending order with respect to their name (alphabetical order) and with respect to their age. Within the sorting according to age, note that if multiple persons have the same age, then they should be sorted alphabetically with respect to their name. Within the sorting according to name, note that if multiple persons have the same name, then they should be sorted with respect to their age. Instead of writing two sorting functions use function pointers such that you can implement one bubblesort function able to sort according to different criteria. You can assume that the input will be valid and that the names will not contain spaces. To pass the testcases your output has to be identical with the provided ones. The pseudocode of the bubblesort algorithm is the following: repeat Swapped - false for i-1 to length (A) -1 inclusive do: if this pair is out of order/ if Ali-1)> A(il then *swap them and remember something changed / owap (Ali-1, Al1) ) swapped-true end if end for until not awapped
Write a program that reads an array of the following structure and sorts the data in ascending order by name or age using the bubblesort algorithm. struct person ( char name [30]; int age; Your program should read the number of persons from the standard input followed by the array of data corresponding to the persons. You should print the lists of sorted persons in ascending order with respect to their name (alphabetical order) and with respect to their age. Within the sorting according to age, note that if multiple persons have the same age, then they should be sorted alphabetically with respect to their name. Within the sorting according to name, note that if multiple persons have the same name, then they should be sorted with respect to their age. Instead of writing two sorting functions use function pointers such that you can implement one bubblesort function able to sort according to different criteria. You can assume that the input will be valid and that the names will not contain spaces. To pass the testcases your output has to be identical with the provided ones. The pseudocode of the bubblesort algorithm is the following: repeat Swapped - false for i-1 to length (A) -1 inclusive do: if this pair is out of order/ if Ali-1)> A(il then *swap them and remember something changed / owap (Ali-1, Al1) ) swapped-true end if end for until not awapped
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I want only code for above problem in C++
![Write a program that reads an array of the following structure and sorts the data in ascending
order by name or age using the bubblesort algorithm.
struct person {
char name [30];
int age;
Your program should read the number of persons from the standard input followed by the array
of data corresponding to the persons. You should print the lists of sorted persons in ascending
order with respect to their name (alphabetical order) and with respect to their age. Within the
sorting according to age, note that if multiple persons have the same age, then they should be
sorted alphabetically with respect to their name. Within the sorting according to name, note that
if multiple persons have the same name, then they should be sorted with respect to their age.
Instead of writing two sorting functions use function pointers such that you can implement one
bubblesort function able to sort according to different criteria.
You can assume that the input will be valid and that the names will not contain spaces. To pass
the testcases your output has to be identical with the provided ones.
The pseudocode of the bubblesort algorithm is the following:
repeat
swapped - false
for i-1to length (A) - 1 inclusive do:
/if this pair is out of order /
if Ali-1] > Alil then
/* swap them and remember something changed /
swap ( A(i-11, A[1) )
Swapped - true
end if
end for
until not swapped
Testcase 7.6: input
Testcase 7.6: output
{anne, 23}; {bob, 20}; (mary, 18};
(mary, 18); (bob, 20}; {anne, 23);
anne
23
mary
18
bob
20](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0db15ac3-a445-4501-87c7-30ee68ca8853%2F76dd4222-ae80-4dd0-b40e-4e05abc09e52%2F98u0kib_processed.png&w=3840&q=75)
Transcribed Image Text:Write a program that reads an array of the following structure and sorts the data in ascending
order by name or age using the bubblesort algorithm.
struct person {
char name [30];
int age;
Your program should read the number of persons from the standard input followed by the array
of data corresponding to the persons. You should print the lists of sorted persons in ascending
order with respect to their name (alphabetical order) and with respect to their age. Within the
sorting according to age, note that if multiple persons have the same age, then they should be
sorted alphabetically with respect to their name. Within the sorting according to name, note that
if multiple persons have the same name, then they should be sorted with respect to their age.
Instead of writing two sorting functions use function pointers such that you can implement one
bubblesort function able to sort according to different criteria.
You can assume that the input will be valid and that the names will not contain spaces. To pass
the testcases your output has to be identical with the provided ones.
The pseudocode of the bubblesort algorithm is the following:
repeat
swapped - false
for i-1to length (A) - 1 inclusive do:
/if this pair is out of order /
if Ali-1] > Alil then
/* swap them and remember something changed /
swap ( A(i-11, A[1) )
Swapped - true
end if
end for
until not swapped
Testcase 7.6: input
Testcase 7.6: output
{anne, 23}; {bob, 20}; (mary, 18};
(mary, 18); (bob, 20}; {anne, 23);
anne
23
mary
18
bob
20
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
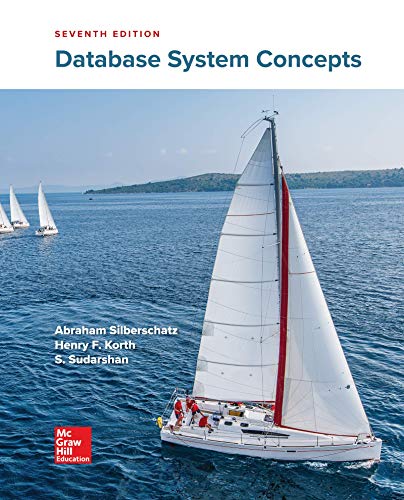
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
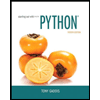
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
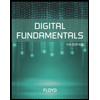
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
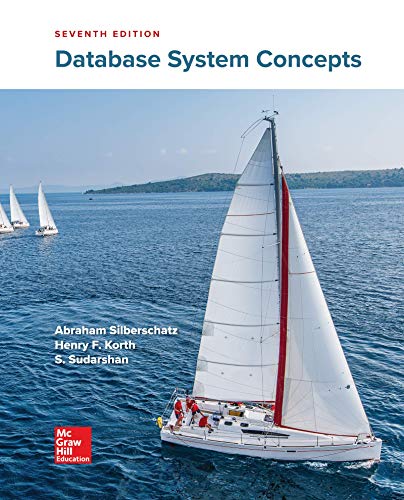
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
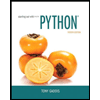
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
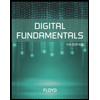
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
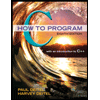
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
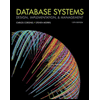
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
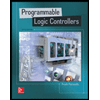
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education