Write a program that passes an unspecified number of integers as command-line arguments to the main method and display the total number of integers that the user entered and their total.
Write a program that passes an unspecified number of integers as command-line arguments to the main method and display the total number of integers that the user entered and their total.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I just started learning java and having trouble with this problem. Please help figure out the coding and please add note if possible next to your codes for my understanding.
![**Task Description: Passing Command-Line Arguments in Java**
**Objective:**
Write a program that passes an unspecified number of integers as command-line arguments to the main method and displays:
- The total number of integers that the user entered
- Their total sum
**Explanation:**
In this task, learners are expected to create a Java program that takes advantage of command-line arguments to accept an unspecified number of integers from the user. The program should then calculate and display two pieces of information:
1. **The Total Number of Integers:** This involves counting how many command-line arguments were provided.
2. **The Sum of the Integers:** This involves summing up all the provided integers.
**Implementation Steps:**
1. **Main Method Setup:** Configure the `main` method to receive command-line inputs.
2. **Input Parsing:** Convert the input arguments from strings to integers.
3. **Counting the Integers:** Use a counter to determine how many integers were entered.
4. **Summing the Integers:** Sum up all the input integers.
5. **Output the Results:** Print the total count of integers and their sum to the console.
**Example Code:**
```java
public class CommandLineSum {
public static void main(String[] args) {
int totalCount = args.length;
int sum = 0;
for (String arg : args) {
sum += Integer.parseInt(arg);
}
System.out.println("Total number of integers entered: " + totalCount);
System.out.println("Sum of the integers: " + sum);
}
}
```
**Explanation of Code:**
- `args` contains the command-line arguments.
- `totalCount` is calculated as the length of `args`.
- The program uses a for-each loop to iterate over `args`, converting each to an integer and adding it to `sum`.
- The results are printed to the console.
**Usage Example:**
To run the program, use the following command in the terminal:
```
java CommandLineSum 3 5 7 2
```
**Expected Output:**
```
Total number of integers entered: 4
Sum of the integers: 17
```
This exercise teaches students how to handle command-line arguments and perform basic operations on them in Java.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2Ff1afebe9-b494-4ea4-bb83-639dfa6537ce%2Flo4wxpb_processed.png&w=3840&q=75)
Transcribed Image Text:**Task Description: Passing Command-Line Arguments in Java**
**Objective:**
Write a program that passes an unspecified number of integers as command-line arguments to the main method and displays:
- The total number of integers that the user entered
- Their total sum
**Explanation:**
In this task, learners are expected to create a Java program that takes advantage of command-line arguments to accept an unspecified number of integers from the user. The program should then calculate and display two pieces of information:
1. **The Total Number of Integers:** This involves counting how many command-line arguments were provided.
2. **The Sum of the Integers:** This involves summing up all the provided integers.
**Implementation Steps:**
1. **Main Method Setup:** Configure the `main` method to receive command-line inputs.
2. **Input Parsing:** Convert the input arguments from strings to integers.
3. **Counting the Integers:** Use a counter to determine how many integers were entered.
4. **Summing the Integers:** Sum up all the input integers.
5. **Output the Results:** Print the total count of integers and their sum to the console.
**Example Code:**
```java
public class CommandLineSum {
public static void main(String[] args) {
int totalCount = args.length;
int sum = 0;
for (String arg : args) {
sum += Integer.parseInt(arg);
}
System.out.println("Total number of integers entered: " + totalCount);
System.out.println("Sum of the integers: " + sum);
}
}
```
**Explanation of Code:**
- `args` contains the command-line arguments.
- `totalCount` is calculated as the length of `args`.
- The program uses a for-each loop to iterate over `args`, converting each to an integer and adding it to `sum`.
- The results are printed to the console.
**Usage Example:**
To run the program, use the following command in the terminal:
```
java CommandLineSum 3 5 7 2
```
**Expected Output:**
```
Total number of integers entered: 4
Sum of the integers: 17
```
This exercise teaches students how to handle command-line arguments and perform basic operations on them in Java.
![Below is the transcription of the provided image for our Educational website, along with an explanation of its components.
---
## Introduction to Java Programming: Lab Assignment
### Lab 9: Simple Addition Program
In this lab, we will learn how to write a basic Java program that prints a message and calculates the sum of numbers. Let's analyze the structure provided in the template:
```java
package homework;
public class Lab9 {
// Main method
public static void main(String[] args) {
System.out.println("You entered " +
/*Please write your code here*/ + " numbers");
// Please write your code here
System.out.println("The sum of these numbers is " +
/*Please write your code here*/);
}
}
```
#### Breakdown of Components:
1. **Package Declaration**:
```java
package homework;
```
- The `package` statement groups related classes. Here, it indicates that the class `Lab9` is part of the `homework` package.
2. **Class Declaration**:
```java
public class Lab9 {
```
- This declares a public class named `Lab9`.
3. **Main Method**:
```java
public static void main(String[] args) {
```
- The `main` method is the entry point of any Java application. It is required to run the program.
4. **Printing the Number of Entries**:
```java
System.out.println("You entered " +
/*Please write your code here*/ + " numbers");
```
- This line prints a message indicating the number of entries. You need to insert the number of entries in place of the highlighted comment `/*Please write your code here*/`.
5. **Calculation and Sum Output**:
```java
// Please write your code here
```
- Here, you should write your own logic to handle the user input and calculate the sum of the numbers.
```java
System.out.println("The sum of these numbers is " +
/*Please write your code here*/);
```
- This line prints the calculated sum. You need to replace the highlighted comment with your code that provides the sum of the numbers.
### Instructions:
1. Fill in the first placeholder with the number of entries (e.g., from user input or predefined values).
2. Write code to calculate the](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F34215fe4-737d-4111-858a-1ca99d565feb%2Ff1afebe9-b494-4ea4-bb83-639dfa6537ce%2Fl1va9nd_processed.png&w=3840&q=75)
Transcribed Image Text:Below is the transcription of the provided image for our Educational website, along with an explanation of its components.
---
## Introduction to Java Programming: Lab Assignment
### Lab 9: Simple Addition Program
In this lab, we will learn how to write a basic Java program that prints a message and calculates the sum of numbers. Let's analyze the structure provided in the template:
```java
package homework;
public class Lab9 {
// Main method
public static void main(String[] args) {
System.out.println("You entered " +
/*Please write your code here*/ + " numbers");
// Please write your code here
System.out.println("The sum of these numbers is " +
/*Please write your code here*/);
}
}
```
#### Breakdown of Components:
1. **Package Declaration**:
```java
package homework;
```
- The `package` statement groups related classes. Here, it indicates that the class `Lab9` is part of the `homework` package.
2. **Class Declaration**:
```java
public class Lab9 {
```
- This declares a public class named `Lab9`.
3. **Main Method**:
```java
public static void main(String[] args) {
```
- The `main` method is the entry point of any Java application. It is required to run the program.
4. **Printing the Number of Entries**:
```java
System.out.println("You entered " +
/*Please write your code here*/ + " numbers");
```
- This line prints a message indicating the number of entries. You need to insert the number of entries in place of the highlighted comment `/*Please write your code here*/`.
5. **Calculation and Sum Output**:
```java
// Please write your code here
```
- Here, you should write your own logic to handle the user input and calculate the sum of the numbers.
```java
System.out.println("The sum of these numbers is " +
/*Please write your code here*/);
```
- This line prints the calculated sum. You need to replace the highlighted comment with your code that provides the sum of the numbers.
### Instructions:
1. Fill in the first placeholder with the number of entries (e.g., from user input or predefined values).
2. Write code to calculate the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
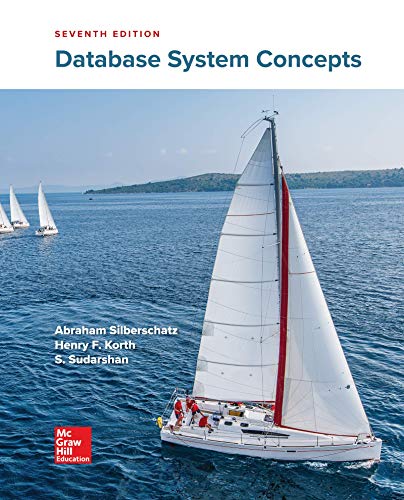
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
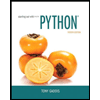
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
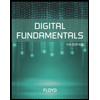
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
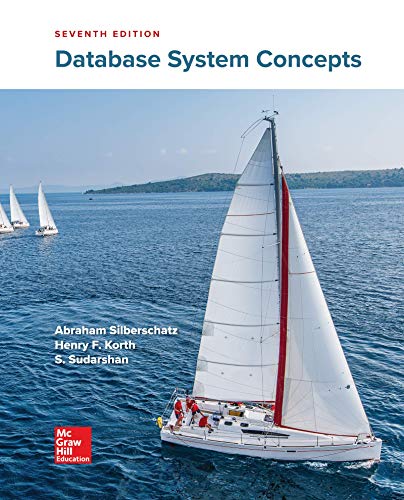
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
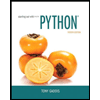
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
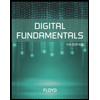
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
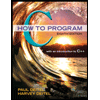
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
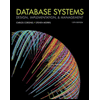
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
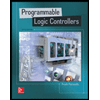
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education