Write a program that asks the user for the number of credits earned so far. If the number of credits is 126 or more, set variable status to 1 and print a message indicating the student can graduate. Otherwise, set status to 0 and print a message indicating the student can't graduate yet. Compute the variable status using the shortcut conditional operator we saw in the lecture (don't use if- statement). Then, use the variable status to decide what to print based on the conditional operator like we did in the lecture (don't use if- statement). Enter credits earned so far: 130 Status = 1 Student is ready to graduate. Enter credits earned so far: 100 Status = 0 Student is not ready to graduate.
Write a program that asks the user for the number of credits earned so far. If the number of credits is 126 or more, set variable status to 1 and print a message indicating the student can graduate. Otherwise, set status to 0 and print a message indicating the student can't graduate yet. Compute the variable status using the shortcut conditional operator we saw in the lecture (don't use if- statement). Then, use the variable status to decide what to print based on the conditional operator like we did in the lecture (don't use if- statement). Enter credits earned so far: 130 Status = 1 Student is ready to graduate. Enter credits earned so far: 100 Status = 0 Student is not ready to graduate.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:### Program Instruction
**Objective**: Write a program that asks the user for the number of credits earned so far. Based on the number of credits, the program will set a status variable and print an appropriate message indicating whether the student can graduate.
**Specification**:
1. **Inputs**: Number of credits earned (integer).
2. **Outputs**: A message indicating whether the student is ready to graduate.
3. **Conditions**:
- If the number of credits is 126 or more, set the `status` variable to 1 and print "Student is ready to graduate."
- Otherwise, set the `status` variable to 0 and print "Student is not ready to graduate."
**Requirement**:
- Compute the `status` variable using the shortcut conditional operator (not with if-statements).
### Example Outputs
1. **Case 1: Student is ready to graduate**
```plaintext
Enter credits earned so far: 130
Status = 1
Student is ready to graduate.
```
2. **Case 2: Student is not ready to graduate**
```plaintext
Enter credits earned so far: 100
Status = 0
Student is not ready to graduate.
```
### Implementation Guide
- **Step 1**: Prompt the user to enter the number of credits earned.
- **Step 2**: Use the conditional operator to set the status based on the number of credits.
- **Step 3**: Print the status and the appropriate message based on the value of the status.
```python
# Sample code outline
credits_earned = int(input("Enter credits earned so far: "))
# Using conditional operator to determine the status
status = 1 if credits_earned >= 126 else 0
# Print the result based on the status
if status == 1:
print("\nStatus = 1")
print("Student is ready to graduate.")
else:
print("\nStatus = 0")
print("Student is not ready to graduate.")
```
By understanding and following these steps and script, students can learn how to incorporate conditional logic effectively without using if-statements, enhancing their programming proficiency.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Recommended textbooks for you
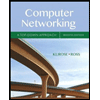
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
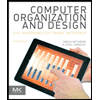
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
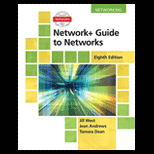
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
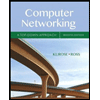
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
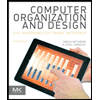
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
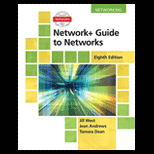
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
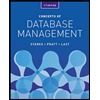
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
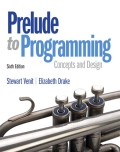
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
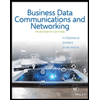
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY