Write a program that allows the user to create and maintain a list of contacts in a file named MyContacts.txt. Each contact will have a name and a phone number. In the file, each line should have a contact's name followed by their phone number you may assume that every contact's name will just be a single word (i.e. no last names) For evamnle if vour fle bas twO contacts in it it might look something like this:
Write a program that allows the user to create and maintain a list of contacts in a file named MyContacts.txt. Each contact will have a name and a phone number. In the file, each line should have a contact's name followed by their phone number you may assume that every contact's name will just be a single word (i.e. no last names) For evamnle if vour fle bas twO contacts in it it might look something like this:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
DO NOT COPY AND PASTE THE ANSWER THAT CHEGG HAS FOR THIS QUESTION. I HAVE ASKED THIS QUESTION ONCE AND THE "EXPERT" COPIED AND PASTED THE ANSWER FROM CHEGG. PLEASE ACTUALLY SOLVE THIS PROBLEM, OR I'LL JUST HAVE TO CANCEL MY BARTLEBY SUBSCRIPTION.
C++ Question
Hello Please answer the following attached C++

Transcribed Image Text:**Contact Management Program Instructions**
This program allows users to create and manage a contact list stored in a file called `MyContacts.txt`. Each contact includes a name and a phone number. Here are the detailed instructions:
1. **File Structure**:
- Each line in the file contains a contact's name followed by their phone number.
- Names are assumed to be a single word (no last names).
Example of file content:
```
Bob 555-1234
Jill 555-4321
```
2. **Handling Non-Existent Files**:
- If the program attempts to read a non-existent file, it will crash.
- Users should add a contact as their first step, which will create a new file if it does not already exist. This occurs within the `addContact` method.
3. **User Options**:
- The program operates in a loop, repeatedly asking users to select an option and executing the corresponding task:
1. **Print List**:
- Function: `printList()`
- This function outputs the contacts in `MyContacts.txt` formatted as:
```
Contact 1: Bob Phone Number: 555-1234
Contact 2: Jill Phone Number: 555-4321
```
2. **Find Number**:
- Function: `findNumber()`
- Users enter a name, and the function searches for the corresponding phone number in `MyContacts.txt`.
- If found, it returns the phone number; otherwise, it returns an empty string.
- The main program prints the result or notifies if the contact does not exist.
3. **Add Contact**:
- Function: `addContact()`
- Allows users to input a name and phone number, which are then appended to `MyContacts.txt`.
- Assumes users will enter single-word names.
4. **Quit**:
- Exits the program.
Each of these functions is designed to facilitate easy management of personal contacts through simple console interactions.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
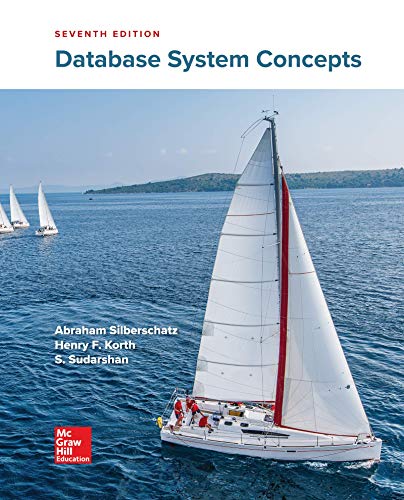
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
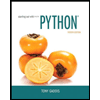
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
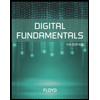
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
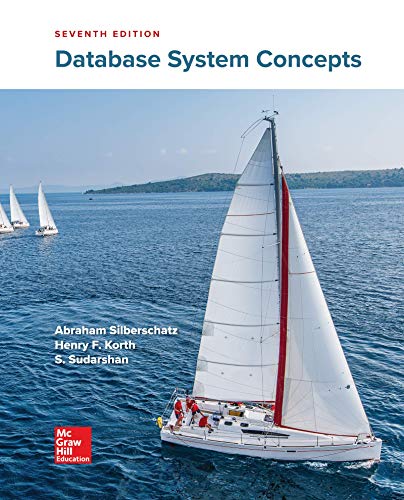
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
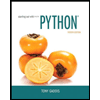
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
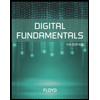
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
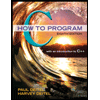
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
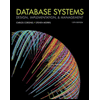
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
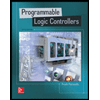
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education