Hello. Please answer the following C programming question correctly by fulfilling all of the directions. Please do not use very advanced syntax to solve the problem and do not use string.h and all functions in this library. Also, please create a complete file header based on the file header style picture. *If correctly fulfill and answer all of the directions correctly, I will give you a thumbs up. Thanks. —------------------------------------------------------------------------------------------------------------------ Question - Instructions: ** For the following question and its steps, do not use string.h and all functions in this library. Step 1. Write a function to reverse a string. Function name: reverse_str Return type: void Parameter list: a pointer to a char Please don’t print the array backward. Don’t use another array to make the string reversed. You should reverse the string in place. Function Description: This function reverses a string by swapping the first character by the last character of the string and continuing with the second character and one character to the last and so on. Make sure don't repeat this procedure up to the size of the string. Otherwise, you will get the same string. To make sure your function works well don't try palindrome strings like RACECAR. Step 2. Write a function to find the size of a string. Function name: size_str Return type: int Parameter list: a pointer to a char. Function Description: Each string has a '\0' or null character(ASCII code of 0) at the end. You can use this property to find the size of a string. Step 3: Write the main function, define a string and call reverse_str to reverse the string and determine if the string is a palindrome or not? Main Function Sample: #include int main() { char str[] = "Your String"; int size; size = size_str(str) //print the size of str reverse_str(str); //print the reverse of str return 0; } void reverse_str(char *str) { //Code it. } int size_str(char *str) { //Code it. }
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
Hello. Please answer the following C
*If correctly fulfill and answer all of the directions correctly, I will give you a thumbs up. Thanks.
—------------------------------------------------------------------------------------------------------------------
Question - Instructions:
** For the following question and its steps, do not use string.h and all functions in this library.
Step 1. Write a function to reverse a string.
- Function name: reverse_str
- Return type: void
- Parameter list: a pointer to a char
- Please don’t print the array backward.
- Don’t use another array to make the string reversed. You should reverse the string in place.
- Function Description: This function reverses a string by swapping the first character by the last character of the string and continuing with the second character and one character to the last and so on. Make sure don't repeat this procedure up to the size of the string. Otherwise, you will get the same string. To make sure your function works well don't try palindrome strings like RACECAR.
Step 2. Write a function to find the size of a string.
- Function name: size_str
- Return type: int
- Parameter list: a pointer to a char.
- Function Description: Each string has a '\0' or null character(ASCII code of 0) at the end. You can use this property to find the size of a string.
Step 3: Write the main function, define a string and call reverse_str to reverse the string and determine if the string is a palindrome or not?
Main Function Sample:
#include <stdio.h>
int main()
{
char str[] = "Your String";
int size;
size = size_str(str)
//print the size of str
reverse_str(str);
//print the reverse of str
return 0;
}
void reverse_str(char *str)
{
//Code it.
}
int size_str(char *str)
{
//Code it.
}


Step by step
Solved in 5 steps with 3 images

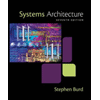
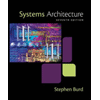