Exercise 3 – Roman Numerals Exercise Tasks: Create a visual studio C++ project exercise3 and submit the cpp file when completed Create a C++ program that converts integers between 1 and 3999 into the corresponding roman numerals. Your solution should print an error message for incorrect input. The following roman numeral conversions should prove useful: 1. I 6. VI 50. L 900. CM 2. II 9. IX 90. XC 1000. M 4. IV 10. X 100. C 5. V 40. XL 500 D. Modify the following tester code to obtain an integer from the user and check that it is between 1 and 3999. Then print out the corresponding roman numerals. While the sample code uses an ifthenelse, you could also use a switch statement. /****************************************************** * Roman Numeral Converter August 13 * converts base 10 positive integer >=0 <4000 to roman numerals * @author */ #include using namespace std; int main(){ int thousands, hundreds, tens, ones; int inputNum, residualNum; cout << "Please enter a positive integer <= 3999: "; cin >> inputNum; if(inputNum < 0 || inputNum >= 4000) { cout << "that input was not correct"; } else { // thousands digit residualNum = inputNum; thousands = residualNum / 1000; residualNum = residualNum % 1000; if(thousands == 3) { cout << "MMM"; } else if (thousands == 2) { cout << "MM"; } else if (thousands == 1) { cout << "M"; } //hundreds digit hundreds = residualNum / 100; residualNum = residualNum % 100; // you need to fill in the missing code here
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
Exercise 3 – Roman Numerals
Exercise Tasks: Create a visual studio C++ project exercise3 and submit the cpp file when completed
Create a C++ program that converts integers between 1 and 3999 into the corresponding roman numerals. Your solution should print an error message for incorrect input. The following roman numeral conversions should prove useful:
1. I 6. VI 50. L 900. CM
2. II 9. IX 90. XC 1000. M
4. IV 10. X 100. C
5. V 40. XL 500 D.
Modify the following tester code to obtain an integer from the user and check that it is between 1 and 3999. Then print out the corresponding roman numerals. While the sample code uses an ifthenelse, you could also use a switch statement.
/******************************************************
* Roman Numeral Converter August 13
* converts base 10 positive integer >=0 <4000 to roman numerals
* @author <your name here>
*/
#include <iostream>
using namespace std;
int main(){
int thousands, hundreds, tens, ones;
int inputNum, residualNum;
cout << "Please enter a positive integer <= 3999: ";
cin >> inputNum;
if(inputNum < 0 || inputNum >= 4000) {
cout << "that input was not correct";
} else {
// thousands digit
residualNum = inputNum;
thousands = residualNum / 1000;
residualNum = residualNum % 1000;
if(thousands == 3) {
cout << "MMM";
} else if (thousands == 2) {
cout << "MM";
} else if (thousands == 1) {
cout << "M";
}
//hundreds digit
hundreds = residualNum / 100;
residualNum = residualNum % 100;
// you need to fill in the missing code here
}
}

Please find the answer below :
Step by step
Solved in 2 steps with 1 images

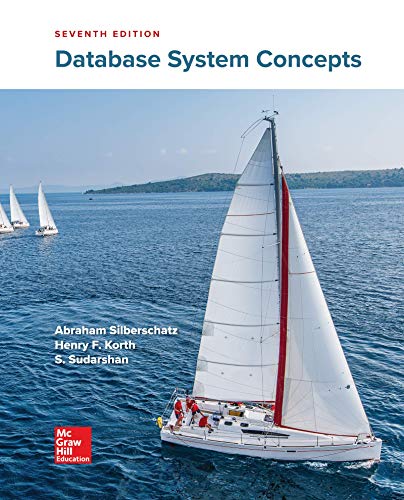
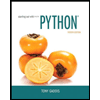
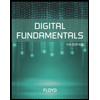
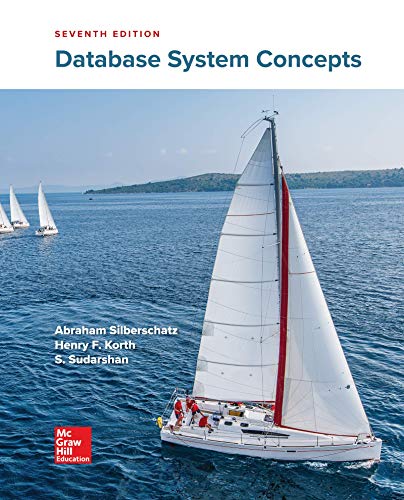
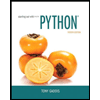
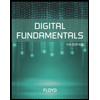
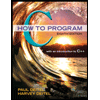
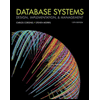
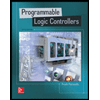