Write A Program In C The Example : #include #include #define MONTHS_SIZE 12 #define DAYS_SIZE 7 int main(void) { const char *month[MONTHS_SIZE] = { "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" }; const char *day[DAYS_SIZE] = { "Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" }; //A for (size_t i = 0; i < MONTHS_SIZE; i++) { const char *mPtr = month[i]; for (; *mPtr != '\0'; ++mPtr) { printf("%c", toupper(*mPtr)); } puts(""); } puts("---------"); for (size_t i = 0; i < DAYS_SIZE; i++) { const char *dPtr = day[i]; for (; *dPtr != '\0'; ++dPtr) { printf("%c", toupper(*dPtr)); } puts(""); } //B return 0; } The question : In the example above, ask the user to input the month in number, then print the equivalent month name from the month array. You need to validate the input from the user and make sure that the user must not enter any number that is beyond months range (1 – 12).
Write A Program In C
The Example :
#include<stdio.h>
#include<ctype.h>
#define MONTHS_SIZE 12
#define DAYS_SIZE 7
int main(void)
{
const char *month[MONTHS_SIZE] = { "January", "February", "March", "April",
"May", "June", "July", "August", "September",
"October", "November", "December" };
const char *day[DAYS_SIZE] = { "Sunday", "Monday", "Tuesday", "Wednesday",
"Thursday", "Friday", "Saturday" };
//A
for (size_t i = 0; i < MONTHS_SIZE; i++)
{
const char *mPtr = month[i];
for (; *mPtr != '\0'; ++mPtr)
{
printf("%c", toupper(*mPtr));
}
puts("");
}
puts("---------");
for (size_t i = 0; i < DAYS_SIZE; i++)
{
const char *dPtr = day[i];
for (; *dPtr != '\0'; ++dPtr)
{
printf("%c", toupper(*dPtr));
}
puts("");
}
//B
return 0;
}
The question :
In the example above, ask the user to input the month in number, then print the equivalent month name from the month array. You need to validate the input from the user and make sure that the user must not enter any number that is beyond months range (1 – 12).

Step by step
Solved in 2 steps with 1 images

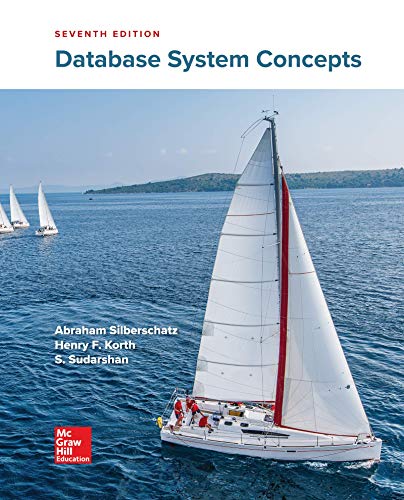
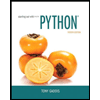
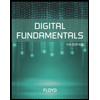
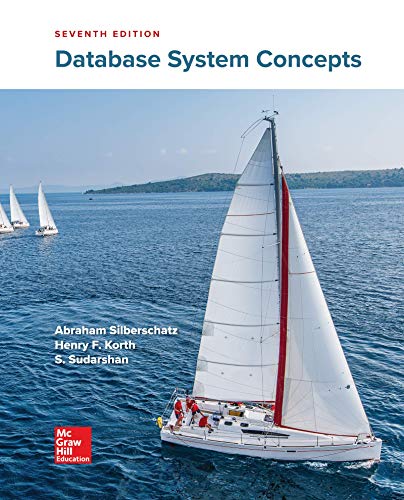
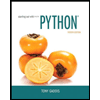
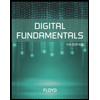
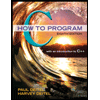
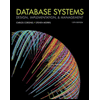
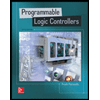