Write a method that takes an integer array as its parameter and sorts the contents of the array in ascending order using the Insertion Sort algorithm (see Topic 6 lecture notes for the algorithm). Call this method (created in exercise 1 of Lab Practice 5) after the original array and other stats have been displayed. Once the array has been sorted by your method, display its contents to the screen in the same manner as the original array was displayed. public class zxc { public static void main(String[] args) { Scanner sc=new Scanner(System.in); //Scanner instance for accepting input from user System.out.print("Enter the number of random numbers to be generated: "); int n=sc.nextInt();//input value of n from user int array[]=new int[n]; //create an array of size n Random generator=new Random(); //Random instance for generating random numbers for(int i=0;ilargest) //if element at index i is more than largest largest=array[i]; //update largest } System.out.println("\n\nSmallest: "+smallest); //print smallest System.out.println("Largest: "+largest); //print largest System.out.println("Average: "+sum/n); //print average } }
Write a method that
takes an integer array as its parameter and sorts the contents of the array in
ascending order using the Insertion Sort
for the algorithm). Call this method (created in exercise 1 of Lab Practice 5)
after the original array and other stats have been displayed. Once the array has
been sorted by your method, display its contents to the screen in the same
manner as the original array was displayed.
public class zxc {
public static void main(String[] args)
{
Scanner sc=new Scanner(System.in); //Scanner instance for accepting input from user
System.out.print("Enter the number of random numbers to be generated: ");
int n=sc.nextInt();//input value of n from user
int array[]=new int[n]; //create an array of size n
Random generator=new Random(); //Random instance for generating random numbers
for(int i=0;i<n;i++) //i from 0 to n-1
{
//generate number from 0 to 998 and then add 1 to it
array[i]=1+generator.nextInt(999);
}
int smallest=array[0]; //initialize smallest
int largest=array[0]; //initialize largest
double sum=0; //initialize sum to 0
for(int i=0;i<n;i++) //i from 0 to n-1
{
if(i%5==0) //if i is multiple of 5
System.out.println(); //move to new line
System.out.print(array[i]+" "); //print the number at index i and a tab space
sum=sum+array[i]; //add the element to sum
if(array[i]<smallest) //if element at index i is less than smallest
smallest=array[i]; //update smallest
if(array[i]>largest) //if element at index i is more than largest
largest=array[i]; //update largest
}
System.out.println("\n\nSmallest: "+smallest); //print smallest
System.out.println("Largest: "+largest); //print largest
System.out.println("Average: "+sum/n); //print average
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

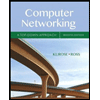
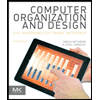
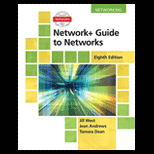
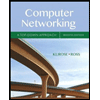
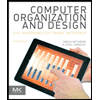
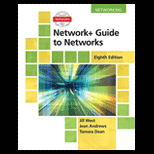
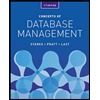
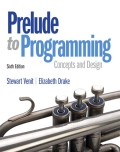
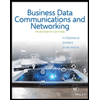