Write a method that takes an integer array as its parameter and sorts the contents of the array in ascending order using the Insertion Sort algorithm. Call this method after the original array (original program below) and other stats have been displayed. Once the array has been sorted by your method, display its contents to the screen in the same manner as the original array was displayed.
Write a method that
takes an integer array as its parameter and sorts the contents of the array in
ascending order using the Insertion Sort
after the original array (original program below) and other stats have been displayed. Once the array has
been sorted by your method, display its contents to the screen in the same
manner as the original array was displayed.
import java.util.Random;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
System.out.println("How many numbers to store?");
//input array size
int size = scnr.nextInt();
//creating array
double[] array = new double[size];
//fill array with random numbers in range 1-999 inclusive
randomArray(array, size, 1, 999);
//find smallest, largest and average
compute(array, size);
}
//method to fill array with randoms numbers within range min to max and print them
private static void randomArray(double[] arr, int n, int min, int max) {
Random q = new Random();
for (int i = 0; i < n; i++) {
arr[i] = q.nextInt((max - min) + 1) + min;
System.out.print(arr[i] + " ");
}
System.out.println();
}
//method to print smallest, largest amd average
private static void compute(double[] val, int n) {
//set min to default max value
double min = Double.MAX_VALUE;
//set min to default min value
double max = Double.MIN_VALUE;
double sum = 0, avg;
//iterate over array
for (int i = 0; i < n; i++) {
//calculate sum
sum = sum + val[i];
//set minimum value
if (val[i] < min)
min = val[i];
//set maximum value
if (val[i] > max)
max = val[i];
}
//calculate average
avg = sum/n;
System.out.println("The smallest number = " + min);
System.out.println("The largest number = " + max);
System.out.format("The average of numbers = %.4f" , avg);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

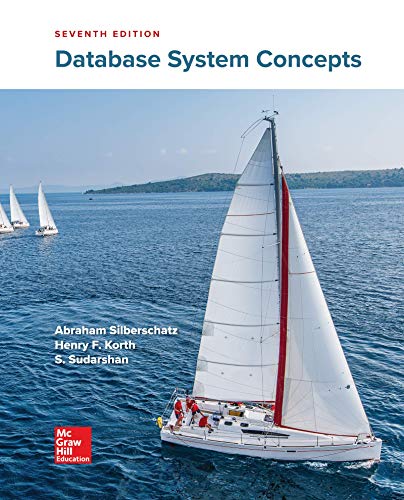
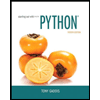
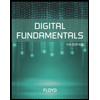
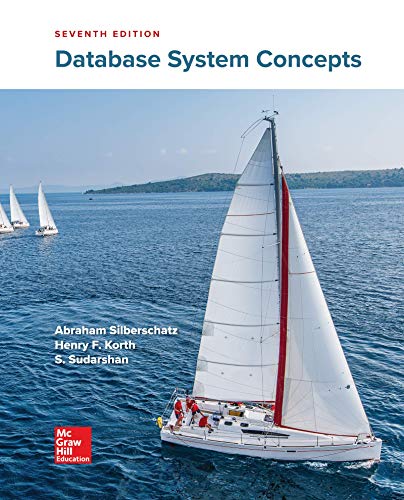
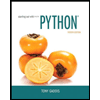
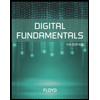
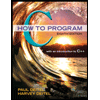
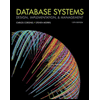
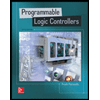