Write a javascript program to print the first occurrence of the I character in the Hello World string without using loops
Write a javascript program to print the first occurrence of the I character in the Hello World string without using loops
Chapter7: Characters, Strings, And The Stringbuilder
Section: Chapter Questions
Problem 12RQ
Related questions
Question

Transcribed Image Text:**Problem Statement:**
Write a JavaScript program to print the first occurrence of the "l" character in the "Hello World" string without using loops.
**Solution Explanation:**
To solve this problem without using loops, you can take advantage of JavaScript's `indexOf` method. This method returns the first index at which a specified character is found in a string, or -1 if the character is not found.
**Sample Solution in JavaScript:**
```javascript
let str = "Hello World";
let index = str.indexOf("l");
if (index !== -1) {
console.log("The first occurrence of 'l' is at index:", index);
} else {
console.log("'l' not found in the string.");
}
```
**Explanation of the Code:**
- **Variable Declaration:** A variable `str` holding the string "Hello World" is declared.
- **Finding the Index:** The `indexOf` method is used on the string `str` to find the first occurrence of the character "l".
- **Conditional Check:** An `if` statement checks if the index is not -1, which means the character is found. If found, it prints the index. Otherwise, it indicates that "l" is not present.
This approach directly finds the first occurrence efficiently without any explicit looping constructs.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
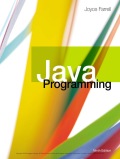
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
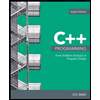
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
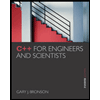
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
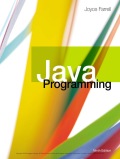
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
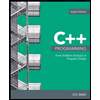
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
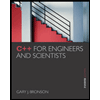
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
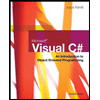
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
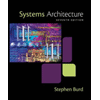
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning