Write a Java program which stores three values by using doubly linked list. - Node class 4. stuID, stuName, stuScore, //data fields 5. constructor 6. update and accessor methods - Singly linked list class which must has following methods: 4. head, tail, size// data fields 5. constructor 6. update and accessor methods a. getSize() //Returns the number of elements in the list. b. isEmpty( ) //Returns true if the list is empty, and false otherwise. c. getFirstStuID( ), getStuName( ), getFirstStuScore( ) d. addFirst (stuID, stuName, stuScore) e. addLast(stuID, stuName, stuScore) f. removeFirst( ) //Removes and returns the first element of the list. g. removeLast( ) //Removes and returns the first element of the list. h. displayList( ) //Displays all elements of the list by traversing the linked list. - Test class – initialize a doubly linked list instance. Test all methods of doubly linked list class.
- Node class
4. stuID, stuName, stuScore, //data fields
5. constructor
6. update and accessor methods
- Singly linked list class which must has following methods:
4. head, tail, size// data fields
5. constructor
6. update and accessor methods
a. getSize() //Returns the number of elements in the list.
b. isEmpty( ) //Returns true if the list is empty, and false otherwise.
c. getFirstStuID( ), getStuName( ), getFirstStuScore( )
d. addFirst (stuID, stuName, stuScore)
e. addLast(stuID, stuName, stuScore)
f. removeFirst( ) //Removes and returns the first element of the list.
g. removeLast( ) //Removes and returns the first element of the list.
h. displayList( ) //Displays all elements of the list by traversing the linked list.
- Test class – initialize a doubly linked list instance. Test all methods of doubly linked list class.

Node.java
1. Create a Node class with the following attributes:
- int stuID
- String stuName
- double stuScore
- Node next (points to the next node)
- Node prev (points to the previous node)
2. Create a constructor for the Node class to initialize stuID, stuName, stuScore, next, and prev.
DoublyLinkedList.java
1. Create a DoublyLinkedList class with the following attributes:
- Node head (points to the first node)
- Node tail (points to the last node)
- int size (stores the number of elements in the list)
2. Create a constructor for the DoublyLinkedList class to initialize head, tail, and size.
3. Implement the following methods:
- getSize(): Returns the number of elements in the list.
- isEmpty(): Returns true if the list is empty; otherwise, returns false.
- getFirstStuID(): Returns the student ID of the first student in the list.
- getStuName(): Returns the student name of the first student in the list.
- getFirstStuScore(): Returns the student score of the first student in the list.
- addFirst(stuID, stuName, stuScore): Adds a student at the beginning of the list.
- addLast(stuID, stuName, stuScore): Adds a student at the end of the list.
- removeFirst(): Removes and returns the first student in the list.
- removeLast(): Removes and returns the last student in the list.
- displayList(): Displays all elements in the list.
4. Implement the addFirst and addLast methods to create a new Node and update the head, tail, and size accordingly.
5. Implement the removeFirst and removeLast methods to remove a student Node from the list and update head, tail, and size accordingly.
6. Implement displayList to iterate through the list and print student information.
TestDoublyLinkedList.java
1. Create a DoublyLinkedList instance called studentList.
2. Use the various methods of the DoublyLinkedList class to add, retrieve, and remove students from the list.
3. Print the results and verify that the doubly linked list operations are working correctly.
Step by step
Solved in 4 steps with 7 images

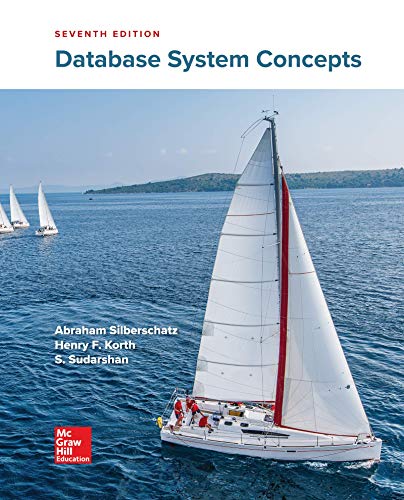
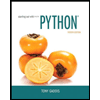
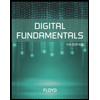
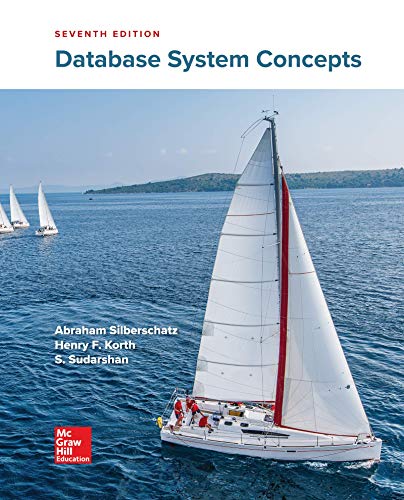
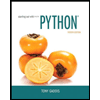
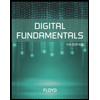
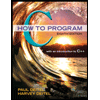
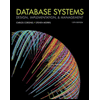
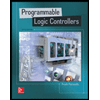