Write a Java program that conforms to the following specifications1. The program consists of a single class called Loops2. Loops has a main method3. Loops has the following static methodsa. calculateSum – the input to this method is an integer. The methods returnsthe sum 1+2+3+…+n. Use a for loop to calculate the sumb. calculateProduct – the input to this method is an integer. The methodreturns 1*2*3*…*n. Use a while loop to calculate the product.c. calculatePower – the input to this method is a double value, say x, and aninteger value – say n. The method calculates and returns xy. Use a for loop to dothe calculation.d. menu – this method requires no input parameters. It provides the user thefollowing choices: 1. Calculate sum 1+2+3+42. Calculate product 1*2*3*…*n3. CalculateXToYPower4. QUITThe method returns the user’s selection. 4. In the main method, you should implement the following:main(){ display menu and get user’s choice (1,2,3,4) LOOP (Use a while or do-while) if the choice is 1 ask the user to enter an integer – say n, then read the input from the user call calculateSum to get sum 1+2+3+…+n print n and the sum if the choice is 2 ask the user to enter an integer – say n, then read the input from the user call calculateProduct to get the product 1*2*3*…*n print n and the product if the choice is 3 ask the user to enter a double, say x, then read the input ask the user to enter an integer – say n, then read the input call calculatePower to get xn print the answer if the choice is 4, terminate the program (You can say System.exit(0); display menu again and get new choiceEND LOOP 5. Here is an outline of the entire programpublic class Loops{ public static int calculateSum(int n) { // put code here } public static double calculateProduct(int n) { // put code here } public static double calculateXToYPower(int n, double x) { //put code here } public static int menu() { //put code here}public static void main(String[] args){ int choice = menu(); while(choice !=4) { if (choice == 1)…… else if(choice == 2) ….etcetera… choice = menu(); }
Write a Java
1. The program consists of a single class called Loops
2. Loops has a main method
3. Loops has the following static methods
a. calculateSum – the input to this method is an integer. The methods returns
the sum 1+2+3+…+n. Use a for loop to calculate the sum
b. calculateProduct – the input to this method is an integer. The method
returns 1*2*3*…*n. Use a while loop to calculate the product.
c. calculatePower – the input to this method is a double value, say x, and an
integer value – say n. The method calculates and returns xy
. Use a for loop to do
the calculation.
d. menu – this method requires no input parameters. It provides the user the
following choices:
1. Calculate sum 1+2+3+4
2. Calculate product 1*2*3*…*n
3. CalculateXToYPower
4. QUIT
The method returns the user’s selection.
4. In the main method, you should implement the following:
main()
{
display menu and get user’s choice (1,2,3,4)
LOOP (Use a while or do-while)
if the choice is 1
ask the user to enter an integer – say n, then read the input from the user
call calculateSum to get sum 1+2+3+…+n
print n and the sum
if the choice is 2
ask the user to enter an integer – say n, then read the input from the user
call calculateProduct to get the product 1*2*3*…*n
print n and the product
if the choice is 3
ask the user to enter a double, say x, then read the input
ask the user to enter an integer – say n, then read the input
call calculatePower to get xn
print the answer
if the choice is 4, terminate the program (You can say System.exit(0);
display menu again and get new choice
END LOOP
5. Here is an outline of the entire program
public class Loops
{
public static int calculateSum(int n)
{
// put code here
}
public static double calculateProduct(int n)
{
// put code here
}
public static double calculateXToYPower(int n, double x)
{
//put code here
}
public static int menu()
{
//put code here
}
public static void main(String[] args)
{
int choice = menu();
while(choice !=4)
{
if (choice == 1)……
else if(choice == 2) ….
etcetera…
choice = menu();
}
Unlock instant AI solutions
Tap the button
to generate a solution
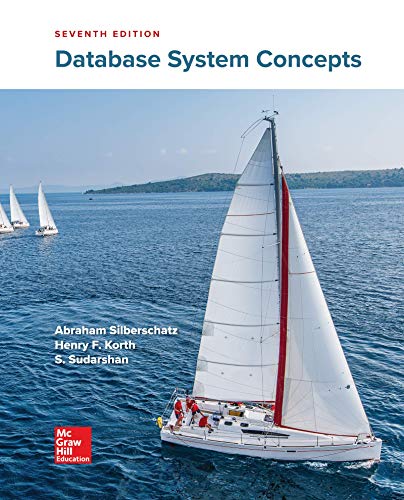
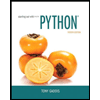
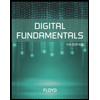
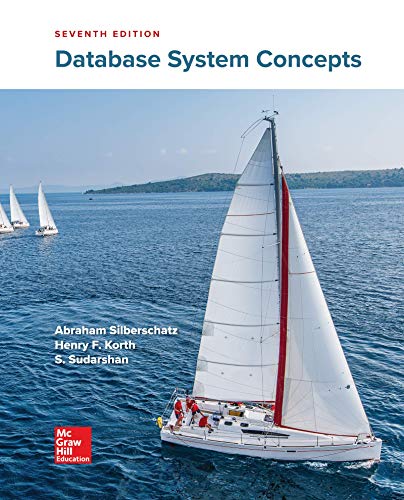
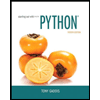
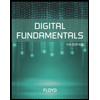
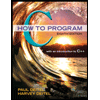
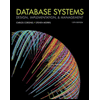
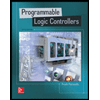