Write a JAVA procedural program that works out the amount a person has to pay for parking in a car park in a tourist town. If they say they are disabled, they are told it is free. Otherwise they enter the number of hours as a whole number (1-8) that they wish to park as well as whether they have an “I live locally” badge or are an old age pensioner both of which leads to a discount. The program tells them the cost to park. The calculation is done in this program as follows. If they are disabled it is free. Otherwise … Take number of hours they wish to park and give a basic charge: • 1 hour: 3.00 pounds • 2-4 hours: 4.00 pounds • 5-6 hours: 4.50 pounds • 7-8 hours: 5.50 pounds Next modify the resulting charge based on whether they are local or not: • If local: subtract 1 pound • If OAP: subtract 2 pounds
Write a JAVA procedural
park in a tourist town. If they say they are disabled, they are told it is free. Otherwise they enter the number
of hours as a whole number (1-8) that they wish to park as well as whether they have an “I live locally”
badge or are an old age pensioner both of which leads to a discount. The program tells them the cost to park.
The calculation is done in this program as follows. If they are disabled it is free. Otherwise … Take number
of hours they wish to park and give a basic charge:
• 1 hour: 3.00 pounds
• 2-4 hours: 4.00 pounds
• 5-6 hours: 4.50 pounds
• 7-8 hours: 5.50 pounds
Next modify the resulting charge based on whether they are local or not:
• If local: subtract 1 pound
• If OAP: subtract 2 pounds
Your program MUST include methods including ones that
- asks for the hours and returns the basic charge.
- asks whether they live locally / are an OAP and returns the amount to subtract.
- EXPLAIN HOW YOUR PROGRAM WORKS
- Write a program that uses if-then-else statements
- Write a program that is split in to methods and includes methods that return a result
- Write a program that includes useful comments, at least one per method saying what it does.
- Write a program that uses indentation in a way that makes its structure clear.
- Provide commenrted code with explanation in every step
Below you will find an example run of the program and the template that you should follow when creating the program.
![import java.util.Scanner; // Needed to make Scanner available
class name // change the name to something appropriate
{
public static void main (String [] a)
{
method_call ); //Change this to a call to the method doing the work
System.exit(0);
}
// Add all methods the program uses here
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa959c3e2-24d7-4bac-89a8-7a621a28f6f4%2F22289558-6fcc-411a-9d8e-ea783711e92d%2Fkobpa1_processed.png&w=3840&q=75)


Step by step
Solved in 4 steps with 6 images

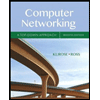
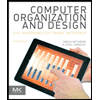
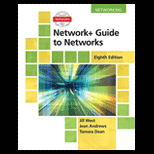
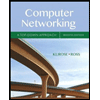
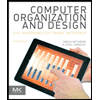
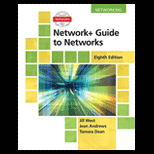
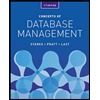
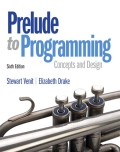
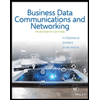