Write a function find_key(input_dict, target_value) that takes as parameters a dictionary and a target value and returns the key in the dictionary that maps to the target value or None if no such key exists. In other words, the function finds the key such that input_dict[key] == target_value. Notes: You may assume that the target_value only appears once in the dictionary and therefore there will be only one key that maps to the target. Hint: You need to iterate over all the keys, as this task is effectively using the dictionary backwards. For example: Test Result test_dictionary = {100:'a', 20:'b', 3:'c', 400:'d'} print(find_key(test_dictionary, 'b')) 20 test_dictionary = {100:'a', 20:'b', 3:'c', 400:'d'} print(find_key(test_dictionary, 'e')) None
Write a function find_key(input_dict, target_value) that takes as parameters a dictionary and a target value and returns the key in the dictionary that maps to the target value or None if no such key exists. In other words, the function finds the key such that input_dict[key] == target_value.
Notes:
- You may assume that the target_value only appears once in the dictionary and therefore there will be only one key that maps to the target.
- Hint: You need to iterate over all the keys, as this task is effectively using the dictionary backwards.
For example:
Test | Result |
---|---|
test_dictionary = {100:'a', 20:'b', 3:'c', 400:'d'} print(find_key(test_dictionary, 'b')) | 20 |
test_dictionary = {100:'a', 20:'b', 3:'c', 400:'d'} print(find_key(test_dictionary, 'e')) | None |

Python
# define a function that takes dictionary and a value as input
def find_key(input_dict, input_value):
# make a list of keys of dictionary
key_list = list(input_dict.keys())
# iterate over the list of keys
for i in key_list:
# if the value at that key is equal to the input value
if input_dict[i] == input_value :
# return that key
return i
# if no key is found, return None
return None
test_dictionary = {100: 'a', 20: 'b', 3:'c', 400: 'd'}
print (find_key(test_dictionary, 'b'))
test_dictionary = {100:'a', 20:'b', 3:'c', 400:'d'}
print(find_key(test_dictionary, 'e'))
Step by step
Solved in 2 steps with 2 images

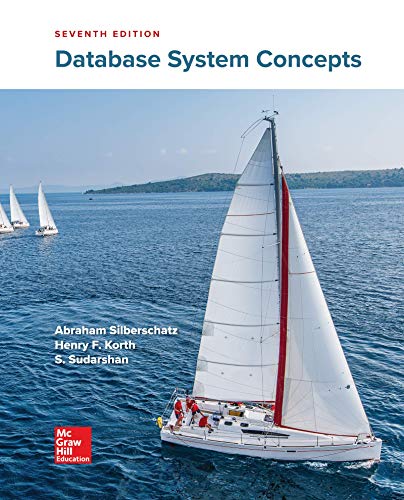
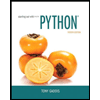
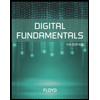
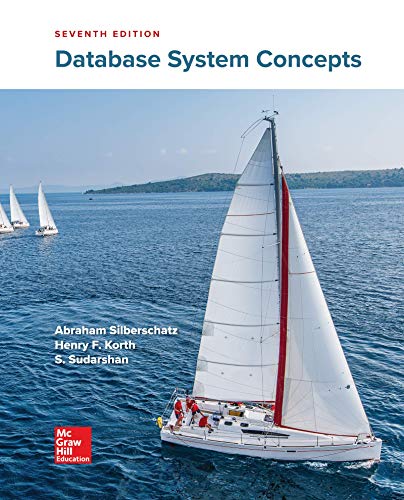
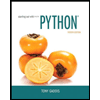
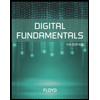
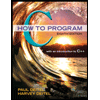
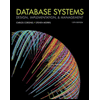
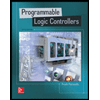