Write a computer program that produces the desired output from the given input. Input: The number of elements in a finite set SOutput: The number of derangements on S
Write a computer
Input: The number of elements in a finite set S
Output: The number of derangements on S

Since you are not mentioning the programming language, here we are using C++ to complete the program.
PROGRAM:
#include <bits/stdc++.h>
using namespace std;
//derange_count() function
int derange_count(int n)
{
// Base case
if (n == 1) return 0;
if (n == 0) return 1;
if (n == 2) return 2;
//counting the Derangements
return ((n - 1) * (derange_count(n - 1) +derange_count(n - 2)));
}
//main() function
int main()
{
int n;
cout<<"\nEnter number of elements is the finite set:";
cin>>n;
cout << "\nNumber of Derangements: "
<< derange_count(n);
return 0;
}
Step by step
Solved in 2 steps with 1 images

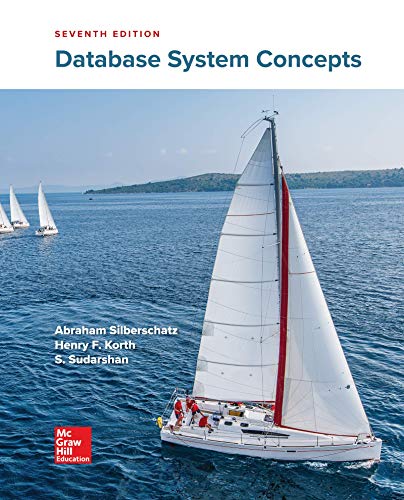
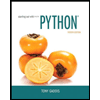
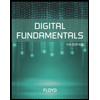
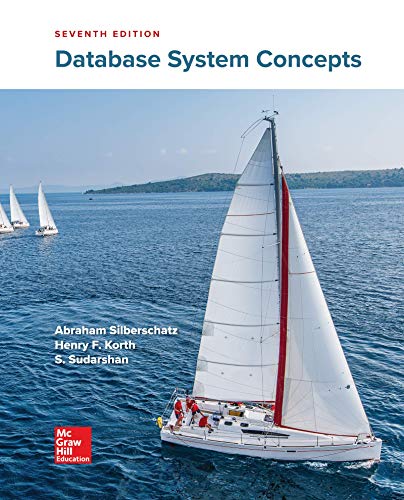
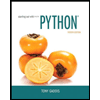
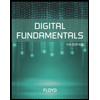
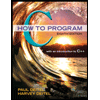
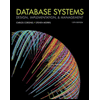
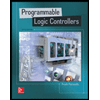