Write a complete program to calculate the value of dollars and cents represented by coins. Your program should accomplish these steps, in order: Part One: Generate the Number of Coins use the Random's nextInt(int) method to generate a random number that represents the number of quarters, dimes, nickels, and pennies there should be at least one of each coin and a max of: 20 quarters, 50 dimes, 100 nickels, and 500 pennies output the number of each coin to the user Part Two: Calculate the Value declare constants to represent the value of each coin note: declare the constants outside of the main method (but inside the class) and declare as: public static final datatype NAME = ...; use arithmetic expressions to calculate how much total dollars and cents are represented by the coins output the total value so that it always displays two decimal points Part Three: Quarters Give-Away ask the user how many people will be given quarters use division and modulus to calculate how many quarters each person will get and how many will be left over output those values to the user Additional Coding Requirements code should compile follow Java naming conventions for variables (lower camel case with no underscores)
Write a complete
Part One: Generate the Number of Coins
- use the Random's nextInt(int) method to generate a random number that represents the number of quarters, dimes, nickels, and pennies
- there should be at least one of each coin and a max of: 20 quarters, 50 dimes, 100 nickels, and 500 pennies
- output the number of each coin to the user
Part Two: Calculate the Value
- declare constants to represent the value of each coin
- note: declare the constants outside of the main method (but inside the class) and declare as:
- public static final datatype NAME = ...;
- use arithmetic expressions to calculate how much total dollars and cents are represented by the coins
- output the total value so that it always displays two decimal points
Part Three: Quarters Give-Away
- ask the user how many people will be given quarters
- use division and modulus to calculate how many quarters each person will get and how many will be left over
- output those values to the user
Additional Coding Requirements
- code should compile
- follow Java naming conventions for variables (lower camel case with no underscores)

Here is a complete Java program that implements the above requirements:
CODE in JAVA:
import java.util.Random;
import java.util.Scanner;
public class CoinValueCalculator {
public static final int QUARTER_VALUE = 25;
public static final int DIME_VALUE = 10;
public static final int NICKEL_VALUE = 5;
public static final int PENNY_VALUE = 1;
public static void main(String[] args) {
Random rand = new Random();
Scanner scan = new Scanner(System.in);
// Part One: Generate the Number of Coins
int numQuarters = rand.nextInt(21) + 1;
int numDimes = rand.nextInt(51) + 1;
int numNickels = rand.nextInt(101) + 1;
int numPennies = rand.nextInt(501) + 1;
System.out.println("Number of quarters: " + numQuarters);
System.out.println("Number of dimes: " + numDimes);
System.out.println("Number of nickels: " + numNickels);
System.out.println("Number of pennies: " + numPennies);
// Part Two: Calculate the Value
int totalCents = (numQuarters * QUARTER_VALUE) + (numDimes * DIME_VALUE) +
(numNickels * NICKEL_VALUE) + (numPennies * PENNY_VALUE);
double totalDollars = (double) totalCents / 100;
System.out.println("\nTotal value: $" + String.format("%.2f", totalDollars));
// Part Three: Quarters Give-Away
System.out.print("\nEnter the number of people who will be given quarters: ");
int numPeople = scan.nextInt();
int quartersPerPerson = numQuarters / numPeople;
int quartersLeft = numQuarters % numPeople;
System.out.println("Each person will receive " + quartersPerPerson + " quarters.");
System.out.println("There will be " + quartersLeft + " quarters left over.");
scan.close();
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

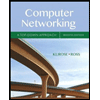
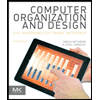
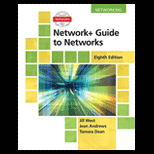
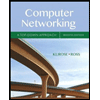
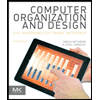
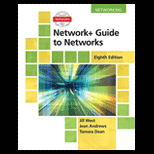
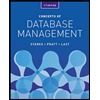
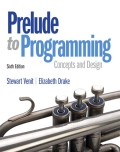
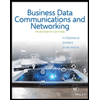