Write a class of objects named Date(in C++) that remembers information about a month and day. Ignore leap years and don't store the year in your object. You must include the following public members: member name description Date(m, d) constructs a new date representing the given month and day daysInMonth() returns the number of days in the month stored by your date object getDay() returns the day getMonth() returns the month nextDay() advances the Date to the next day, wrapping to the next month and/or year if necessary toString() returns a string representation such as "07/04" You should define the entire class including the class heading, the private member variables, and the declarations and definitions of all the public member functions and constructor.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write a class of objects named Date(in C++) that remembers information about a month and day. Ignore leap years and don't store the year in your object. You must include the following public members:
member name | description |
---|---|
Date(m, d) | constructs a new date representing the given month and day |
daysInMonth() | returns the number of days in the month stored by your date object |
getDay() | returns the day |
getMonth() | returns the month |
nextDay() | advances the Date to the next day, wrapping to the next month and/or year if necessary |
toString() | returns a string representation such as "07/04" |
You should define the entire class including the class heading, the private member variables, and the declarations and definitions of all the public member functions and constructor.

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

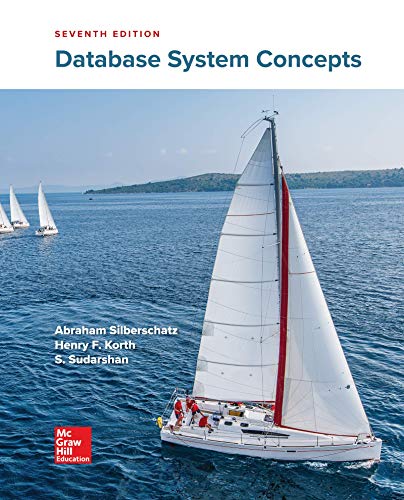
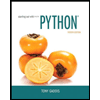
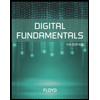
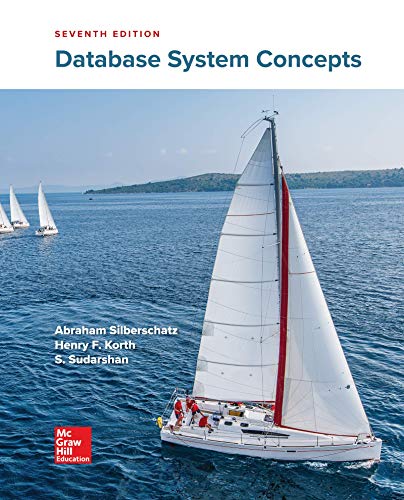
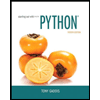
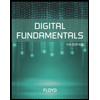
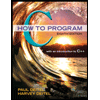
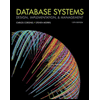
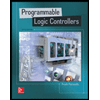