Write a class called BasicPricer. Your class should have the following: A private field called prices that is an ArrayList of Doubles (e.g., ArrayList). A public default constructor that initializes prices to an empty ArrayList of Doubles. A method called getPrices() that returns prices. A method called addPrice(double) that adds the specified price to prices. A method called calclulateTotalPrice() that returns the sum of all of the prices that have been added. Part 2 Edit calclulateTotalPrice() so that it applies a 10% discount to the total price if the total price is >= 1000. Part 3 Write a class called AdvancedPricer that extends BasicPricer. Your class should have the following: A new override version of calclulateTotalPrice() that calculates the total price after dropping the two lowest prices. HINT: you can sort an ArrayList of Doubles in ascending order by calling Collections.sort(getPrices());. public class Main { public static void main(String[] args) { BasicPricer p1 = new BasicPricer(); p1.addPrice(100); p1.addPrice(200); p1.addPrice(300); System.out.println("Price:" + p1.calclulateTotalPrice()); BasicPricer p2 = new AdvancedPricer(); p1.addPrice(100); p1.addPrice(200); p1.addPrice(300); System.out.println("Price:" + p2.calclulateTotalPrice()); BasicPricer p3 = new AdvancedPricer(); p3.addPrice(1000); p3.addPrice(20000); p3.addPrice(600); p3.addPrice(400); System.out.println("Price:" + p3.calclulateTotalPrice()); } }
Write a class called BasicPricer. Your class should have the following:
- A private field called prices that is an ArrayList of Doubles (e.g., ArrayList<Double>).
- A public default constructor that initializes prices to an empty ArrayList of Doubles.
- A method called getPrices() that returns prices.
- A method called addPrice(double) that adds the specified price to prices.
- A method called calclulateTotalPrice() that returns the sum of all of the prices that have been added.
Part 2
- Edit calclulateTotalPrice() so that it applies a 10% discount to the total price if the total price is >= 1000.
Part 3
Write a class called AdvancedPricer that extends BasicPricer. Your class should have the following:
- A new override version of calclulateTotalPrice() that calculates the total price after dropping the two lowest prices. HINT: you can sort an ArrayList of Doubles in ascending order by calling Collections.sort(getPrices());.
public class Main
{
public static void main(String[] args)
{
BasicPricer p1 = new BasicPricer();
p1.addPrice(100);
p1.addPrice(200);
p1.addPrice(300);
System.out.println("Price:" + p1.calclulateTotalPrice());
BasicPricer p2 = new AdvancedPricer();
p1.addPrice(100);
p1.addPrice(200);
p1.addPrice(300);
System.out.println("Price:" + p2.calclulateTotalPrice());
BasicPricer p3 = new AdvancedPricer();
p3.addPrice(1000);
p3.addPrice(20000);
p3.addPrice(600);
p3.addPrice(400);
System.out.println("Price:" + p3.calclulateTotalPrice());
}
}

Below is the required code in java and sample output:
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

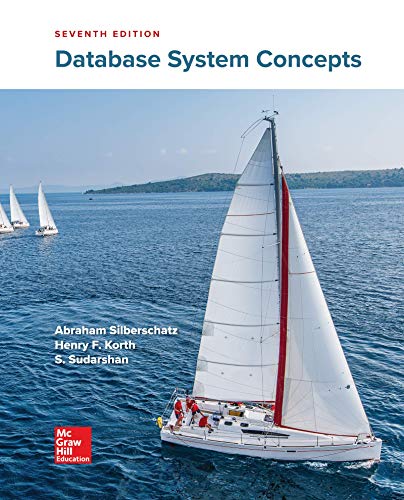
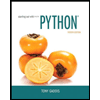
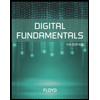
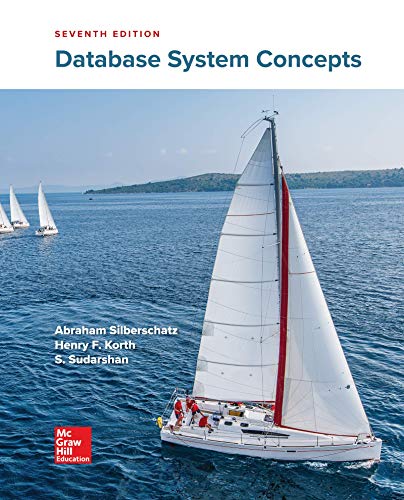
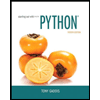
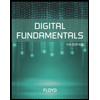
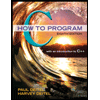
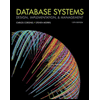
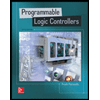