Write a class called BankAccount with the following specifications: Fields (You should encapsulate all data inside your objects) iD (int) balance (double) name (string) Methods Default constructor sets the ID to 0 sets the balance to 0 sets the name to "unknown" Full constructor Passes all three values as parameters and set the values accordingly String toString() returns a string in the format as follows: Name [ID] = $balance Example: Betty [1233203] $105.5 String getName () returns the Name of this BankAccount double getBalance () returns the Balance of this BankAccount
Write a class called BankAccount with the following specifications: Fields (You should encapsulate all data inside your objects) iD (int) balance (double) name (string) Methods Default constructor sets the ID to 0 sets the balance to 0 sets the name to "unknown" Full constructor Passes all three values as parameters and set the values accordingly String toString() returns a string in the format as follows: Name [ID] = $balance Example: Betty [1233203] $105.5 String getName () returns the Name of this BankAccount double getBalance () returns the Balance of this BankAccount
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please read all directions carefully!
Java Program Please
![### BankAccount Class Specification
#### Overview:
Create a class called `BankAccount` with the following specifications:
#### Fields:
- **iD (int)**: A unique identifier for each bank account.
- **balance (double)**: Represents the account balance.
- **name (string)**: The name of the account holder.
*Note: All data should be encapsulated within the objects.*
#### Methods:
##### Default Constructor:
- Initializes the `iD` to `0`.
- Sets the `balance` to `0`.
- Sets the `name` to `"unknown"`.
##### Full Constructor:
- Accepts all three values (iD, balance, name) as parameters and sets them accordingly.
##### String toString():
- Returns a string formatted as:
```
Name [ID] = $balance
```
- Example:
```
Betty [1233203] = $105.5
```
##### String getName():
- Returns the `name` of the `BankAccount`.
##### double getBalance():
- Returns the `balance` of the `BankAccount`.
This class and its methods provide the basic structure and functionality for managing bank accounts programmatically.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5c647828-f044-4f1b-b879-19c790819471%2Fb2128d60-2ba2-4dee-82d6-deaa6c730277%2F5tckgzc_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### BankAccount Class Specification
#### Overview:
Create a class called `BankAccount` with the following specifications:
#### Fields:
- **iD (int)**: A unique identifier for each bank account.
- **balance (double)**: Represents the account balance.
- **name (string)**: The name of the account holder.
*Note: All data should be encapsulated within the objects.*
#### Methods:
##### Default Constructor:
- Initializes the `iD` to `0`.
- Sets the `balance` to `0`.
- Sets the `name` to `"unknown"`.
##### Full Constructor:
- Accepts all three values (iD, balance, name) as parameters and sets them accordingly.
##### String toString():
- Returns a string formatted as:
```
Name [ID] = $balance
```
- Example:
```
Betty [1233203] = $105.5
```
##### String getName():
- Returns the `name` of the `BankAccount`.
##### double getBalance():
- Returns the `balance` of the `BankAccount`.
This class and its methods provide the basic structure and functionality for managing bank accounts programmatically.
![## BankAccount Class Methods and Sample Output
### Methods
#### `int getID()`
- **Purpose**: Returns the ID of this `BankAccount`.
#### `void setName(String newName)`
- **Purpose**: Changes the name on the account with `newName`.
#### `void changeBalance(double x)`
- **Purpose**:
- Adds the amount `x` to the `balance`.
- If `x` is negative, it is still added, but the balance should decrease.
- If the resulting `balance` is negative, set it to zero before finishing.
### Sample Output
```
----jGRASP exec: java BankAccountMainProgram
*** Does the toStringMethod work?
First account : unknown[0] = $0.0
Second account : Betty[1233203] = $105.51
Third account : Veronica[6542345] = $33.11
*** Does the get() methods work?
Betty has $105.51 in account number 1233203.
*** Does the set() methods work?
Second account Before : Betty[1233203] = $105.51
Second account After : Archie[1233203] = $105.51
*** Does the changeBalance() methods work?
Third account Before : Veronica[6542345] = $33.11
Third account After : Veronica[6542345] = $43.21
Third account After : Veronica[6542345] = $0.0
```
This output tests the functionality of various methods in the `BankAccount` class, demonstrating changes to account names and balances.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5c647828-f044-4f1b-b879-19c790819471%2Fb2128d60-2ba2-4dee-82d6-deaa6c730277%2Fbsrgrym_processed.jpeg&w=3840&q=75)
Transcribed Image Text:## BankAccount Class Methods and Sample Output
### Methods
#### `int getID()`
- **Purpose**: Returns the ID of this `BankAccount`.
#### `void setName(String newName)`
- **Purpose**: Changes the name on the account with `newName`.
#### `void changeBalance(double x)`
- **Purpose**:
- Adds the amount `x` to the `balance`.
- If `x` is negative, it is still added, but the balance should decrease.
- If the resulting `balance` is negative, set it to zero before finishing.
### Sample Output
```
----jGRASP exec: java BankAccountMainProgram
*** Does the toStringMethod work?
First account : unknown[0] = $0.0
Second account : Betty[1233203] = $105.51
Third account : Veronica[6542345] = $33.11
*** Does the get() methods work?
Betty has $105.51 in account number 1233203.
*** Does the set() methods work?
Second account Before : Betty[1233203] = $105.51
Second account After : Archie[1233203] = $105.51
*** Does the changeBalance() methods work?
Third account Before : Veronica[6542345] = $33.11
Third account After : Veronica[6542345] = $43.21
Third account After : Veronica[6542345] = $0.0
```
This output tests the functionality of various methods in the `BankAccount` class, demonstrating changes to account names and balances.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
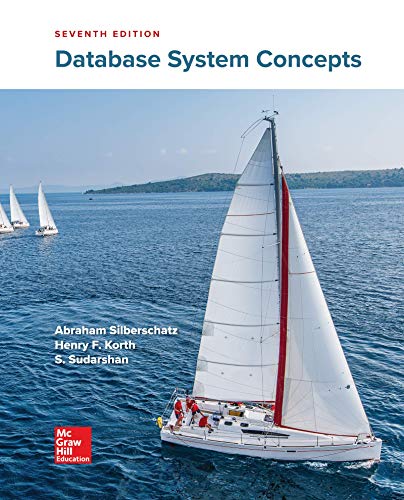
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
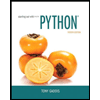
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
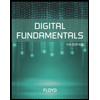
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
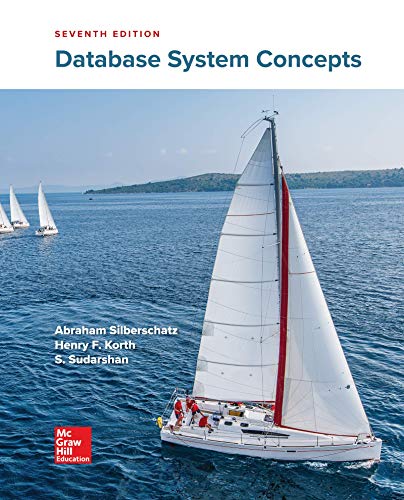
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
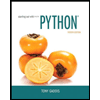
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
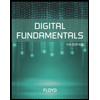
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
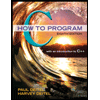
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
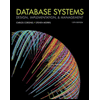
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
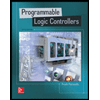
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education