Write a C++ program that fills a 5 x 5 matrix of integers. The matrix must be a static matrix; its size must be fixed at compile-time. Display the contents of the matrix. Query the user for an integer. Pass the matrix and the integer to a bool function that searches for the first occurrence, and only the first occurrence, of the integer in the matrix. If the integer is found, the function returns true (and passes back the row and column coordinates of the first occurrence of the integer); if not, the function returns false. Main then displays whether the integer was found in the matrix and if so, the coordinates of the first occurrence. The use of a break to exit a loop or a function should be avoided. My code does not find the location of the first occurrence and does not use a bool function. Can you please help me to find the error. This is my code: #include #include using namespace std; int main() { int guess, array[5][5]; bool found = false; for(int i=0; i<5; i++) { // Populate matrix for(int j=0; j<5; j++) { array[i][j] = rand() % 20 + 1; // seems to generate the same set of numbers every time } } for(int i=0; i<5; i++) { // display matrix for(int j=0; j<5; j++) { cout << array[i][j] << " "; } cout << endl; } // Query for a guess cout << endl << "What number would you like to search for?"; cin >> guess; cout << endl; for(int i=0; i<5; i++) { // search matrix for(int j=0; j<5; j++) { if(guess == array[i][j] && found == false) { found = true; } } } if(found == false) { // display result cout << "Your guess has not been found" << endl; } else{ cout << "Your guess has been found" << endl; } return 0;
Write a C++ program that fills a 5 x 5 matrix of integers. The matrix must be a static matrix; its size must be fixed at compile-time. Display the contents of the matrix. Query the user for an integer. Pass the matrix and the integer to a bool function that searches for the first occurrence, and only the first occurrence, of the integer in the
matrix. If the integer is found, the function returns true (and passes back the row and column coordinates of the first occurrence of the integer); if not, the function returns false. Main then displays whether the integer was found in the matrix and if so, the coordinates of the first occurrence. The use of a break to exit a loop or a function should be avoided.
My code does not find the location of the first occurrence and does not use a bool function. Can you please help me to find the error.
This is my code:
#include <iostream>
#include <random>
using namespace std;
int main()
{
int guess, array[5][5];
bool found = false;
for(int i=0; i<5; i++)
{ // Populate matrix
for(int j=0; j<5; j++)
{
array[i][j] = rand() % 20 + 1; // seems to generate the same set of numbers every time
}
}
for(int i=0; i<5; i++)
{ // display matrix
for(int j=0; j<5; j++)
{
cout << array[i][j] << " ";
}
cout << endl;
}
// Query for a guess
cout << endl << "What number would you like to search for?";
cin >> guess;
cout << endl;
for(int i=0; i<5; i++)
{ // search matrix
for(int j=0; j<5; j++)
{
if(guess == array[i][j] && found == false)
{
found = true;
}
}
}
if(found == false)
{ // display result
cout << "Your guess has not been found" << endl;
}
else{
cout << "Your guess has been found" << endl;
}
return 0;
}

Step by step
Solved in 4 steps with 4 images

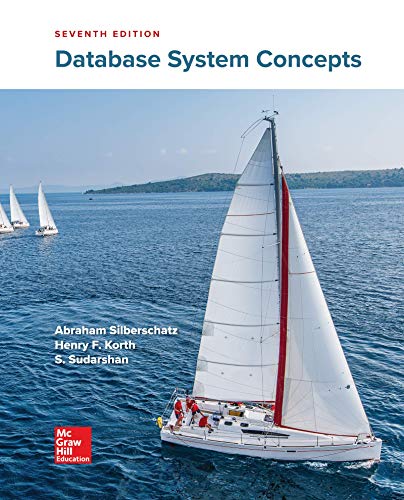
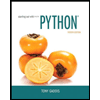
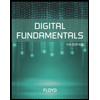
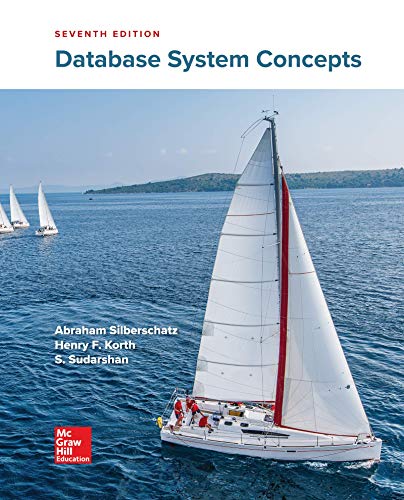
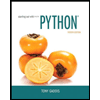
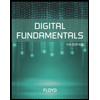
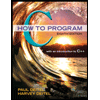
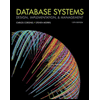
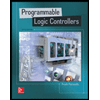