Write a C++ program that asks the user how many schools are participating in this year's competition. You can assume that this number is at least 3. Then, ask for each school's name and call another function; this separate function should ask the user for 3 scores and return the average of those scores. Once you have gotten each school's name and average score, print out everyone's score and then who won gold, silver, and bronze. Your C++ program MUST include at least 1 array, at least 1 loop, and at least 1 function other than int main. If you are having difficulty determining gold, silver, and bronze, you can determine just who gets gold and still receive a high grade. You do not have to do any input validation. You must display all decimals with 3 digits after the decimal point. You can also assume that there will be no tied scores. SAMPLE OUTPUT Welcome to the 2023 CUNY Gymnastics Competition! How many schools are participating this year? 5 What is school 1's name? Brooklyn What score did their 1st athlete get? 8.7 What score did their 2nd athlete get? 6.25 What score did their 3rd athlete get? 7.97 What is school 2's name? Medgar What score did their 1st athlete get? 7.432 What score did their 2nd athlete get? 9.5 What score did their 3rd athlete get? 8.637 What is school 3's name? Queens What score did their 1st athlete get? 8.33 What score did their 2nd athlete get? 8.167 What score did their 3rd athlete get? 7.462 What is school 4's name? BMCC What score did their 1st athlete get? 7 What score did their 2nd athlete get? 6.551 What score did their 3rd athlete get? 6.23 What is school 5's name? Kingsborough What score did their 1st athlete get? 9 What score did their 2nd athlete get? 7.65 What score did their 3rd athlete get? 7.955 Here are the final scores: Brooklyn - 7.640 Medgar - 8.523 Queens - 7.986 BMCC - 6.594 Kingsborough - 8.202 Gold goes to Medgar! Silver goes to Kingsborough! Bronze goes to Queens
Write a C++ program that asks the user how many schools are participating in this year's competition. You can assume that this number is at least 3. Then, ask for each school's name and call another function; this separate function should ask the user for 3 scores and return the average of those scores. Once you have gotten each school's name and average score, print out everyone's score and then who won gold, silver, and bronze.
Your C++ program MUST include at least 1 array, at least 1 loop, and at least 1 function other than int main. If you are having difficulty determining gold, silver, and bronze, you can determine just who gets gold and still receive a high grade.
You do not have to do any input validation. You must display all decimals with 3 digits after the decimal point. You can also assume that there will be no tied scores.
SAMPLE OUTPUT
Welcome to the 2023 CUNY Gymnastics Competition!
How many schools are participating this year? 5
What is school 1's name? Brooklyn
What score did their 1st athlete get? 8.7
What score did their 2nd athlete get? 6.25
What score did their 3rd athlete get? 7.97
What is school 2's name? Medgar
What score did their 1st athlete get? 7.432
What score did their 2nd athlete get? 9.5
What score did their 3rd athlete get? 8.637
What is school 3's name? Queens
What score did their 1st athlete get? 8.33
What score did their 2nd athlete get? 8.167
What score did their 3rd athlete get? 7.462
What is school 4's name? BMCC
What score did their 1st athlete get? 7
What score did their 2nd athlete get? 6.551
What score did their 3rd athlete get? 6.23
What is school 5's name? Kingsborough
What score did their 1st athlete get? 9
What score did their 2nd athlete get? 7.65
What score did their 3rd athlete get? 7.955
Here are the final scores:
Brooklyn - 7.640
Medgar - 8.523
Queens - 7.986
BMCC - 6.594
Kingsborough - 8.202
Gold goes to Medgar!
Silver goes to Kingsborough!
Bronze goes to Queens!

Step by step
Solved in 3 steps with 1 images

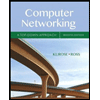
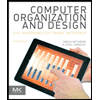
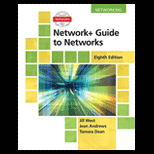
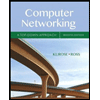
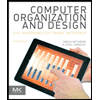
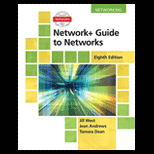
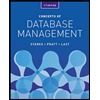
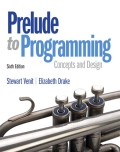
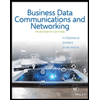