Write a C program for survey data analysis, that can compute the mean, median and mode of the data.
Write a C program for survey data analysis, that can compute the mean, median and mode of the data.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a C
![47
48
49
50
51
52
53
54
55
56
57
58
59
60 /* sort array and determine median element's value */
61 void median(int answer [])
62
{
63
64
/* total response values */
for (j = 0; j < SIZE; j++ ) {
total + answer[j];
} /* end for */
65
66
67
68
69
70
71
72
73
74
75
76
77
printf("The mean is the average value of the data\n"
"items. The mean is equal to the total of\n"
"all the data items divided by the number\n"
"of data items (%d). The mean value for\n"
"this run is: %d/%d = %.4f\n\n",
SIZE, total, SIZE, ( double ) total / SIZE );
} /* end function mean */
拜安安安安安安伯公里。 " Median", 并安安安安安安安林;
"The unsorted array of responses is" );
printArray( answer ); /* output unsorted array */
bubbleSort( answer ); /* sort array */
Fig. 6.16 Survey data analysis program. (Part 3 of 8.)
printf("\n%s\n%s\n%s\n%s",
©1992-2010 by Pearson Education, Inc. All Rights Reserved.
printf("\n\nThe sorted array is" );
printArray( answer ); /* output sorted array */
/* display median element */
printf("\n\nThe median is element %d of\n"
"the sorted %d element array.\n"
"For this run the median is %d\n\n",
SIZE / 2, SIZE, answer[ SIZE / 2 ] );
78
79
80
81
/* determine most frequent response */
82 void mode(int freq[], const int answer [])
83
{
84
85
86
87
88
89
90
91
92
} /* end function median */
int rating; /* counter for accessing elements 1-9 of array freq */
int j; /* counter for summarizing elements 0-98 of array answer */
int h; /* counter for diplaying histograms of elements in array freq */
int largest = 0; /* represents largest frequency */
int modeValue = 0; /* represents most frequent response */
printf("\n%s\n%s\n%s\n",
林公安窃窃窃窃窃窃群,
Mode", "********");
Fig. 6.16 Survey data analysis program. (Part 4 of 8.)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F09c257a9-c4f0-4111-92e4-af310914193a%2F7cd414db-9df3-419e-a6b8-4a72887bb240%2Fe61f03_processed.jpeg&w=3840&q=75)
Transcribed Image Text:47
48
49
50
51
52
53
54
55
56
57
58
59
60 /* sort array and determine median element's value */
61 void median(int answer [])
62
{
63
64
/* total response values */
for (j = 0; j < SIZE; j++ ) {
total + answer[j];
} /* end for */
65
66
67
68
69
70
71
72
73
74
75
76
77
printf("The mean is the average value of the data\n"
"items. The mean is equal to the total of\n"
"all the data items divided by the number\n"
"of data items (%d). The mean value for\n"
"this run is: %d/%d = %.4f\n\n",
SIZE, total, SIZE, ( double ) total / SIZE );
} /* end function mean */
拜安安安安安安伯公里。 " Median", 并安安安安安安安林;
"The unsorted array of responses is" );
printArray( answer ); /* output unsorted array */
bubbleSort( answer ); /* sort array */
Fig. 6.16 Survey data analysis program. (Part 3 of 8.)
printf("\n%s\n%s\n%s\n%s",
©1992-2010 by Pearson Education, Inc. All Rights Reserved.
printf("\n\nThe sorted array is" );
printArray( answer ); /* output sorted array */
/* display median element */
printf("\n\nThe median is element %d of\n"
"the sorted %d element array.\n"
"For this run the median is %d\n\n",
SIZE / 2, SIZE, answer[ SIZE / 2 ] );
78
79
80
81
/* determine most frequent response */
82 void mode(int freq[], const int answer [])
83
{
84
85
86
87
88
89
90
91
92
} /* end function median */
int rating; /* counter for accessing elements 1-9 of array freq */
int j; /* counter for summarizing elements 0-98 of array answer */
int h; /* counter for diplaying histograms of elements in array freq */
int largest = 0; /* represents largest frequency */
int modeValue = 0; /* represents most frequent response */
printf("\n%s\n%s\n%s\n",
林公安窃窃窃窃窃窃群,
Mode", "********");
Fig. 6.16 Survey data analysis program. (Part 4 of 8.)
![93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
/* initialize frequencies to 0 */
for ( rating = 1; rating <= 9; rating++ ) {
freq[ rating ] = 0;
} /* end for */
112
113
114
115
116
/* summarize frequencies */
for (j = 0; j < SIZE; j++ ) {
++freq[ answer[j] ];
} /* end for */
/* output headers for result columns */
printf("%s%lls%19s\n\n%54s\n%54s\n\n",
"Response", "Frequency", "Histogram",
"1 1 2 2", "5 05
/* output results */
for ( rating = 1; rating <= 9; rating++ ) {
printf("%8d%11d
0
5" );
", rating, freq[ rating ] );
/* keep track of mode value and largest frequency value */
if ( freq[ rating ] > largest ) {
largest
modeValue
freq[ rating ];
rating;
} /* end if */
Fig. 6.16 | Survey data analysis program. (Part 5 of 8.)
117
118
119
120
121
122
123
124
125
126
127
128
129
130} /* end function mode */
131
Fig. 6.16 Survey data analysis program. (Part 6 of 8.)
©1992-2010 by Pearson Education, Inc. All Rights Reserved.
/* output histogram bar representing frequency value */
for (h= 1; h <= freq[ rating ]; h++ ) {
printf("*" );
} /* end inner for */
printf("\n"); /* being new line of output */
} /* end outer for */
/* display the mode value */
printf("The mode is the most frequent value.\n"
"For this run the mode is %d which occurred"
" %d times.\n", modeValue, largest );](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F09c257a9-c4f0-4111-92e4-af310914193a%2F7cd414db-9df3-419e-a6b8-4a72887bb240%2Fmjag7rq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
/* initialize frequencies to 0 */
for ( rating = 1; rating <= 9; rating++ ) {
freq[ rating ] = 0;
} /* end for */
112
113
114
115
116
/* summarize frequencies */
for (j = 0; j < SIZE; j++ ) {
++freq[ answer[j] ];
} /* end for */
/* output headers for result columns */
printf("%s%lls%19s\n\n%54s\n%54s\n\n",
"Response", "Frequency", "Histogram",
"1 1 2 2", "5 05
/* output results */
for ( rating = 1; rating <= 9; rating++ ) {
printf("%8d%11d
0
5" );
", rating, freq[ rating ] );
/* keep track of mode value and largest frequency value */
if ( freq[ rating ] > largest ) {
largest
modeValue
freq[ rating ];
rating;
} /* end if */
Fig. 6.16 | Survey data analysis program. (Part 5 of 8.)
117
118
119
120
121
122
123
124
125
126
127
128
129
130} /* end function mode */
131
Fig. 6.16 Survey data analysis program. (Part 6 of 8.)
©1992-2010 by Pearson Education, Inc. All Rights Reserved.
/* output histogram bar representing frequency value */
for (h= 1; h <= freq[ rating ]; h++ ) {
printf("*" );
} /* end inner for */
printf("\n"); /* being new line of output */
} /* end outer for */
/* display the mode value */
printf("The mode is the most frequent value.\n"
"For this run the mode is %d which occurred"
" %d times.\n", modeValue, largest );
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
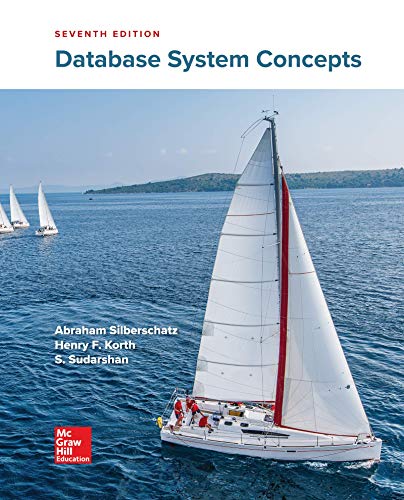
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
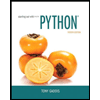
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
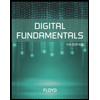
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
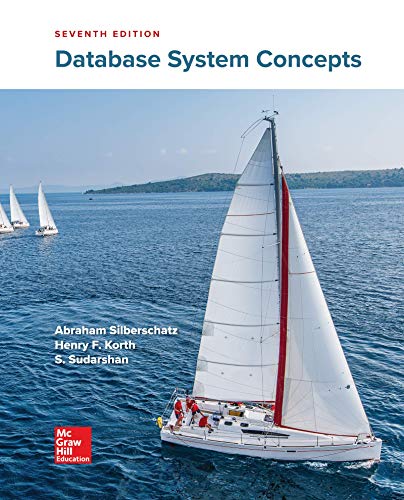
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
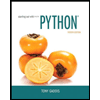
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
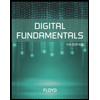
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
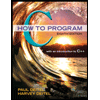
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
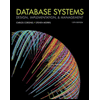
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
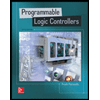
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education