Working with Queues 1. Create a method on the Queue class that calculates the number of times a given value occurs in the queue. int Queue::count( const T & data ) const; Example: count(...) function Result Queue count (5) count (10) front->(1) (1) (5) (3) (5) (5) (2)<-back front->(1) (1) (5) (3) (5) (5) (5) (2)<-back 2. Create a method on the Queue class that determines whether the queue is in descending order. bool Queue::isDecreasing( ) const; Example: isDecreasing() function Result Queue front->(10) (9) (8) (7) (1)<-back front->(1) (1) (5) (3) (5) (5) (5) (2)<-back count (5) true count (10) false
This is the source code for Queues, but it has so many errors. Please update this without errors and warnings.
template<class T>
int queue <T>::isDecreasing() const
{
if (size() < 2) return true;
cout << "test" << endl;
for (size_t i = 0; i < size() - 1; i++)
{
cout << (i + 1 + _front) % _capacity << " : " << elements[(i + 1 + _front) % _capacity] << " vvvvv "
<< (i + _front) % _capacity << " : " << elements[(i + _front) % _capacity] << endl;
if (elements[(i + 1 + _front) % _capacity] > elements[(i + _front) % _capacity])
return false;
}
return true;
}
template<class T>
size_t queue<T>::count(const T& data) const;
{
size_t counter = 0;
for (size_t i = 0; i < size(); i++)
{
cout << i << ":" << elements[(_front + i) % _capacity] << endl;
if (elements[(_front + i) % _capacity] == data) counter++;
}
return counter;
}
And the output must be like this


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

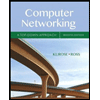
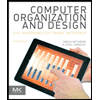
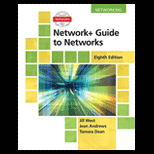
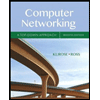
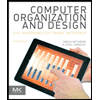
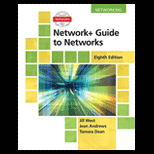
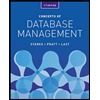
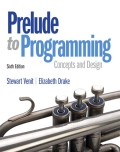
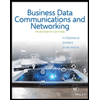