Working on program #2 this is what I have so far but it keeps saying my turtle is undefined. Can anybody help?
Working on program #2 this is what I have so far but it keeps saying my turtle is undefined. Can anybody help?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Working on program #2 this is what I have so far but it keeps saying my turtle is undefined. Can anybody help?

Transcribed Image Text:Below is the transcription of the Python turtle graphics code, suitable for an educational context:
```python
import turtle
# Prompts
number_of_sides = int(input("How many sides?"))
pen_size = int(input("What size should the pen be?"))
side_size = int(input("What size should each side be?"))
side_color = input("What color should the side be?")
# Polygon
polygon = turtle.Turtle()
pen_size = (pen_size)
turtle.color(side_color)
for x in range(0, number_of_sides):
polygon.forward(side_size)
polygon.right(360/number_of_sides)
turtle.done()
```
### Explanation:
This script utilizes the Python 'turtle' module to draw a polygon. The user is prompted to enter:
1. **number_of_sides**: Determines how many sides the polygon has.
2. **pen_size**: Specifies the thickness of the lines drawn.
3. **side_size**: Defines the length of each side of the polygon.
4. **side_color**: Sets the color for the sides of the polygon.
The turtle then draws the polygon by iterating the specified number of sides, moving forward by the side size, and turning by an angle calculated as `360/number_of_sides` to create the polygon. Once complete, the `turtle.done()` function is called to finish the drawing.

Transcribed Image Text:**Turtle Graphics Programming Exercises**
**Objective:** Write two Python programs that utilize Turtle graphics:
**Program #1:**
- Task: Draw a square, triangle, and octagon on the screen.
- Requirements:
- The shapes should not touch or overlap.
- Each shape should be a different color.
- The octagon should be drawn using a while loop.
**Program #2:**
- Task: Ask the user to input the following details:
- Number of sides
- Length of each side
- Color of the sides
- The pen size
- Once the inputs are received, the program should draw a regular polygon based on the specified properties.
Feel free to explore different shapes and customize the designs using Turtle graphics by experimenting with colors and length dimensions!
Expert Solution

Step 1
Below is the modified python program: -
Approach: -
- Import turtle.
- Prompts the user to enter the pen size, the number of sides, side size, and the side color.
- Create the object of the turtle.
- Read the values.
- Use the loop to create the polygon.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
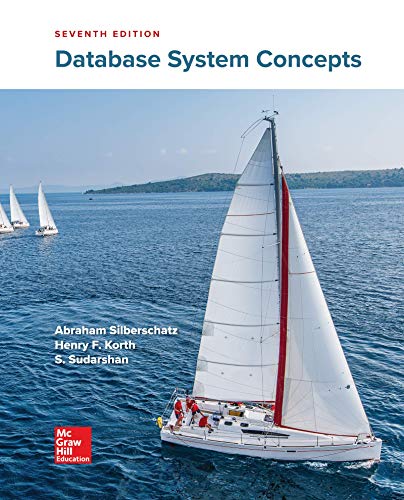
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
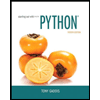
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
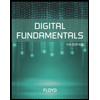
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
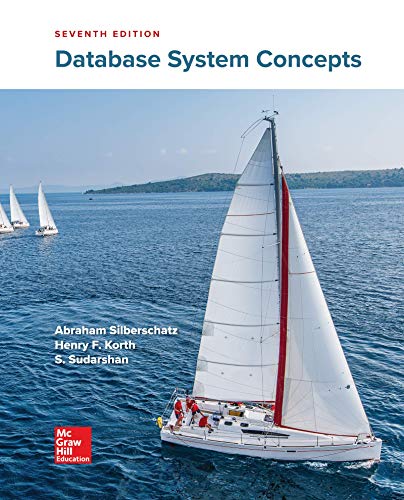
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
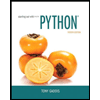
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
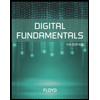
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
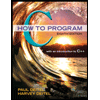
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
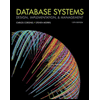
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
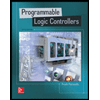
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education