Java Programming: Add the components needed for lexer.java according to the rubric attached.The goal is to make sure to read the shank.txt file as a series of tokens & show the shank.txt printed in console of eclipse and the image of the output is attached. Make sure the lexer has all the state machines. Make sure to have main.java. There must be no error in the lexer at all. Below is the lexer.java and token.java. Lexer.java package mypack; import java.util.ArrayList; import java.util.List; public class Lexer { private static final int INTEGER_STATE = 1; private static final int DECIMAL_STATE = 2; private static final int IDENTIFIER_STATE = 3; private static final int ERROR_STATE = 4; private static final char EOF = (char) -1; private static String input; private static int index; private static char currentChar; public List lex(String inputString) throws Exception { input = inputString; index = 0; currentChar = input.charAt(index); List tokens = new ArrayList(); while (currentChar != EOF) { switch (currentState()) { case INTEGER_STATE: integerState(tokens); break; case DECIMAL_STATE: decimalState(tokens); break; case IDENTIFIER_STATE: identifierState(tokens); break; case ERROR_STATE: throw new Exception("Invalid character: " + currentChar); default: break; } } return tokens; } private static int currentState() { if (Character.isDigit(currentChar)) { return INTEGER_STATE; } else if (Character.isLetter(currentChar)) { return IDENTIFIER_STATE; } else if (currentChar == '.') { return DECIMAL_STATE; } else { return ERROR_STATE; } } private static void integerState(List tokens) { StringBuilder builder = new StringBuilder(); while (Character.isDigit(currentChar)) { builder.append(currentChar); advance(); } tokens.add(new Token(Token.TokenType.NUMBER, builder.toString())); } private static void decimalState(List tokens) { StringBuilder builder = new StringBuilder(); builder.append(currentChar); advance(); while (Character.isDigit(currentChar)) { builder.append(currentChar); advance(); } tokens.add(new Token(Token.TokenType.NUMBER, builder.toString())); } private static void identifierState(List tokens) { StringBuilder builder = new StringBuilder(); while (Character.isLetterOrDigit(currentChar)) { builder.append(currentChar); advance(); } tokens.add(new Token(Token.TokenType.WORD, builder.toString())); } private static void advance() { index++; if (index >= input.length()) { currentChar = EOF; } else { currentChar = input.charAt(index); } } } Token.java package mypack; public class Token { public enum TokenType { WORD, NUMBER, SYMBOL } public TokenType tokenType; private String value; public Token(TokenType type, String val) { this.tokenType = type; this.value = val; } public TokenType getTokenType() { return this.tokenType; } public String toString() { return this.tokenType + ": " + this.value; } } shank.txt Fibonoacci (Iterative) define add (num1,num2:integer var sum : integer) variable counter : integer Finonacci(N) int N = 10; while counter < N define start () variables num1,num2,num3 : integer add num1,num2,var num3 {num1 and num2 are added together to get num3} num1 = num2; num2 = num3; counter = counter + 1; GCD (Recursive) define add (int a,int b : gcd) if b = 0 sum = a sum gcd(b, a % b) GCD (Iterative) define add (inta, intb : gcd) if a = 0 sum = b if b = 0 sum = a while counter a != b if a > b a = a - b; else b = b - a; sum = a; variables a,b : integer a = 60 b = 96 subtract a,b
Java
Lexer.java
package mypack;
import java.util.ArrayList;
import java.util.List;
public class Lexer {
private static final int INTEGER_STATE = 1;
private static final int DECIMAL_STATE = 2;
private static final int IDENTIFIER_STATE = 3;
private static final int ERROR_STATE = 4;
private static final char EOF = (char) -1;
private static String input;
private static int index;
private static char currentChar;
public List<Token> lex(String inputString) throws Exception {
input = inputString;
index = 0;
currentChar = input.charAt(index);
List<Token> tokens = new ArrayList<Token>();
while (currentChar != EOF) {
switch (currentState()) {
case INTEGER_STATE:
integerState(tokens);
break;
case DECIMAL_STATE:
decimalState(tokens);
break;
case IDENTIFIER_STATE:
identifierState(tokens);
break;
case ERROR_STATE:
throw new Exception("Invalid character: " + currentChar);
default:
break;
}
}
return tokens;
}
private static int currentState() {
if (Character.isDigit(currentChar)) {
return INTEGER_STATE;
} else if (Character.isLetter(currentChar)) {
return IDENTIFIER_STATE;
} else if (currentChar == '.') {
return DECIMAL_STATE;
} else {
return ERROR_STATE;
}
}
private static void integerState(List<Token> tokens) {
StringBuilder builder = new StringBuilder();
while (Character.isDigit(currentChar)) {
builder.append(currentChar);
advance();
}
tokens.add(new Token(Token.TokenType.NUMBER, builder.toString()));
}
private static void decimalState(List<Token> tokens) {
StringBuilder builder = new StringBuilder();
builder.append(currentChar);
advance();
while (Character.isDigit(currentChar)) {
builder.append(currentChar);
advance();
}
tokens.add(new Token(Token.TokenType.NUMBER, builder.toString()));
}
private static void identifierState(List<Token> tokens) {
StringBuilder builder = new StringBuilder();
while (Character.isLetterOrDigit(currentChar)) {
builder.append(currentChar);
advance();
}
tokens.add(new Token(Token.TokenType.WORD, builder.toString()));
}
private static void advance() {
index++;
if (index >= input.length()) {
currentChar = EOF;
} else {
currentChar = input.charAt(index);
}
}
}
Token.java
package mypack;
public class Token {
public enum TokenType {
WORD,
NUMBER,
SYMBOL
}
public TokenType tokenType;
private String value;
public Token(TokenType type, String val) {
this.tokenType = type;
this.value = val;
}
public TokenType getTokenType() {
return this.tokenType;
}
public String toString() {
return this.tokenType + ": " + this.value;
}
}
shank.txt
Fibonoacci (Iterative)
define add (num1,num2:integer var sum : integer)
variable counter : integer
Finonacci(N)
int N = 10;
while counter < N
define start ()
variables num1,num2,num3 : integer
add num1,num2,var num3
{num1 and num2 are added together to get num3}
num1 = num2;
num2 = num3;
counter = counter + 1;
GCD (Recursive)
define add (int a,int b : gcd)
if b = 0
sum = a
sum gcd(b, a % b)
GCD (Iterative)
define add (inta, intb : gcd)
if a = 0
sum = b
if b = 0
sum = a
while counter a != b
if a > b
a = a - b;
else
b = b - a;
sum = a;
variables a,b : integer
a = 60
b = 96
subtract a,b



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Where is the lexer.Java file? The rubric is given for the tutor to add all the needed components the lexer.Java needs to have which the lexer file I originally provided in the question. Write a lexer.Java code with all the components that is attached in the rubric with a main.Java that uses File.readAllLines() method to print out the shank.txt in the console of eclipse. The image of how the output is attached to the original problem as well.
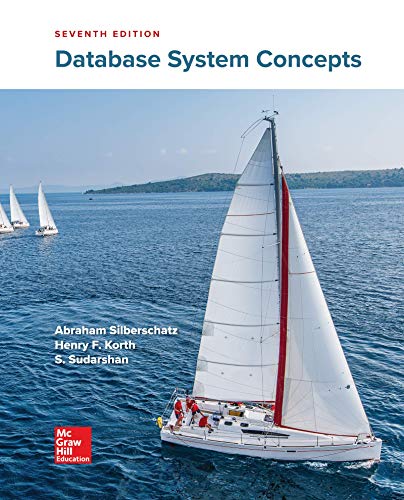
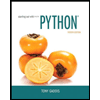
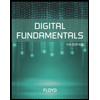
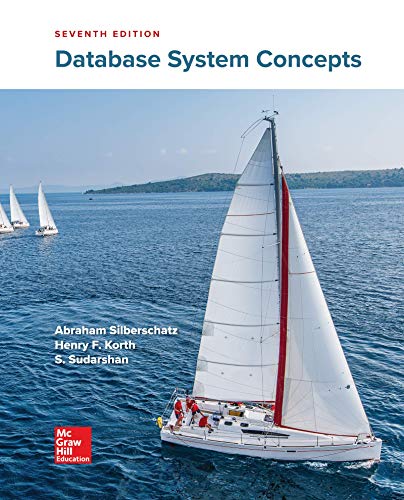
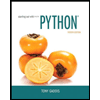
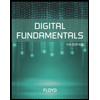
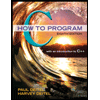
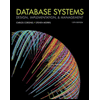
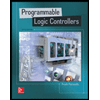