Whenever I rung my code and add an argument, for this case Rectangle, it will give me a java.io.FileNotFoundException: Rectangle (The system cannot find the file specified) at java.base/java.io.FileInputStream.open0(Native Method) at java.base/java.io.FileInputStream.open(FileInputStream.java:211) at java.base/java.io.FileInputStream.(FileInputStream.java:153) at java.base/java.util.Scanner.(Scanner.java:639) at Project3.main(Project3.java:135) What can I do to fix this? here is my code
Whenever I rung my code and add an argument, for this case Rectangle, it will give me a
java.io.FileNotFoundException: Rectangle (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:211)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:153)
at java.base/java.util.Scanner.<init>(Scanner.java:639)
at Project3.main(Project3.java:135)
What can I do to fix this? here is my code
import java.io.File;
import java.io.FileNotFoundException;
import java.util.ArrayList;
import java.util.Scanner;
public class Project3 {
public abstract class Shape {
protected String name;
abstract String getName();
abstract double getArea();
}
public class Rectangle extends Shape{
private double width;
private double length;
protected String name;
public Rectangle(double width, double length, String name) {
this.width = width;
this.length = length;
this.name=name;
}
@Override
String getName() {
return name;
}
@Override
double getArea() {
return width * length;
}
}
public class Square extends Rectangle{
private double side;
public Square(double width, double length, String name) {
super(width, length, name);
this.side =width;
}
@Override
String getName() {
// TODO Auto-generated method stub
return name;
}
@Override
double getArea() {
// TODO Auto-generated method stub
return side*side;
}
}
public class Triangle extends Shape{
private double base;
private double height;
public Triangle(double base,double height,String name) {
this.name = name;
this.base = base;
this.height = height;
}
@Override
String getName() {
return name;
}
@Override
double getArea() {
double area = (base* height)/2;
return area;
}
}
public class Circle extends Shape{
private double radius;
public Circle(double radius,String name) {
this.radius = radius;
this.name = name;
}
@Override
String getName() {
return name;
}
@Override
double getArea() {
return Math.PI * Math.pow(radius, 2);
}
}
static ArrayList<Shape> shapeList = new ArrayList<>();
public static void main(String[] args){
Project3 project3= new Project3();
String fileName = args[0];
//String fileName = "documentation.txt";
try {
File myObj = new File(fileName);
Scanner myReader = new Scanner(myObj);
while (myReader.hasNextLine()) {
String data = myReader.nextLine();
String[] tokens = data.split(",");
if(tokens[0].equals("Rectangle")){
if(tokens.length>=4){
String name = tokens[1];
double width= Double.parseDouble(tokens[2]);
double length = Double.parseDouble(tokens[3]);
if(width>0 && length>0){
Rectangle rectangle = project3.new Rectangle(width, length, name);
shapeList.add(rectangle);
}
}
}else if(tokens[0].equals("Square")){
if(tokens.length>=3){
String name = tokens[1];
double base = Double.parseDouble(tokens[2]);
if(base>0){
Square square = project3.new Square(base, base, name);
shapeList.add(square);
}
}
}else if(tokens[0].equals("Triangle")){
if(tokens.length>=4){
String name = tokens[1];
double base= Double.parseDouble(tokens[2]);
double height = Double.parseDouble(tokens[3]);
if(base>0 && height>0){
Triangle triangle = project3.new Triangle(base, height, name);
shapeList.add(triangle);
}
}
}else if(tokens[0].equals("Circle")){
if(tokens.length>=3){
String name = tokens[1];
double radius= Double.parseDouble(tokens[2]);
if(radius>0){
Circle circle = project3.new Circle(radius, name);
shapeList.add(circle);
}
}
}
}
myReader.close();
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
System.out.println("Cumulative Area: "+project3.getCumulativeArea());
System.out.println("Largest Shape: "+project3.getLargestShape());
}
public double getCumulativeArea(){
double cummulativeArea=0;
for(Shape shape:shapeList){
cummulativeArea = cummulativeArea + shape.getArea();
}
return cummulativeArea;
}
public String getLargestShape(){
double largestArea = 0;
String shapeName = "There are no shapes";
if(shapeList.size()>0){
largestArea = shapeList.get(0).getArea();
shapeName = shapeList.get(0).getName();
}
for(Shape shape:shapeList){
if(shape.getArea()> largestArea){
largestArea = shape.getArea();
shapeName = shape.getName();
}
}
return shapeName;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

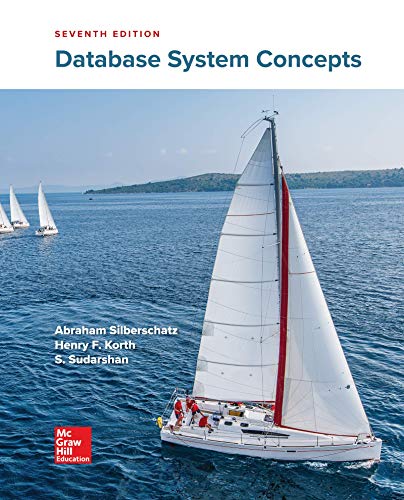
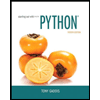
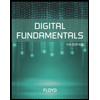
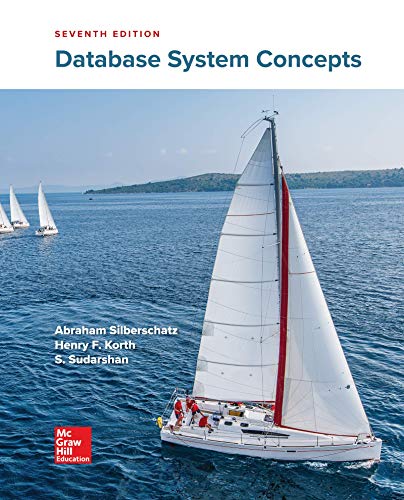
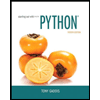
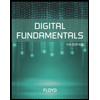
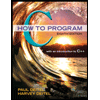
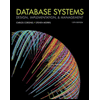
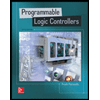