Write a program IN JAVA that replaces every “Java” word in a file into the word “HTML” and saves the file in the same location on disk after printing both the old lines and new lines to the output. The input file is passed to the program as a command line argument
Write a program IN JAVA that replaces every “Java” word in a file into the word “HTML” and saves
the file in the same location on disk after printing both the old lines and new lines to the
output. The input file is passed to the program as a command line argument.
Sample file: input.txt
Welcome to Java!
This is a Java program.
I like
Use the following statements to create a file instance and make a scanner for it:
// Create a File instance
java.io.File file = new java.io.File("inputFileName.txt");
// Create a Scanner for the file
Scanner input = new Scanner(file);
// Read data from a file using input, e.g. input.next()
// input.hasNext() determines if there's more data in the file
Use the following statements to write to a file:
// Create a File instance
java.io.File file = new java.io.File("outputFileName.txt");
// Create the file
java.io.PrintWriter output = new java.io.PrintWriter(file);
// Write output to the file, this can be repeated
output.print(someString);
// Close the file
output.close();

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

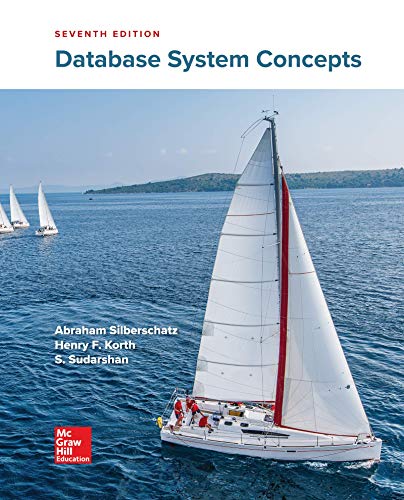
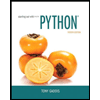
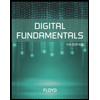
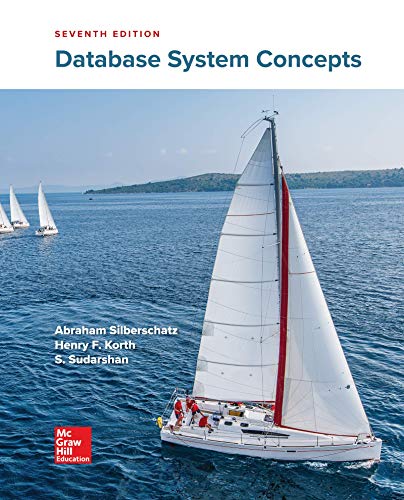
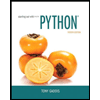
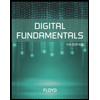
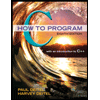
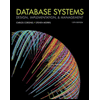
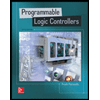