Whenever I create an input text file and run the application I get this error message. Exception in thread "main" java.lang.NumberFormatException: empty String at java.base/jdk.internal.math.FloatingDecimal.readJavaFormatString(FloatingDecimal.java:1842) at java.base/jdk.internal.math.FloatingDecimal.parseDouble(FloatingDecimal.java:110) at java.base/java.lang.Double.parseDouble(Double.java:651) at javaapplication3.RegularPolygonMain.main(RegularPolygonMain.java:30) How do I fix this? Code: File 1: package javaapplication3; public class RegularPolygon { private int numSides; private double side; private double x; private double y; RegularPolygon() { this.numSides = 3; this.side = 1; this.x = this.y = 0; } RegularPolygon(int sides, double sideLength) { this.numSides = sides; this.side = sideLength; this.x = this.y = 0; } RegularPolygon(int sides, double eachSideLen, double x1, double y1) { this.numSides = sides; this.side = eachSideLen; this.x = x1; this.y = y1; } public int getNumSides() { return numSides; } public void setNumSides(int numSides) { this.numSides = numSides; } public double getSide() { return side; } public void setSide(double side) { this.side = side; } public double getX() { return x; } public void setX(double x) { this.x = x; } public double getY() { return y; } public void setY(double y) { this.y = y; } public double perimeter() { return side * numSides; } public double area() { return (numSides * Math.pow(side, 2)) / (4 * Math.tan(Math.PI / numSides)); } } File 2: package javaapplication3; import java.io.File; import java.io.IOException; import java.util.Scanner; public class RegularPolygonMain { private static final String FILE_NAME = "input.txt"; public static void main(String[] args) { try (Scanner reader = new Scanner(new File(FILE_NAME))){ while (reader.hasNext()) { String line = reader.nextLine(); line = line.trim(); String[] values = line.split(""); RegularPolygon p; int numSides = Integer.parseInt(values[0]); double sideLength = Double.parseDouble(values[1]); if (values.length == 2) { p = new RegularPolygon(numSides, sideLength); } else { int x = Integer.parseInt(values[2]); int y = Integer.parseInt(values[3]); p = new RegularPolygon(numSides, sideLength, x, y); } printPolygonDetails(p); } } catch (IOException e) { System.out.println(e.getMessage()); } } private static void printPolygonDetails(RegularPolygon p) { System.out.print( p.getNumSides() + " " + p.getSide() + " (" + p.getX() + "," + p.getY() + ") " + p.perimeter() + " "); System.out.println(String.format("%.2f", +p.area())); } }
Whenever I create an input text file and run the application I get this error message.
Exception in thread "main" java.lang.NumberFormatException: empty String at java.base/jdk.internal.math.FloatingDecimal.readJavaFormatString(FloatingDecimal.java:1842) at java.base/jdk.internal.math.FloatingDecimal.parseDouble(FloatingDecimal.java:110) at java.base/java.lang.Double.parseDouble(Double.java:651) at javaapplication3.RegularPolygonMain.main(RegularPolygonMain.java:30)
How do I fix this?
Code:
File 1:
package javaapplication3;
public class RegularPolygon {
private int numSides;
private double side;
private double x;
private double y;
RegularPolygon() {
this.numSides = 3;
this.side = 1;
this.x = this.y = 0;
}
RegularPolygon(int sides, double sideLength) {
this.numSides = sides;
this.side = sideLength;
this.x = this.y = 0;
}
RegularPolygon(int sides, double eachSideLen, double x1, double y1) {
this.numSides = sides;
this.side = eachSideLen;
this.x = x1;
this.y = y1;
}
public int getNumSides() {
return numSides;
}
public void setNumSides(int numSides) {
this.numSides = numSides;
}
public double getSide() {
return side;
}
public void setSide(double side) {
this.side = side;
}
public double getX() {
return x;
}
public void setX(double x) {
this.x = x;
}
public double getY() {
return y;
}
public void setY(double y) {
this.y = y;
}
public double perimeter() {
return side * numSides;
}
public double area() {
return (numSides * Math.pow(side, 2)) / (4 * Math.tan(Math.PI / numSides));
}
}
File 2:
package javaapplication3;
import java.io.File;
import java.io.IOException;
import java.util.Scanner;
public class RegularPolygonMain {
private static final String FILE_NAME = "input.txt";
public static void main(String[] args) {
try (Scanner reader = new Scanner(new File(FILE_NAME))){
while (reader.hasNext()) {
String line = reader.nextLine();
line = line.trim();
String[] values = line.split("");
RegularPolygon p;
int numSides = Integer.parseInt(values[0]);
double sideLength = Double.parseDouble(values[1]);
if (values.length == 2) {
p = new RegularPolygon(numSides, sideLength);
} else {
int x = Integer.parseInt(values[2]);
int y = Integer.parseInt(values[3]);
p = new RegularPolygon(numSides, sideLength, x, y);
}
printPolygonDetails(p);
}
} catch (IOException e) {
System.out.println(e.getMessage());
}
}
private static void printPolygonDetails(RegularPolygon p) {
System.out.print(
p.getNumSides() + " " + p.getSide() + " (" + p.getX() + "," + p.getY() + ") " + p.perimeter() + " ");
System.out.println(String.format("%.2f", +p.area()));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

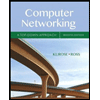
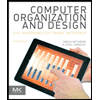
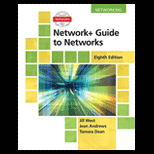
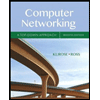
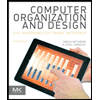
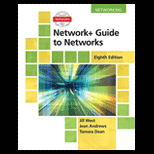
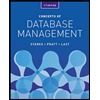
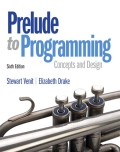
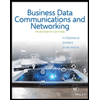