What would the required pseudocode look like for the below C++ program? Please and thank you Program source code: #include <iostream> #include <string> using namespace std; // Function to concatenate the strings together std::string concatenateStrings(const std::string& str1, const std::string& str2) { std::string result = str1; result.append(str2); return result; } int main() { // Loop to take user's input three times for (int i=0; i <3; ++i) { // Prompt user for first string input std::cout << "Please enter a string: "; std::string input1; std::getline(std::cin, input1); // Allowing spaces in input // Prompt user for second string input std::cout << "Please enter another string: "; std::string input2; std::getline(std::cin, input2); // Allowing spaces in input // Concatenate the strings std:: string result = concatenateStrings(input1, input2); // Print the concatenated result std::cout << "Your concatenated string result: " << result << "\n\n"; } return 0; }
What would the required pseudocode look like for the below C++
Please and thank you
Program source code:
#include <iostream>
#include <string>
using namespace std;
// Function to concatenate the strings together
std::string concatenateStrings(const std::string& str1, const std::string& str2) {
std::string result = str1;
result.append(str2);
return result;
}
int main() {
// Loop to take user's input three times
for (int i=0; i <3; ++i) {
// Prompt user for first string input
std::cout << "Please enter a string: ";
std::string input1;
std::getline(std::cin, input1); // Allowing spaces in input
// Prompt user for second string input
std::cout << "Please enter another string: ";
std::string input2;
std::getline(std::cin, input2); // Allowing spaces in input
// Concatenate the strings
std:: string result = concatenateStrings(input1, input2);
// Print the concatenated result
std::cout << "Your concatenated string result: " << result << "\n\n";
}
return 0;
}
Unlock instant AI solutions
Tap the button
to generate a solution
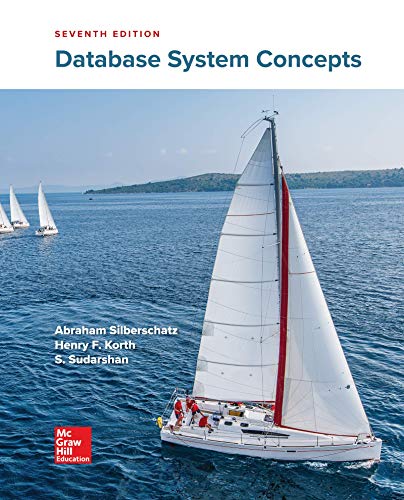
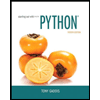
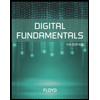
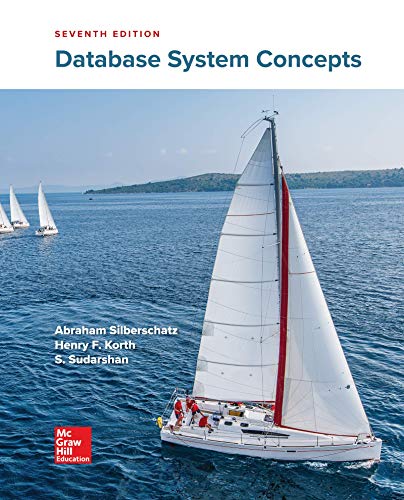
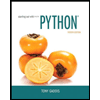
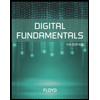
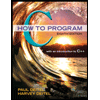
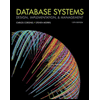
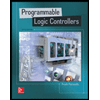