What is/are the dimension(s) of the array m? What is the length of the array r? What does r[2] represent? What does m[0].length stands for? Can you explain how the average was calculated?
What is/are the dimension(s) of the array m? What is the length of the array r? What does r[2] represent? What does m[0].length stands for? Can you explain how the average was calculated?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
What is/are the dimension(s) of the array m?
What is the length of the array r?
What does r[2] represent?
What does m[0].length stands for?
Can you explain how the average was calculated?
![```java
import java.util.Scanner;
public class Pass2D {
public static void main(String[] args) {
// Get an array from the user
int[][] m = getArray();
// Display array elements
System.out.println("\nYou provided the following array " + java.util.Arrays.deepToString(m));
// Display array characteristics
int[] r = findCharacteristics(m);
System.out.println("The minimum value is: " + r[0] +
", the maximum value is: " + r[1] +
", the average is: " + r[2] * 1.0/(m.length * m[0].length));
}
// this method finds array characteristics
public static int[] findCharacteristics(int[][] m) {
int min = m[0][0];
int max = m[0][0];
int sum = 0;
for (int[] arr : m) {
for (int element : arr) {
if (element < min) min = element;
if (element > max) max = element;
sum += element;
}
}
// create a new array to be returned
int[] c = new int[3];
c[0] = min;
c[1] = max;
c[2] = sum;
return c;
}
// this method creates an array based on the user input
public static int[][] getArray() {
// Create a Scanner
Scanner input = new Scanner(System.in);
System.out.println("Provide an integer for a number of rows: ");
int row = input.nextInt();
System.out.println("Provide an integer for a number of columns: ");
int col = input.nextInt();
// Create an array
int[][] m = new int[row][col];
System.out.println("Enter a " + m.length + " x " + m[0].length + " matrix and press Enter: ");
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m[i].length; j++)
m[i][j] = input.nextInt();
}
input.close();
return m;
}
}
```
**Explanation of the Code:**
1.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2ec814c7-90f3-4435-9bb8-fcbab3f733ac%2F75c26b24-bae9-4e62-a836-3d89cb37eecc%2Fz8ya1_processed.png&w=3840&q=75)
Transcribed Image Text:```java
import java.util.Scanner;
public class Pass2D {
public static void main(String[] args) {
// Get an array from the user
int[][] m = getArray();
// Display array elements
System.out.println("\nYou provided the following array " + java.util.Arrays.deepToString(m));
// Display array characteristics
int[] r = findCharacteristics(m);
System.out.println("The minimum value is: " + r[0] +
", the maximum value is: " + r[1] +
", the average is: " + r[2] * 1.0/(m.length * m[0].length));
}
// this method finds array characteristics
public static int[] findCharacteristics(int[][] m) {
int min = m[0][0];
int max = m[0][0];
int sum = 0;
for (int[] arr : m) {
for (int element : arr) {
if (element < min) min = element;
if (element > max) max = element;
sum += element;
}
}
// create a new array to be returned
int[] c = new int[3];
c[0] = min;
c[1] = max;
c[2] = sum;
return c;
}
// this method creates an array based on the user input
public static int[][] getArray() {
// Create a Scanner
Scanner input = new Scanner(System.in);
System.out.println("Provide an integer for a number of rows: ");
int row = input.nextInt();
System.out.println("Provide an integer for a number of columns: ");
int col = input.nextInt();
// Create an array
int[][] m = new int[row][col];
System.out.println("Enter a " + m.length + " x " + m[0].length + " matrix and press Enter: ");
for (int i = 0; i < m.length; i++) {
for (int j = 0; j < m[i].length; j++)
m[i][j] = input.nextInt();
}
input.close();
return m;
}
}
```
**Explanation of the Code:**
1.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
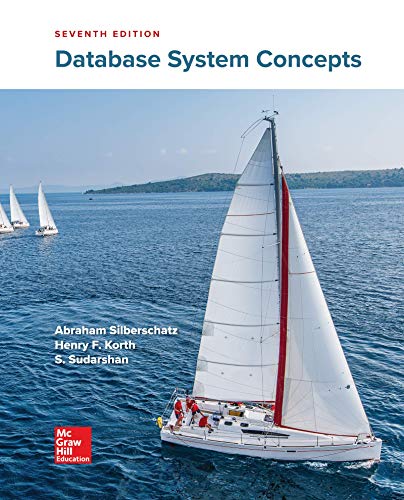
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
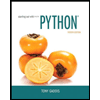
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
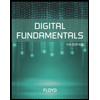
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
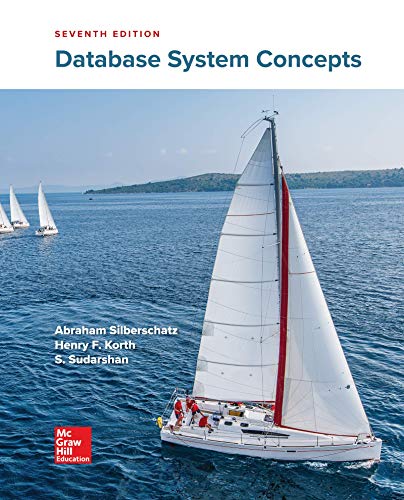
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
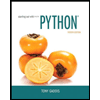
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
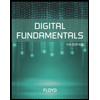
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
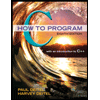
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
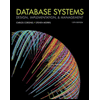
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
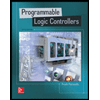
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education