Write a program in python Using swarm basic optimization with arguments to find a minimum of Rosenbrook function, To find the minimum of the test function y = x – 2sin(x). set up the bound for the value [-5.12, 5.12]. Use Swarm to find the value of X which minimizes the test function. Use the default hyperparameters. # hyperparameters options = {'c1': 0.5, 'c2': 0.3, 'w':0.9}
Write a program in python Using swarm basic optimization with arguments to find a minimum of Rosenbrook function, To find the minimum of the test function y = x – 2sin(x).
set up the bound for the value [-5.12, 5.12].
Use Swarm to find the value of X which minimizes the test function.
Use the default hyperparameters.
# hyperparameters
options = {'c1': 0.5, 'c2': 0.3, 'w':0.9}
![Using Arguments
Arguments can either be passed in using a tuple or a dictionary, using the kwargs={} paradigm. First
lets optimize the Rosenbrock function using keyword arguments. Note in the definition of the
Rosenbrock function above, there were two arguments that need to be passed other than the
design variables, and one optional keyword argument, a, b, and c, respectively
[7]: from pyswarms.single.global_best import GlobalBest PSO
#instatiate the optimizer
x_max = 10 np.ones (2)
x_min = -1 * x_max
*
bounds = (x_min, x_max)
options {'c1': 0.5, 'c2': 0.3, 'w': 0.9}
optimizer = GlobalBest PSO(n_particles=10, dimensions=2, options=options, bounds-bounds)
# now run the optimization, pass a=1 and b=100 as a tuple assigned to args
cost, pos = optimizer.optimize(rosenbrock_with_args, 1000, a=1, b=100, c=0)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2Fb81a5524-dbcb-45f1-bd84-354f980f3fc3%2Fcywhn8b_processed.png&w=3840&q=75)
![Basic Optimization with Arguments
Here, we will run a basic optimization using an objective function that needs parameterization. We
will use the single. GBestPSO and a version of the rosenbrock function to demonstrate
[6]: # import modules
import numpy as np
# create a parameterized version of the classic Rosenbrock unconstrained optimzation function
def rosenbrock_with_args(x, a, b, c=0):
f = (a - x[:, 0]) ** 2 + b * (x[:, 1] x[:, 0] ** 2) ** 2 + c
return f](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84b9759d-3bd7-481b-9da5-01ee09be0953%2Fb81a5524-dbcb-45f1-bd84-354f980f3fc3%2Fxmbn7cq_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

What if the swarm was 2-dimentional and the max bound was 10* np.ones(2) and minimum bound = -1 *max.
How can I write a helper function that, given a
every element’s value is sine of the corresponding element’s value in A. For instance,
given array A=(e1 e2, e3), the helper function will return an array B=(sine(e1), sine(e2),
sine(e3)).
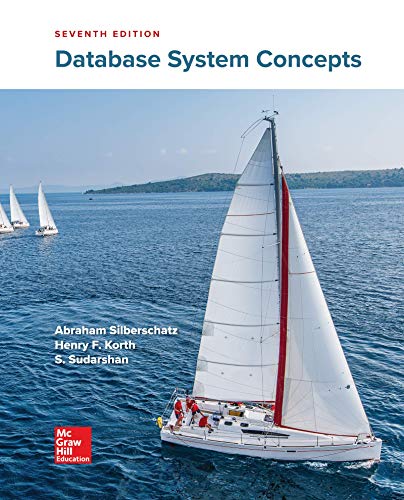
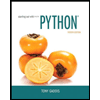
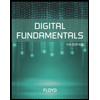
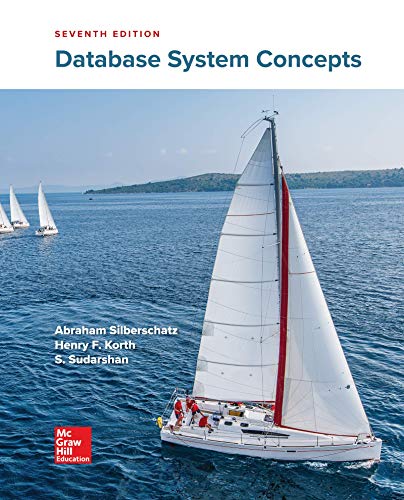
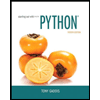
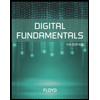
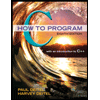
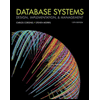
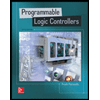