We will need to sort this. The following function is called a Bubble Sort. Explain what the code is doing below. bubblesort <- function(x) { if (length(x) < 2) return (x) for(last in length (x):2) { for (first in 1: (last - 1)) { if(x[first] > x[first + 1]) { save <- x[first] x[first] <- x[first + 1] x[first + 1] <- save } } return (x) }
We will need to sort this. The following function is called a Bubble Sort. Explain what the code is doing below. bubblesort <- function(x) { if (length(x) < 2) return (x) for(last in length (x):2) { for (first in 1: (last - 1)) { if(x[first] > x[first + 1]) { save <- x[first] x[first] <- x[first + 1] x[first + 1] <- save } } return (x) }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
We will need to sort this. The following function is called a Bubble Sort (see attached image). Explain what the code in R is doing below (in words). Please be detailed in your response.
![Here is a transcription and explanation of a Bubble Sort function in code, suitable for an educational website:
---
**Understanding Bubble Sort**
Bubble Sort is a simple sorting algorithm that works by repeatedly stepping through the list to be sorted, comparing each pair of adjacent items and swapping them if they are in the wrong order. The pass-through is repeated until no swaps are needed, which means the list is sorted.
Below is a Bubble Sort function in a programming language:
```javascript
bubblesort <- function(x) {
if (length(x) < 2) return (x)
for(last in length(x):2) {
for(first in 1:(last - 1)) {
if(x[first] > x[first + 1]) {
save <- x[first]
x[first] <- x[first + 1]
x[first + 1] <- save
}
}
}
return (x)
}
```
**Explanation:**
- **Function Definition:** The function `bubblesort` takes a single argument `x`, which is expected to be a list or array of numbers.
- **Base Case Check:** The line `if (length(x) < 2) return (x)` checks if the list has fewer than two elements. If true, it returns the list as is, since lists with zero or one element are already sorted.
- **Outer Loop (`for(last in length(x):2)`):** This loop iterates backward from the end of the list towards the beginning. The variable `last` represents the boundary of the unsorted portion of the list, reducing with each full pass.
- **Inner Loop (`for(first in 1:(last - 1))`):** This loop goes through each adjacent pair up to the `last` index. The variable `first` represents the current index being compared.
- **Swap Condition:** The condition `if(x[first] > x[first + 1])` checks if the current element is greater than the next element. If true, it performs a swap, storing the value temporarily in `save`, then swapping the values of `x[first]` and `x[first + 1]`.
- **Final Return:** After all necessary swaps have been performed, the sorted list `x` is returned.
This method is called "Bubble Sort" because smaller elements "bubble" to the top of the list with each pass through the data.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8c2ff348-a0ac-49b4-af78-d8b182dcdea2%2F8f509bdb-598f-4474-b943-8ce9dd17278f%2F44sq16g_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Here is a transcription and explanation of a Bubble Sort function in code, suitable for an educational website:
---
**Understanding Bubble Sort**
Bubble Sort is a simple sorting algorithm that works by repeatedly stepping through the list to be sorted, comparing each pair of adjacent items and swapping them if they are in the wrong order. The pass-through is repeated until no swaps are needed, which means the list is sorted.
Below is a Bubble Sort function in a programming language:
```javascript
bubblesort <- function(x) {
if (length(x) < 2) return (x)
for(last in length(x):2) {
for(first in 1:(last - 1)) {
if(x[first] > x[first + 1]) {
save <- x[first]
x[first] <- x[first + 1]
x[first + 1] <- save
}
}
}
return (x)
}
```
**Explanation:**
- **Function Definition:** The function `bubblesort` takes a single argument `x`, which is expected to be a list or array of numbers.
- **Base Case Check:** The line `if (length(x) < 2) return (x)` checks if the list has fewer than two elements. If true, it returns the list as is, since lists with zero or one element are already sorted.
- **Outer Loop (`for(last in length(x):2)`):** This loop iterates backward from the end of the list towards the beginning. The variable `last` represents the boundary of the unsorted portion of the list, reducing with each full pass.
- **Inner Loop (`for(first in 1:(last - 1))`):** This loop goes through each adjacent pair up to the `last` index. The variable `first` represents the current index being compared.
- **Swap Condition:** The condition `if(x[first] > x[first + 1])` checks if the current element is greater than the next element. If true, it performs a swap, storing the value temporarily in `save`, then swapping the values of `x[first]` and `x[first + 1]`.
- **Final Return:** After all necessary swaps have been performed, the sorted list `x` is returned.
This method is called "Bubble Sort" because smaller elements "bubble" to the top of the list with each pass through the data.
Expert Solution

Step 1 :-
- The question is to explain the functioning of the code of R language.
Step by step
Solved in 2 steps

Recommended textbooks for you
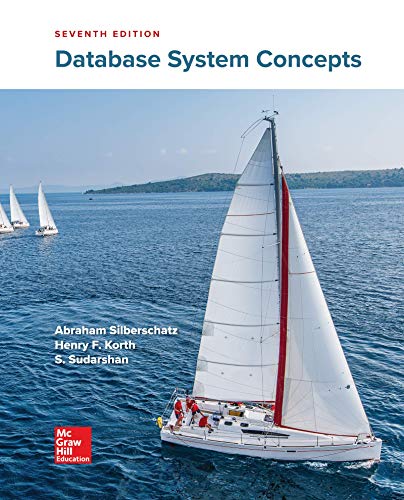
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
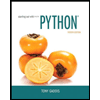
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
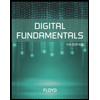
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
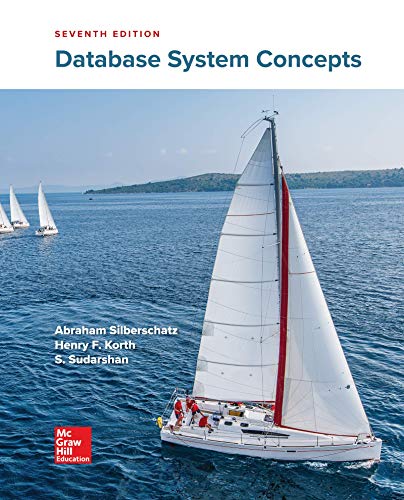
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
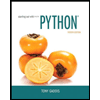
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
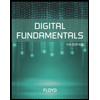
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
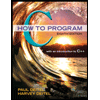
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
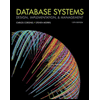
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
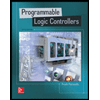
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education