Write the four sorting functions and the three comparison functionsnecessary to perform the following actions. No need to write the algorithmfor this problem.● Sort an array of integers in ascending order.● Sort an array of doubles in descending order.● Sort an array of characters in ascending order.In the main program call your functions to sort the three arrays alreadydeclared and initialized.Note: You must use the qsort function as presented in the slides. Youcannot use any other method to sort the arrays.intmain (void) {int array1[8] = {67, 98, 23, 11, 47, 13, 94, 58};double array2[8] = {-6.4, 2.65, 32.745, -3.9, 2.2, 11.742, -23.523,0.0};char array3[8] = {'a', 'G', '?', 'm', '#', 'B', 'n', '%'};// sort array1 in ascending order and print the resulting array.// sort array2 in descending order and print the resulting array.// sort array3 in ascending order and print the resulting array.return (0);}
Write the four sorting functions and the three comparison functions
necessary to perform the following actions. No need to write the algorithm
for this problem.
● Sort an array of integers in ascending order.
● Sort an array of doubles in descending order.
● Sort an array of characters in ascending order.
In the main program call your functions to sort the three arrays already
declared and initialized.
Note: You must use the qsort function as presented in the slides. You
cannot use any other method to sort the arrays.
int
main (void) {
int array1[8] = {67, 98, 23, 11, 47, 13, 94, 58};
double array2[8] = {-6.4, 2.65, 32.745, -3.9, 2.2, 11.742, -23.523,
0.0};
char array3[8] = {'a', 'G', '?', 'm', '#', 'B', 'n', '%'};
// sort array1 in ascending order and print the resulting array.
// sort array2 in descending order and print the resulting array.
// sort array3 in ascending order and print the resulting array.
return (0);
}

Step by step
Solved in 1 steps

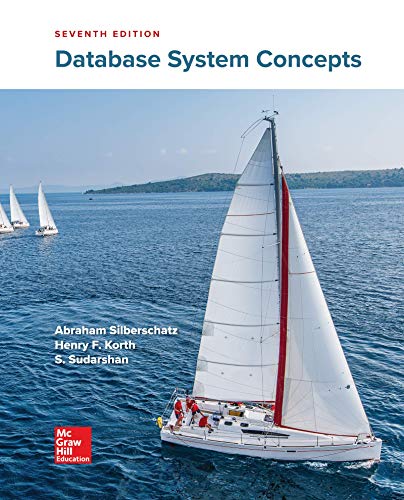
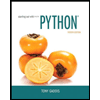
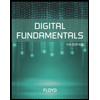
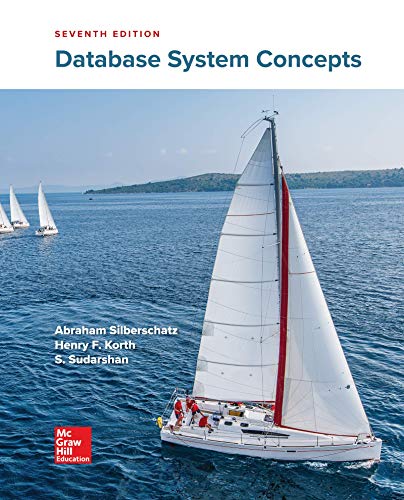
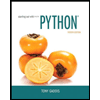
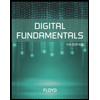
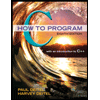
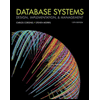
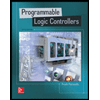