We want to create a program that reads integer values and displays the average of those that are even, and those that are odd. The program should accepts input values until the user enters 999. We have the following fill-in template: print("This program calculates the average of even and odd numbers.") # Initialize accumulators and counters total_even = total_odd = count_even = count_odd = # Process input values )) while ! = ....if % 2 == 0: .total_even += .....count_even += ....else: .total_odd += ..count_odd += )) # Calculate averages if >
We want to create a program that reads integer values and displays the average of those that are even, and those that are odd. The program should accepts input values until the user enters 999. We have the following fill-in template: print("This program calculates the average of even and odd numbers.") # Initialize accumulators and counters total_even = total_odd = count_even = count_odd = # Process input values )) while ! = ....if % 2 == 0: .total_even += .....count_even += ....else: .total_odd += ..count_odd += )) # Calculate averages if >
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![In this educational exercise, we provide a Python code snippet and a set of expressions. Your task is to drag and drop the appropriate expressions into the code to achieve the desired functionality.
Code Snippet:
```python
else:
....print("No even numbers input.")
if [_____] > [_____]:
....[_____] = [_____] / [_____]
....print("Average of odd numbers is", [_____])
else:
....print("No odd numbers input.")
```
Available Expressions:
- x
- avg_even
- 0
- avg_odd
- count_odd
- int
- 999
- total_even
- input
- 1
- total_odd
- -1
- count_even
Explanation:
- You are given a code snippet with placeholders where expressions need to be filled in.
- The objective is to complete the code such that it correctly calculates and displays the average of even numbers and odd numbers entered by the user.
- The expressions provided need to be accurately positioned in the code to ensure it works as intended.
Here's a breakdown of what each part of the code should accomplish:
1. **else block for even numbers**: If no even numbers are input, a message "No even numbers input." will be printed.
2. **if block for odd numbers**: Checks if the count of odd numbers is greater than zero.
- If true, it calculates the average of odd numbers.
- Then it prints the average.
- Otherwise, it prints "No odd numbers input."
This exercise helps in understanding conditionals and arithmetic operations in Python, and the importance of proper variable placement for efficient computation. Drag and drop the correct expressions into the placeholders to complete the program logic.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F47e293e7-9f62-4fc2-a86c-08857850f6fd%2F707524fe-c8e2-4d12-96fd-76688ed46630%2Fevzsbi_processed.png&w=3840&q=75)
Transcribed Image Text:In this educational exercise, we provide a Python code snippet and a set of expressions. Your task is to drag and drop the appropriate expressions into the code to achieve the desired functionality.
Code Snippet:
```python
else:
....print("No even numbers input.")
if [_____] > [_____]:
....[_____] = [_____] / [_____]
....print("Average of odd numbers is", [_____])
else:
....print("No odd numbers input.")
```
Available Expressions:
- x
- avg_even
- 0
- avg_odd
- count_odd
- int
- 999
- total_even
- input
- 1
- total_odd
- -1
- count_even
Explanation:
- You are given a code snippet with placeholders where expressions need to be filled in.
- The objective is to complete the code such that it correctly calculates and displays the average of even numbers and odd numbers entered by the user.
- The expressions provided need to be accurately positioned in the code to ensure it works as intended.
Here's a breakdown of what each part of the code should accomplish:
1. **else block for even numbers**: If no even numbers are input, a message "No even numbers input." will be printed.
2. **if block for odd numbers**: Checks if the count of odd numbers is greater than zero.
- If true, it calculates the average of odd numbers.
- Then it prints the average.
- Otherwise, it prints "No odd numbers input."
This exercise helps in understanding conditionals and arithmetic operations in Python, and the importance of proper variable placement for efficient computation. Drag and drop the correct expressions into the placeholders to complete the program logic.

Transcribed Image Text:## Program to Calculate the Average of Even and Odd Numbers
We want to create a program that reads integer values and displays the average of those that are even, and those that are odd. The program should accept input values until the user enters 999.
We have the following fill-in template:
```python
print("This program calculates the average of even and odd numbers.")
# Initialize accumulators and counters
total_even = total_odd = count_even = count_odd = ____(1)____
# Process input values
____(2)____ = ____(3)____(____(4)____(____(5)____))
while ____(6)____ != ____(7)____:
....if ____(8)____ % 2 == 0:
........total_even += ____(9)____
........count_even += ____(10)____
....else:
........total_odd += ____(11)____
........count_odd += ____(12)____
____(13)____ = ____(14)____(____(15)____(____(16)____))
# Calculate averages
if ____(17)____ > ____(18)____:
....____(19)____ = ____(20)____ / ____(21)____
....print("Average of even numbers is", ____(22)____)
else:
....print("No even numbers were entered.")
if ____(23)____ > ____(24)____:
....____(25)____ = ____(26)____ / ____(27)____
....print("Average of odd numbers is", ____(28)____)
else:
....print("No odd numbers were entered.")
```
### Diagram Explanation
This template contains a Python script skeleton designed to calculate and print the average of even and odd numbers. Let's break down its structure:
1. **Initialization of Variables (Line 4)**: This line initializes four variables (`total_even`, `total_odd`, `count_even`, `count_odd`) to zero. These will keep track of the sums and counts of even and odd numbers.
2. **Input Capture (Lines 6, 7, 8)**: A prompt captures user input, which is converted into an integer and stored in a variable. This captured value is then checked in a loop (`while`) until it equals `999`.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
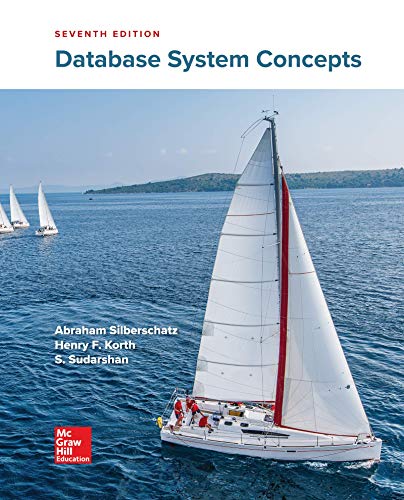
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
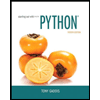
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
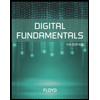
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
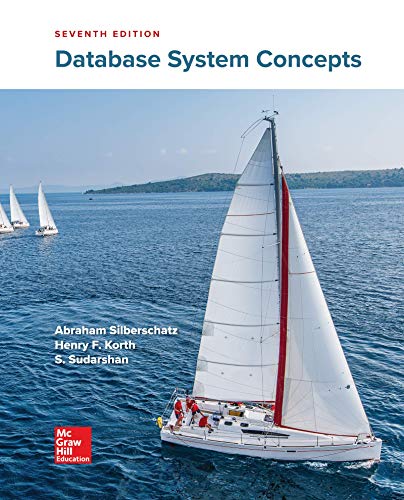
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
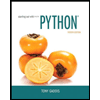
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
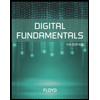
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
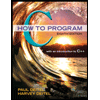
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
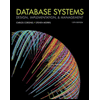
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
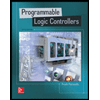
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education