Using the MorseCode.csv file, read the file and process the comma separated variables. See the Elements.py file for an example of reading a CSV file. File excerpt: A,.- B,-... C,-.-. D,-.. E,. * Design a Morse Code class to contain ASCII and Morse Code characters. For example, the Morse Code for the letter 'C' is "-.-.". * Add the Dunders to the Morse Code class to support: initialization, iterating, printing, searching, sorting. * Design a Morse Code collection class to contain the 39 Morse Code characters listed in the CSV file. The Morse Code collection class contains an internal Dictionary for storing each individual Morse Code characters. * Demonstrate your Dunders work correctly for initialization, iterating, printing, searching and sorting.
* Using the MorseCode.csv file, read the file and process the comma separated variables. See the Elements.py file for an example of reading a CSV file. File excerpt:
A,.-
B,-...
C,-.-.
D,-..
E,.
* Design a Morse Code class to contain ASCII and Morse Code characters. For example, the Morse Code for the letter 'C' is "-.-.".
* Add the Dunders to the Morse Code class to support: initialization, iterating, printing, searching, sorting.
* Design a Morse Code collection class to contain the 39 Morse Code characters listed in the CSV file. The Morse Code collection class contains an internal Dictionary for storing each individual Morse Code characters.
* Demonstrate your Dunders work correctly for initialization, iterating, printing, searching and sorting.
Morse Codes:
E .
T -
A .-
I ..
M --
N -.
D -..
G --.
K -.-
O ---
R .-.
S ...
U ..-
W .--
B -...
C -.-.
F ..-.
H ....
J .---
L .-..
P .--.
Q --.-
V ...-
X -..-
Y -.--
Z --..
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
Elements code:
class Element :
def __init__(self,nu,ab,na) : # constructor
self.number = int(nu)
self.abbrev = ab
self.name = na
def __str__(self): # string conversion operator
return str(self.name + '\t' + self.abbrev + '\t' + str(self.number))
def __lt__(self,right): # less-than operator
print(str(self.number) + "__lt__" + str(right.number))
return self.number < right.number
def __eq__(self,right): # equality operator
print(str(self.number) + "__eq__" + str(right.number))
return self.number == right.number
class PeriodicList :
def __init__(self): #constructor
self.table = []
def __getitem__(self,index):
print("__getitem__ index = ",index)
return self.table[index]
def __setitem__(self,index,value):
print("__setitem__ index = ",index,value)
self.table[index] = value
def __str__(self) :
stable = ""
for i in range(0,len(self.table)):
stable = stable + str(self.table[i])
return stable
def Sort(self):
self.table.sort()
def Reader(self,csvfile):
csv = open(csvfile)
for line in csv :
rline = line.rstrip()
cline = rline.split(',')
e = Element(int(cline[0]),cline[1],cline[2])
self.table.append(e)
def main():
pt = PeriodicList()
pt.Reader('ptable.csv')
print(pt) # sorted by name
pt.Sort()
print(pt) # sorted by number
efind = Element(80,"Hg","Mercury")
if efind in pt:
print("Found: ",efind)
if __name__=="__main__":
main()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

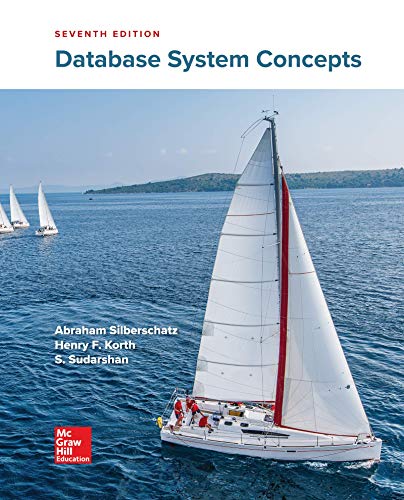
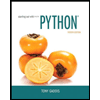
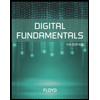
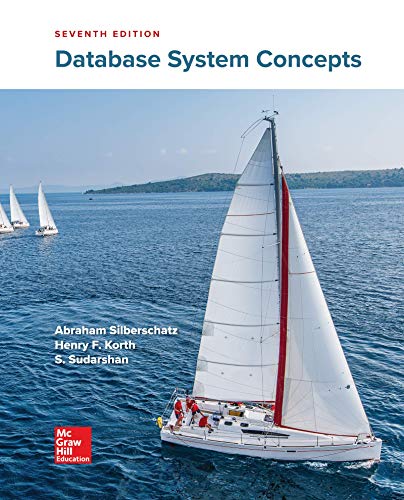
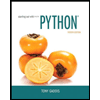
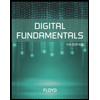
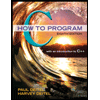
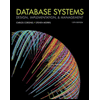
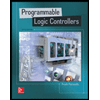