Using SceneBuilder and Java fx, code an an Elevator simulation using polymorphism and object-oriented programming Design. The simulation have 4 different types of elevators and passengers. There are 4 types of passengers in the system: Standard: This is the most common type of passenger and has a request percentage of 70%. Standard passengers have no special requirements. VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority and are more likely to be picked up by express elevators. Freight: This type of passenger has a request percentage of 15%. Freight passengers have large items that need to be transported and are more likely to be picked up by freight elevators. Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items that need to be transported and are more likely to be picked up by glass elevators. There are 4 types of elevators in the system: StandardElevator: This is the most common type of elevator and has a request percentage of 70%. Standard elevators have a maximum capacity of 10 passengers. ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a maximum capacity of 8 passengers and are faster than standard elevators. FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a maximum capacity of 5 passengers and are designed to transport heavy items. GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a maximum capacity of 6 passengers and are designed to transport fragile item
Help, I am not sure how to write this code. I been working on this for hours and it's due in few days. I don't need the answer right away. I don't mind if it's take two or three days. I got few answer for this question using this website but they didn't include Poylmorphism of passenger and elevator, scenebuilder, and javafx. Any help would be appreciated.
Using SceneBuilder and Java fx, code an an Elevator simulation using polymorphism and object-oriented
There are 4 types of passengers in the system:
Standard: This is the most common type of passenger and has a request percentage of 70%.
Standard passengers have no special requirements.
VIP: This type of passenger has a request percentage of 10%. VIP passengers are given priority
and are more likely to be picked up by express elevators.
Freight: This type of passenger has a request percentage of 15%. Freight passengers have large
items that need to be transported and are more likely to be picked up by freight elevators.
Glass: This type of passenger has a request percentage of 5%. Glass passengers have fragile items
that need to be transported and are more likely to be picked up by glass elevators.
There are 4 types of elevators in the system:
StandardElevator: This is the most common type of elevator and has a request percentage of 70%.
Standard elevators have a maximum capacity of 10 passengers.
ExpressElevator: This type of elevator has a request percentage of 20%. Express elevators have a
maximum capacity of 8 passengers and are faster than standard elevators.
FreightElevator: This type of elevator has a request percentage of 5%. Freight elevators have a
maximum capacity of 5 passengers and are designed to transport heavy items.
GlassElevator: This type of elevator has a request percentage of 5%. Glass elevators have a
maximum capacity of 6 passengers and are designed to transport fragile item
The project should include classes similar to this:
public class Simulation {
SimulatorSettings settings = new SimulatorSettings("settings.txt");
public void InitSimulation() throws FileNotFoundException{
///// Read all parameters from the file and store in the clas
File file = new File("settings.txt");
Scanner scanner = new Scanner(file);
//FileReader freader = new FileReader(file);
while(scanner.hasNextLine()){
String line = scanner.nextLine();
if(line.startsWith("floor="))
{
line= line.replace("floor=", "");
// Convert the value to the wanted type
System.out.println(line);
}
}
settings.setNofloors(55);
Passenger pass1 = new StandardPassenger();
pass1.requestElevator(settings);
ArrayList<Passenger> passengers = null;
for(int i = 0; i < 100; i++){
//Use the percentage from the file
passengers.add(new StandardPassenger());
}
}
}
public class SimulatorSettings {
SimulatorSettings(String fileName){
}
private int nofloors;
public int getNofloors() {
return nofloors;
}
public void setNofloors(int nofloors) {
this.nofloors = nofloors;
}
}
public abstract class Passenger {
public static int passangerCounter = 0;
private String passengerID;
protected int startFloor;
protected int endFloor;
Passenger(){
this.passengerID = ""+passangerCounter;
passangerCounter++;
}
public abstract boolean requestElevator(SimulatorSettings settings);
}
public class StandardPassenger extends Passenger{
private String type;
public StandardPassenger() {
}
public boolean requestElevator(SimulatorSettings settings){
Random rand = new Random();
this.startFloor = rand.nextInt()% settings.getNofloors();
this.endFloor = rand.nextInt() % settings.getNofloors();
while(this.startFloor == this.endFloor){
this.endFloor = rand.nextInt() % settings.getNofloors();
}
return true;
}
}
public class VIPPassenger extends Passenger{
}
public class FreightPassenger extends Passenger{
}
public class GlassPassenger extends Passenger{
}
public abstract class Elevator{
}
public class StandardElevator extends Elevator{
}
public class ExpressElevator extends Elevator{
}
public class FreightElevator extends Elevator{
}
public class GlassElevator extends Elevator{
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

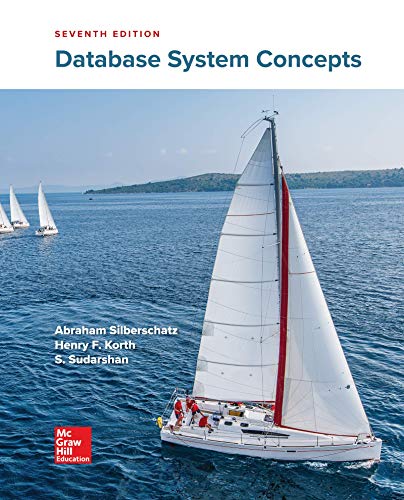
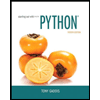
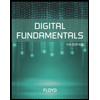
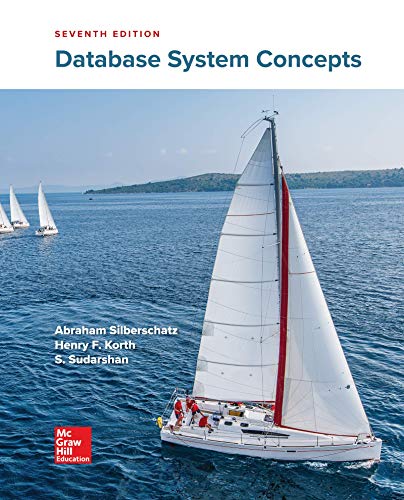
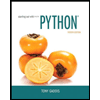
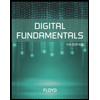
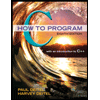
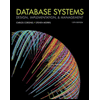
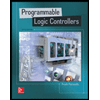