Using Python you may not use any list functions other than len(), pop(), extend() and append(). List slices are allowed used the [:] operated if wanted. You may not import any additional modules other than hw4data mentioned. Filename: py Starter code for data: py Download hw4data.py Download hw4data.py You must provide a function named decode()that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this: def decode(data : list) -> list: Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run. For example, using Python lists, the initial list: [‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’] would be encoded as: ['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2] So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the smaller your overall data size, the less opportunity for any compression algorithm to save much space) but imagine if we had a run of 30 or 40 P’s and 10 Y’s. The space savings begin to add up. Our RLE scheme for this assignment uses the format shown in the example above. Data is contained in a list such that the elements follow the pattern: Notice the data contained in our list is heterogeneous. In other words, it's NOT all the same type. strings and integers are interleaved in the list. Be sure to consider this as you're designing your solution. Do this: Write a program that decompresses the data included in the file starter file py. IMPORTANT: Importhw4data.py, do NOT copy/paste the data! If you copy-paste and introduce a subtle data error due to "fat fingering" or some other mistake, your RLE program may not work correctly and you will lose points. The data is a Python file - import it as any other "outside asset" file (e.g. external functions) and proceed from there. There are six data values of increasing size and complexity: DATA0, DATA1, …DATA5. Your program must provide a function named decode(list) that takes a list of RLE-encoded values and returns a list containing the decoded values with the “runs” expanded. We will call your decode()function in our test suite, so name the function exactly as specified, and make sure it returns a Python list. hw4data: DATA0 = ['P', 5, 'Y', 3, "P", 1, "G", 2] DATA1 = ['=', 98, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 9, ' ', 2, '=', 2, ' ', 2, '=', 16, ' ', 5, '=', 4, ' ', 6, '=', 3, ' ', 9, '=', 3, ' ', 6, '=', 4, ' ', 6, '=', 6, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 9, ' ', 2, '=', 2, ' ', 2, '=', 15, ' ', 2, '=', 3, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 9, ' ', 3, '=', 2, ' ', 3, '=', 2, ' ', 3, '=', 2, ' ', 3, '=', 4, ' ', 3, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 9, ' ', 2, '=', 2, ' ', 2, '=', 14, ' ', 2, '=', 8, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 9, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 3, ' ', 3, '=', 3, ' ', 2, '=', 2, ' ', 2, '=', 3, ' ', 3, '=', 8, ' ', 2, '=', 9, ' ', 2, '=', 7, ' ', 2, '=', 1, ' ', 4, '=', 4, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 8, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 5, '=', 7, ' ', 2, '=', 11, ' ', 2, '=', 5, ' ', 3, '=', 3, ' ', 2, '=', 3, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 5, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 7, ' ', 2, '=', 13, ' ', 2, '=', 3, ' ', 2, '=', 5, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 5, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 7, ' ', 2, '=', 8, ' ', 2, '=', 4, ' ', 2, '=', 9, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 8, ' ', 2, '=', 3, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 3, ' ', 3, '=', 2, ' ', 3, '=', 2, ' ', 3, '=', 2, ' ', 3, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 3, ' ', 3, '=', 3, ' ', 2, '=', 2, ' ', 2, '=', 3, ' ', 3, '=', 10, ' ', 5, '=', 4, ' ', 6, '=', 5, ' ', 5, '=', 5, ' ', 6, '=', 4, ' ', 6, '=', 4, ' ', 6, '=', 2, '\n', 1, '=', 98]
Using Python you may not use any list functions other than len(), pop(), extend() and append().
List slices are allowed used the [:] operated if wanted.
You may not import any additional modules other than hw4data mentioned.
- Filename: py
- Starter code for data: py Download hw4data.py
- Download hw4data.py You must provide a function named decode()that takes a list of RLE-encoded values as its single parameter, and returns a list containing the decoded values with the “runs” expanded. If we were to use Python annotations, the function signature would look similar to this:
def decode(data : list) -> list:
Run-length encoding (RLE) is a simple, “lossless” compression scheme in which “runs” of data. The same value in consecutive data elements are stored as a single occurrence of the data value, and a count of occurrences for the run.
For example, using Python lists, the initial list:
[‘P’,‘P’,‘P’,‘P’,‘P’,‘Y’,‘Y’,‘Y’,‘P’,‘G’,‘G’]
would be encoded as:
['P', 5, 'Y', 3, ‘P’, 1, ‘G’, 2]
So, instead of using 11 “units” of space (if we consider the space for a character/int 1 unit), we only use 8 “units”. In this small example, we don’t achieve much of a savings (and indeed the smaller your overall data size, the less opportunity for any compression
Our RLE scheme for this assignment uses the format shown in the example above. Data is contained in a list such that the elements follow the pattern:
Notice the data contained in our list is heterogeneous. In other words, it's NOT all the same type. strings and integers are interleaved in the list. Be sure to consider this as you're designing your solution.
Do this:
- Write a program that decompresses the data included in the file starter file py.
IMPORTANT: Importhw4data.py, do NOT copy/paste the data! If you copy-paste and introduce a subtle data error due to "fat fingering" or some other mistake, your RLE program may not work correctly and you will lose points. The data is a Python file - import it as any other "outside asset" file (e.g. external functions) and proceed from there. - There are six data values of increasing size and complexity: DATA0, DATA1, …DATA5. Your program must provide a function named decode(list) that takes a list of RLE-encoded values and returns a list containing the decoded values with the “runs” expanded. We will call your decode()function in our test suite, so name the function exactly as specified, and make sure it returns a Python list.
hw4data:
DATA0 = ['P', 5, 'Y', 3, "P", 1, "G", 2]
DATA1 = ['=', 98, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 9, ' ', 2, '=',
2, ' ',
2, '=', 16, ' ', 5, '=', 4, ' ', 6, '=', 3, ' ', 9, '=', 3, ' ', 6, '=', 4,
' ', 6, '=', 6, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=',
9, ' ', 2, '=', 2, ' ', 2, '=', 15, ' ', 2, '=', 3, ' ', 2, '=', 2, ' ', 2,
'=', 4, ' ', 2, '=', 2, ' ', 2, '=', 9, ' ', 3, '=', 2, ' ', 3, '=', 2, ' ',
3, '=', 2, ' ', 3, '=', 4, ' ', 3, '=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4,
' ', 2, '=', 9, ' ', 2, '=', 2, ' ', 2, '=', 14, ' ', 2, '=', 8, ' ', 2,
'=', 4, ' ', 2, '=', 2, ' ', 2, '=', 9, ' ', 2, '=', 4, ' ', 2, '=', 2,
' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 2,
'=', 4, ' ', 2, '=', 3, ' ', 3, '=', 3, ' ', 2, '=', 2, ' ', 2, '=', 3,
' ', 3, '=', 8, ' ', 2, '=', 9, ' ', 2, '=', 7, ' ', 2, '=', 1, ' ',
4, '=', 4, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2,
'=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1, ' ', 8, '=', 2, ' ', 2,
'=', 1, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 5,
'=', 7, ' ', 2, '=', 11, ' ', 2, '=', 5, ' ', 3, '=', 3, ' ', 2, '=', 3,
' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2,
'=', 4, '\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 5, '=', 2,
' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 7, ' ', 2,
'=', 13, ' ', 2, '=', 3, ' ', 2, '=', 5, ' ', 2, '=', 2, ' ', 2, '=',
4, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4,
'\n', 1, '=', 1, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 5, ' ', 2,
'=', 2, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 7, ' ', 2, '=', 8,
' ', 2, '=', 4, ' ', 2, '=', 9, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2,
'=', 2, ' ', 2, '=', 4, ' ', 2, '=', 5, ' ', 2, '=', 4, '\n', 1, '=',
1, ' ', 2, '=', 4, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 2,
' ', 2, '=', 2, ' ', 2, '=', 2, ' ', 2, '=', 1, ' ', 2, '=', 8,
' ', 2, '=', 3, ' ', 2, '=', 2, ' ', 2, '=', 4, ' ', 2, '=', 2,
' ', 2, '=', 4, ' ', 2, '=', 3, ' ', 3, '=', 2, ' ', 3, '=', 2,
' ', 3, '=', 2, ' ', 3, '=', 5, ' ', 2, '=', 4, '\n', 1, '=', 1,
' ', 2, '=', 4, ' ', 2, '=', 3, ' ', 3, '=', 3, ' ', 2, '=', 2, ' ', 2, '=',
3, ' ', 3, '=', 10, ' ', 5, '=', 4, ' ', 6, '=', 5, ' ', 5, '=', 5, ' ', 6,
'=', 4, ' ', 6, '=', 4, ' ', 6, '=', 2, '\n', 1, '=', 98]

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

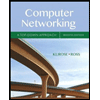
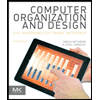
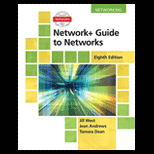
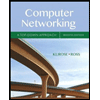
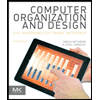
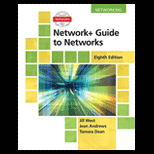
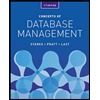
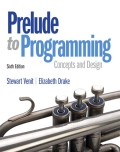
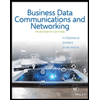