Using Java program the following: This program should prompt a user for an integer n and then add first n positive integers, (i.e. 1+2+3+...+n) The program should use a while loop to do that and print out each intermediate step. The last formula (given to you) acts as a check. e.g. Enter the number of integers to add: 10 1 = 1 1+2 = 3 1+2+3 = 6 1+2+3+4 = 10 1+2+3+4+5 = 15 1+2+3+4+5+6 = 21 1+2+3+4+5+6+7 = 28 1+2+3+4+5+6+7+8 = 36 1+2+3+4+5+6+7+8+9 = 45 1+2+3+4+5+6+7+8+9+10 = 55 check: last sum=55 import java.util.Scanner; public class AddFirstNIntegers{ public static void main(String[] args){ //1. Create a Scanner object named sc; //2. Prompt the user to enter the number of positive integers to add //3. Read n, the number of integers to add: //4. Initialize the counter for the loop (1, 2, or 0 would work, just need to keep it in mind what works better down the road) //5. Initialize the variable sum to 1. (Will represent your current sum. We start with 1=1) //6. Initialize the String variable s to be "1" //(Will represent the string for the current sum. e.g. "1+2+3+4+5".) //7. Use s and sum to print out (s+" = "+sum) (which should end up being 1 = 1 -- our initial statement) System.out.println(s+" = "+sum); //8. create a while loop that in each iteration will add the next //integer to the previous sum, uppend "+" and next integer to String s, //and print them out as shown above. while (...){ } //Check: your last number should be (n+1)*n/2: System.out.println(" check: "+(n+1)*n/2); } }
Using Java program the following: This program should prompt a user for an integer n and then add first n positive integers, (i.e. 1+2+3+...+n) The program should use a while loop to do that and print out each intermediate step. The last formula (given to you) acts as a check. e.g. Enter the number of integers to add: 10 1 = 1 1+2 = 3 1+2+3 = 6 1+2+3+4 = 10 1+2+3+4+5 = 15 1+2+3+4+5+6 = 21 1+2+3+4+5+6+7 = 28 1+2+3+4+5+6+7+8 = 36 1+2+3+4+5+6+7+8+9 = 45 1+2+3+4+5+6+7+8+9+10 = 55 check: last sum=55 import java.util.Scanner; public class AddFirstNIntegers{ public static void main(String[] args){ //1. Create a Scanner object named sc; //2. Prompt the user to enter the number of positive integers to add //3. Read n, the number of integers to add: //4. Initialize the counter for the loop (1, 2, or 0 would work, just need to keep it in mind what works better down the road) //5. Initialize the variable sum to 1. (Will represent your current sum. We start with 1=1) //6. Initialize the String variable s to be "1" //(Will represent the string for the current sum. e.g. "1+2+3+4+5".) //7. Use s and sum to print out (s+" = "+sum) (which should end up being 1 = 1 -- our initial statement) System.out.println(s+" = "+sum); //8. create a while loop that in each iteration will add the next //integer to the previous sum, uppend "+" and next integer to String s, //and print them out as shown above. while (...){ } //Check: your last number should be (n+1)*n/2: System.out.println(" check: "+(n+1)*n/2); } }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Using Java program the following:
This program should prompt a user for an integer n
and then add first n positive integers,
(i.e. 1+2+3+...+n)
The program should use a while loop to do that
and print out each intermediate step.
The last formula (given to you) acts as a check.
e.g.
Enter the number of integers to add: 10
1 = 1
1+2 = 3
1+2+3 = 6
1+2+3+4 = 10
1+2+3+4+5 = 15
1+2+3+4+5+6 = 21
1+2+3+4+5+6+7 = 28
1+2+3+4+5+6+7+8 = 36
1+2+3+4+5+6+7+8+9 = 45
1+2+3+4+5+6+7+8+9+10 = 55
check: last sum=55
import java.util.Scanner;
public class AddFirstNIntegers{
public static void main(String[] args){
//1. Create a Scanner object named sc;
//2. Prompt the user to enter the number of positive integers to add
//3. Read n, the number of integers to add:
//4. Initialize the counter for the loop (1, 2, or 0 would work, just need to keep it in mind what works better down the road)
//5. Initialize the variable sum to 1. (Will represent your current sum. We start with 1=1)
//6. Initialize the String variable s to be "1"
//(Will represent the string for the current sum. e.g. "1+2+3+4+5".)
//7. Use s and sum to print out (s+" = "+sum) (which should end up being 1 = 1 -- our initial statement)
System.out.println(s+" = "+sum);
//8. create a while loop that in each iteration will add the next
//integer to the previous sum, uppend "+" and next integer to String s,
//and print them out as shown above.
while (...){
}
//Check: your last number should be (n+1)*n/2:
System.out.println(" check: "+(n+1)*n/2);
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
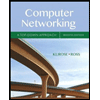
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
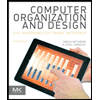
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
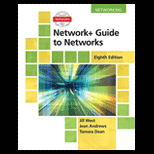
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
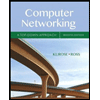
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
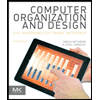
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
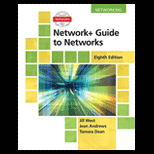
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
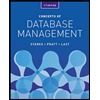
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
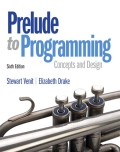
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
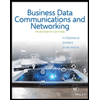
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY