Using C++, write a payroll system program using the concepts of inheritance and polymorphism. Write the program following these steps: 1. Define the following constants in a header file: FACULTY_MONTHLY_SALARY = 5000.00 STAFF_MONTHLY_HOURS_WORKED = 160
Using C++, write a payroll system program using the concepts of inheritance and polymorphism.
Write the program following these steps:
1. Define the following constants in a header file:
- FACULTY_MONTHLY_SALARY = 5000.00
- STAFF_MONTHLY_HOURS_WORKED = 160
2. Implement an abstract class Employee with the following requirements:
Attributes:
- last name (String)
- first name (String)
- ID number (String)
- Sex - M or F
- Birthdate (Date)
- Default argument constructor and argument constructors.
- Public methods
- putData that displays the following information:
ID Employee number :_________
Employee name: __________
Birth date: _______
- get and set methods
- pure virtual method monthlyEarning that returns the monthly earning.
3. Implement a class called Staff extending from the class Employee with the following requirements:
Attributes:
-Hourly rate
-Default argument and argument contructors
-Public methods
-get and set
-The method monthlyEarning returns monthly salary (hourly rate times 160)
-putData that displays the following information:
ID Employee number :_________
Employee name: __________
Birth date: _______
Full Time
Monthly Salary: _________
4.Implement a class Education with the following requirements:
Attributes:
- Degree (MS or PhD )
- Major (Engineering, Chemistry, English, etc... )
- Research (number of researches)
Default argument and argument constructors.
Public methods:
-get and set
5. Implement a class Faculty extending from the class Employee with the following requirements:
Attributes:
Level:
"AS": assistant professor
"AO": associate professor
"FU": professor
-Education object
- Default argument and argument constructor
- Public methods
-get and set
-The method monthlyEarning returns monthly salary based on the faculty's level.
AS - faculty monthly salary
AO - 1.2 times faculty monthly salary
FU - 1.4 times faculty monthly salary
-putData that displays the following information:
ID Employee number :_________
Employee name: __________
Birth date: _______
XXXXX Professor where XXXXX can be Assistant, Associate or Full
Monthly Salary: _________
6. Implement a class called PartTime extending from the class Staff with the following requirements:
Attributes:
- Hours worked per week
- Default argument and argument constructors
- Public methods
- set and get
- The method monthlyEarning returns monthly salary which hourly rate multiplied hours worked per week multiplied four.
- putData that displays the following information:
ID Employee number :_________
Employee name: __________
Birth date: _______
Hours works per month: ______
Monthly Salary: _________
7.Implement a test driver program that creates a one-dimensional array of class Employee to store the objects Staff, Faculty and PartTime.
Using polymorphism, display the following outputs:
8. Employee information using the method putData.
- All employees
- Staff
- Faculty
- Part-time
- Total monthly salary for all the part-time staff.
- Total monthly salary for all employees.
Test Data to output:
Staff
Last name: Costello
First name: Maria
ID: 1421
Sex: F
Birth date: 1/13/55
Hourly rate: $50.00
Last name: Buenaventura
First Name: Emilio
ID: 125
Sex: M
Birth date: 10/31/68
Hourly rate: $35.00
Last name: Rodriguez
First name: Juan
ID: 891
Sex: M
Birth date: 12/29/66
Hourly rate: $40.00
Faculty
Last name: Poisson
First name: James
ID: 781
Sex: M
Birth date: 7/27/82
Level: Full
Degree: Ph.D
Major: Engineering
Reseach: 3
Last name: Mandarin
First name: Matthew
ID: 7432
Sex: M
Birth date: 12/15/76
Level: Associate
Degree: Ph.D
Major: English
Reseach: 1
Last name: Kristoff
First name: Conrad
ID: 481
Sex: M
Birth date: 04/02/1975
Level: Assistant
Degree: MS
Major: Physical Education
Research: 0
Part-time
Last name: Augustin
First name: Fernando
ID: 551
Sex: M
Birth date: 2/20/1978
Hourly rate: $35.00
Hours worked per week: 30
Last name: Casimiro
First name: Henry
ID: 467
Sex: M
Birth date: 01/15/68
Hourly rate: $30.00
Hours worked per week:15
Last name: Yassar
First name: John
ID: 169
Sex: M
Birth date: 09/21/91
Hourly rate: $18.00
Hours worked per week: 35

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

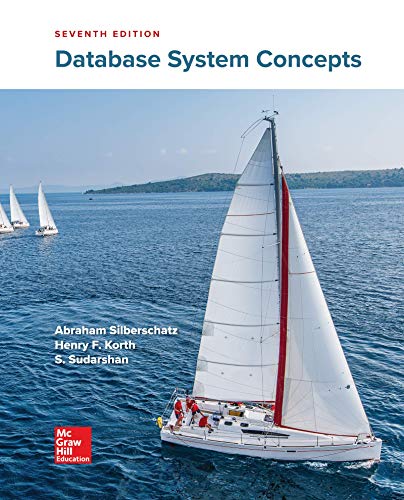
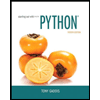
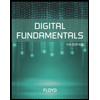
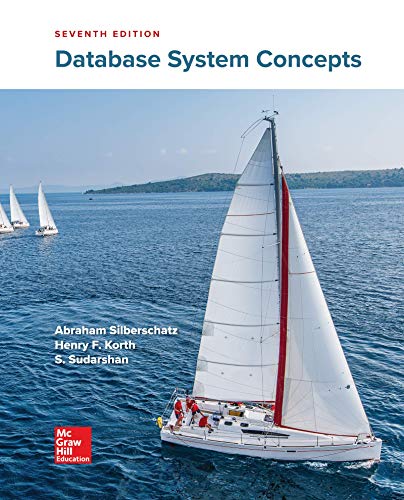
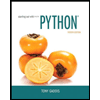
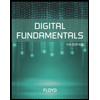
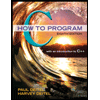
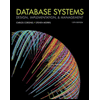
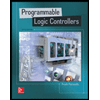