Using C programming language: A Transposition Cipher A very simple transposition cipher encrypt(S, N) can be described by the following rules: If the length of S is 1 or 2, then encrypt(S, N) is S. If S is a string of N characters s1 s2 s3... sN and k = N/2, then encrypt(S)= encrypt(sk sk−1... s2 s1 ,K)+ encrypt(sN sN−1... sk+1 ,N - K) where + indicates string concatenation. For example, encrypt("Ok", 2) = "Ok" and encrypt("12345678", 8) = "34127856". Write a program to implement this cipher. The input is a file that is guaranteed to have less then 2048 characters. Code structure might look like this: #define MAX_SIZE 2048 char text_buffer[MAX_SIZE]; int main() { // read file into text_buffer encrypt(text_buffer, n); // print out text_buffer return 0; }
Using C
A Transposition Cipher
A very simple transposition cipher encrypt(S, N) can be described by the following rules:
If the length of S is 1 or 2, then encrypt(S, N) is S.
If S is a string of N characters s1 s2 s3... sN and k = N/2, then
encrypt(S)= encrypt(sk sk−1... s2 s1 ,K)+ encrypt(sN sN−1... sk+1 ,N - K)
where + indicates string concatenation.
For example, encrypt("Ok", 2) = "Ok" and encrypt("12345678", 8) = "34127856".
Write a program to implement this cipher.
The input is a file that is guaranteed to have less then 2048 characters.
Code structure might look like this:
#define MAX_SIZE 2048
char text_buffer[MAX_SIZE];
int main()
{
// read file into text_buffer
encrypt(text_buffer, n);
// print out text_buffer
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

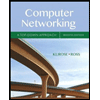
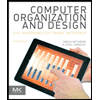
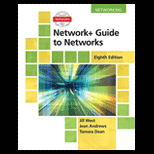
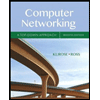
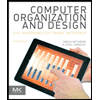
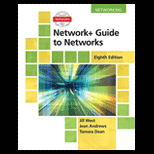
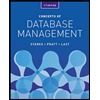
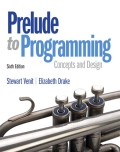
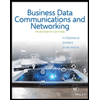