use the method setData(String,String,doule,int) to set the employee’s details; and the method getDetails() that will return a descriptive statement about the employee. import java.util.*; class Department { private int groupScore; private double groupAverage; private static int overallScore; private static double overallAverage; public Department(int r1,int r2,int r3,int r4,int r5) { Department.overallScore=Department.overallScore+r1+r2+r3+r4+r5; Department.overallAverage=Department.overallScore/10.0; this.groupScore=r1+r2+r3+r4+r5; this.groupAverage=this.groupScore/5.0; } public double getGroupAvergae() { return this.groupAverage; } public void displayAverage() { System.out.println("Overall Average:"+Department.overallAverage); } } public class Main { public static void main(String args[]) { Department d1=new Department(1,2,3,4,5); d1.displayAverage(); Department d2=new Department(5,4,4,4,1); d2.displayAverage(); System.out.println("D1 Group average:"+d1.getGroupAvergae()); System.out.println("D2 Group average:"+d2.getGroupAvergae()); } }
- use the method setData(String,String,doule,int) to set the employee’s details; and the method getDetails() that will return a descriptive statement about the employee.
import java.util.*;
class Department
{
private int groupScore;
private double groupAverage;
private static int overallScore;
private static double overallAverage;
public Department(int r1,int r2,int r3,int r4,int r5)
{
Department.overallScore=Department.overallScore+r1+r2+r3+r4+r5;
Department.overallAverage=Department.overallScore/10.0;
this.groupScore=r1+r2+r3+r4+r5;
this.groupAverage=this.groupScore/5.0;
}
public double getGroupAvergae()
{
return this.groupAverage;
}
public void displayAverage()
{
System.out.println("Overall Average:"+Department.overallAverage);
}
}
public class Main
{
public static void main(String args[])
{
Department d1=new Department(1,2,3,4,5);
d1.displayAverage();
Department d2=new Department(5,4,4,4,1);
d2.displayAverage();
System.out.println("D1 Group average:"+d1.getGroupAvergae());
System.out.println("D2 Group average:"+d2.getGroupAvergae());
}
}

Step by step
Solved in 3 steps

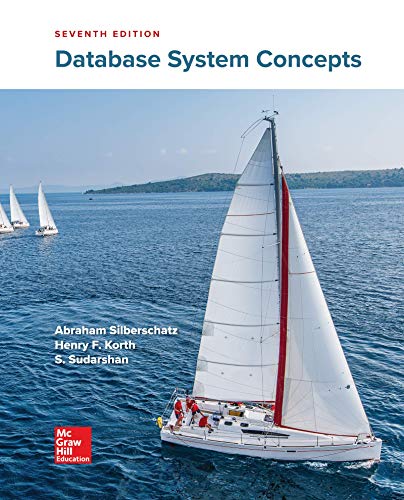
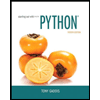
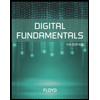
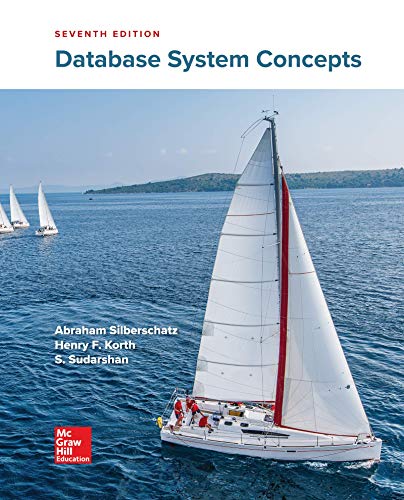
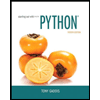
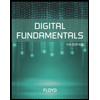
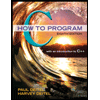
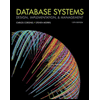
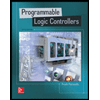